How to Reverse Array in NumPy
- Reverse a NumPy Array With the Basic Slicing Method in Python
-
Reverse a NumPy Array With the
numpy.flipud()
Function in Python -
Reverse a NumPy Array With the
numpy.flip()
Function in Python
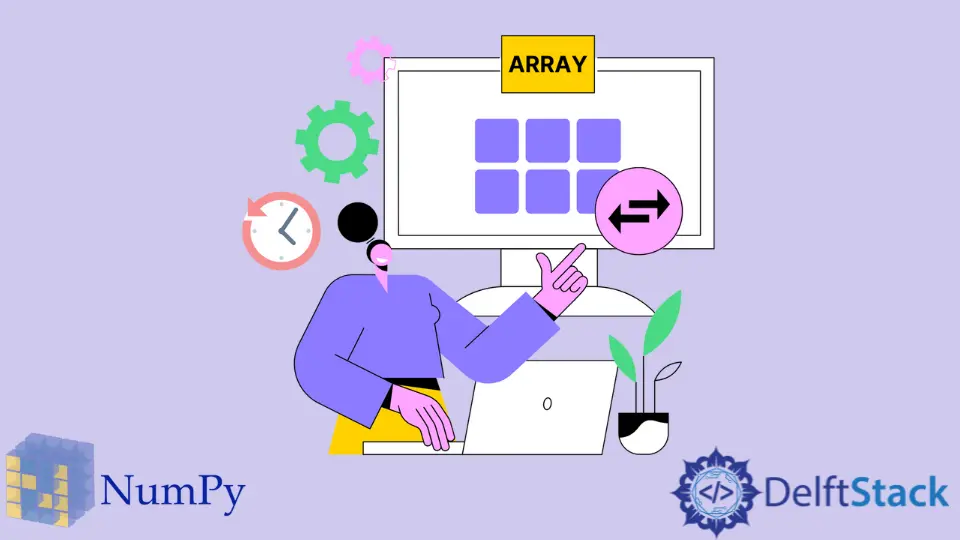
This tutorial will introduce the methods to reverse a NumPy array in Python.
Reverse a NumPy Array With the Basic Slicing Method in Python
We can use the basic slicing method to reverse a NumPy array. We can use the [::-1]
as the index of the array to reverse it. This method does not actually reverse the original array. Instead, it creates a custom view of the array that points to the original array but in a reverse sequence. The following code example demonstrates how we can reverse a NumPy array with the basic slicing method in Python.
import numpy as np
array = np.array([1, 2, 3, 4, 5])
reverse = array[::-1]
print(reverse)
Output:
[5 4 3 2 1]
In the above code, we reversed elements of the NumPy array array
with the array[::-1]
index in Python. We first created and initialized the array array
and saved the reverse view of the array
inside the reverse
array with the basic slicing method. In the end, we display the values inside the reverse
array with the print()
function in Python.
Reverse a NumPy Array With the numpy.flipud()
Function in Python
Another function that can be used to reverse an array is the numpy.flipud()
function. The numpy.flipud()
function flips the elements of the array upside down. The numpy.flipud()
function takes the array as an argument and returns the reverse of that array. See the following code example.
import numpy as np
array = np.array([1, 2, 3, 4, 5])
reverse = np.flipud(array)
print(reverse)
Output:
[5 4 3 2 1]
In the above code, we reversed the NumPy array array
elements with the numpy.flipud()
function in Python. We first created and initialized our original array array
with the numpy.array()
function. We then reversed the array
with the numpy.flipud()
function and saved the result inside the reverse
array.
Reverse a NumPy Array With the numpy.flip()
Function in Python
We can also use the numpy.flip()
function to reverse a NumPy array in Python. The numpy.flip()
function reverses the order of the elements inside the array along a specified axis
in Python. By default, the value of the axis
is set to None
. We would not need to specify the axis for a 1-dimensional NumPy array. See the following code example.
import numpy as np
array = np.array([1, 2, 3, 4, 5])
reverse = np.flip(array)
print(reverse)
Output:
[5 4 3 2 1]
In the above code, we reversed the NumPy array array
elements with the numpy.flip()
function in Python. We first created and initialized our original array array
with the numpy.array()
function. We then reversed the sequence of elements inside the array
with the numpy.flip()
function and saved the result inside the reverse
array.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn