Overflow Encountered in numpy.exp() Function in Python
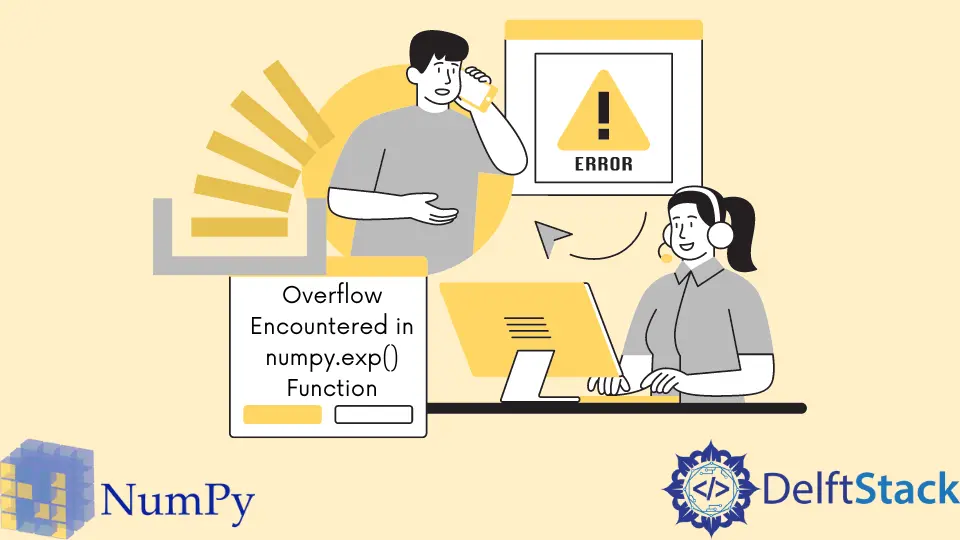
The NumPy
is a Python package that is rich with utilities for playing around with large multi-dimensional matrices and arrays and performing both complex and straightforward mathematical operations over them.
These utilities are dynamic to the inputs and highly optimized and fast. The NumPy
package has a function exp()
that calculates the exponential of all the elements of an input numpy array.
In other words, it computes ex, x
is every number of the input numpy array, and e
is the Euler’s number that is approximately equal to 2.71828
.
Since this calculation can result in a huge number, some data types fail to handle such big values, and hence, this function will return inf
and an error instead of a valid floating value.
For example, this function will return 8.21840746e+307
for numpy.exp(709)
but runtimeWarning: overflow encountered in exp inf
for numpy.exp(710)
.
In this article, we will learn how to fix this issue.
Fix for Overflow in numpy.exp()
Function in Python NumPy
We have to store values in a data type capable of holding such large values to fix this issue.
For example, np.float128
can hold way bigger numbers than float64
and float32
. All we have to do is just typecast each value of an array to a bigger data type and store it in a numpy array.
The following Python code depicts this.
import numpy as np
a = np.array([1223, 2563, 3266, 709, 710], dtype=np.float128)
print(np.exp(a))
Output:
[1.38723925e+0531 1.24956001e+1113 2.54552810e+1418 8.21840746e+0307
2.23399477e+0308]
Although the above Python code runs seamlessly without any issues, still, we are prone to the same error.
The reason behind it is pretty simple; even np.float128
has a threshold value for numbers it can hold. Every data type has an upper-cap, and if that upper-cap is crossed, things start getting buggy, and programs start running into overflow errors.
To understand the point mentioned above, refer to the following Python code. Even though np.float128
solved our problem in the last Python code snippet, it would not work for even bigger values.
import numpy as np
a = np.array([1223324, 25636563, 32342266, 235350239, 27516346320], dtype=np.float128)
print(np.exp(a))
Output:
<string>:4: RuntimeWarning: overflow encountered in exp
[inf inf inf inf inf]
The exp()
function returns an infinity for every value in the numpy array.
To learn about the
numpy.exp()
function, refer to the officialNumPy
documentation here.