NumPy dot vs matmul in Python
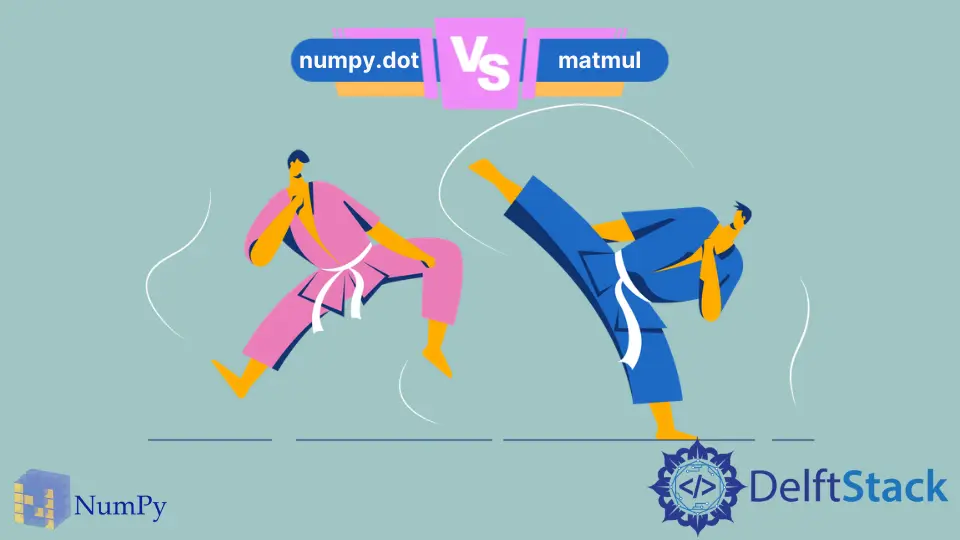
In Python, arrays are treated as vectors. 2-D arrays are also called matrices. We have functions available to carry out multiplication between them in Python. The two methods used are the numpy.dot()
function and the @
operator (the array’s __matmul__
method). Now it may seem that they both perform the same function of multiplication. However, there is some difference between both of them, which is explained in this tutorial.
The numpy.dot()
function is used for performing matrix multiplication in Python. It also checks the condition for matrix multiplication, that is, the number of columns of the first matrix must be equal to the number of the rows of the second. It works with multi-dimensional arrays also. We can also specify an alternate array as a parameter to store the result. The @
operator for multiplication invokes the matmul()
function of an array that is used to perform the same multiplication. For example,
import numpy as np
a = np.array([[1, 2], [2, 3]])
b = np.array(([8, 4], [4, 7]))
print(np.dot(a, b))
print(a @ b)
Output:
[[16 18]
[28 29]]
[[16 18]
[28 29]]
However, when we deal with multi-dimensional arrays (N-D arrays with N>2) the result is slightly different. You can see the difference below.
a = np.random.rand(2, 3, 3)
b = np.random.rand(2, 3, 3)
c = a @ b
d = np.dot(a, b)
print(c, c.shape)
print(d, d.shape)
Output:
[[[0.63629871 0.55054463 0.22289276]
[1.27578425 1.13950519 0.55370078]
[1.37809353 1.32313811 0.75460862]]
[[1.63546361 1.54607801 0.67134528]
[1.05906619 1.07509384 0.42526795]
[1.38932102 1.32829749 0.47240808]]] (2, 3, 3)
[[[[0.63629871 0.55054463 0.22289276]
[0.7938068 0.85668481 0.26504028]]
[[1.27578425 1.13950519 0.55370078]
[1.55589497 1.45794424 0.5335743 ]]
[[1.37809353 1.32313811 0.75460862]
[1.60564885 1.39494713 0.59370927]]]
[[[1.48529826 1.55580834 0.96142976]
[1.63546361 1.54607801 0.67134528]]
[[0.94601586 0.97181894 0.56701004]
[1.05906619 1.07509384 0.42526795]]
[[1.13268609 1.00262696 0.47226983]
[1.38932102 1.32829749 0.47240808]]]] (2, 3, 2, 3)
The matmul()
function broadcasts the array like a stack of matrices as elements residing in the last two indexes, respectively. The numpy.dot()
function, on the other hand, performs multiplication as the sum of products over the last axis of the first array and the second-to-last of the second.
Another difference between the matmul()
and the numpy.dot
function is that the matmul()
function cannot perform multiplication of array with scalar values.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn