How to Count Zeros in NumPy Array
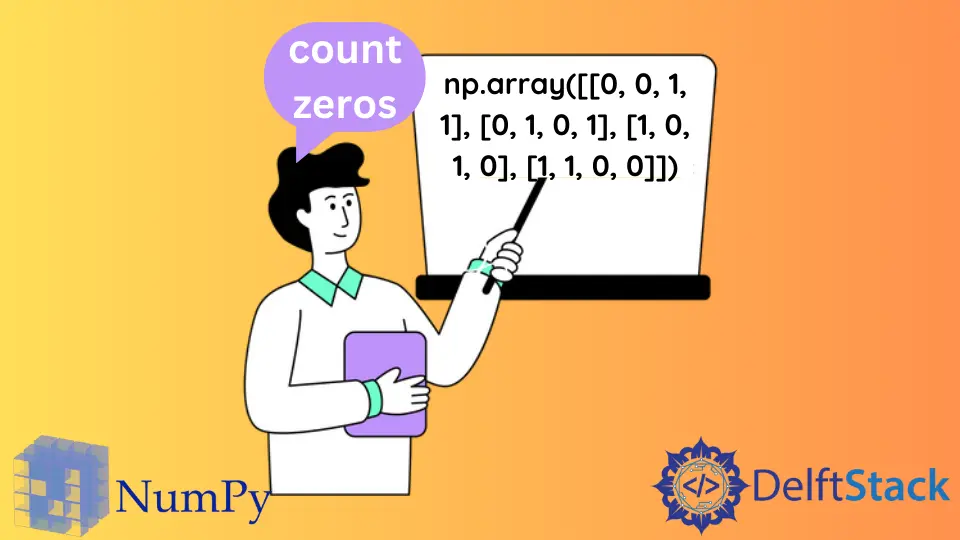
Sometimes, we have to count elements inside an array. When counting, we sometimes associate a condition and count the elements satisfying that condition. It can be a greater than condition, a less than condition, an equals condition, etc.
In this article, we will learn how to count zeroes in a NumPy array efficiently.
NumPy comes with all sorts of methods that we can apply to multidimensional NumPy arrays and matrices. It also has some functions that we can use to count zeroes.
This article will discuss two such methods, count_nonzero()
and where()
.
Count Zeroes in a NumPy Array Using count_nonzero()
As the name suggests, this method counts the non-zero elements. We will use this function to count zeroes.
count_nonzero()
returns an integer value or an array of integer values.
The syntax of count_nonzero()
is below.
count_nonzero(a, axis, keepdims)
It has the following parameters.
a
- The array in which the function will count zeroesaxis
- It is an optional parameter, and it refers to the axis or tuple of axes along which the non-zero elements will be counted. The default value of this parameter isNone
, which means that the counting will be done on a flattened array.keepdims
- This is an optional boolean parameter. By default, it isFalse
. If set toTrue
, the axes counted are left in the result as dimensions with size 1.
Now, let’s count zeroes using this method. Refer to the following code snippet for the first way.
import numpy as np
myArray = np.array([1, 2, 0, 3, 4, 0, 5, 6, 0])
myMatrix = np.array([[0, 0, 1, 1], [0, 1, 0, 1], [1, 0, 1, 0], [1, 1, 0, 0]])
print(myArray)
print(myMatrix)
print(f"Number of Non-Zeroes in Array --> {np.count_nonzero(myArray)}")
print(f"Number of Non-Zeroes in Matrix --> {np.count_nonzero(myMatrix)}")
print(f"Number of Zeroes in Array --> {myArray.size - np.count_nonzero(myArray)}")
print(f"Number of Zeroes in Matrix --> {myMatrix.size - np.count_nonzero(myMatrix)}")
Output:
[1 2 0 3 4 0 5 6 0]
[[0 0 1 1]
[0 1 0 1]
[1 0 1 0]
[1 1 0 0]]
Number of Non-Zeroes in Array --> 6
Number of Non-Zeroes in Matrix --> 8
Number of Zeroes in Array --> 3
Number of Zeroes in Matrix --> 8
In the above code snippet, all we have done is counting the number of non-zero elements and then subtracting the number of non-zero elements from the total size of the array or matrix.
This solution might not be the best use of this function, but the following is.
import numpy as np
myArray = np.array([1, 2, 0, 3, 4, 0, 5, 6, 0])
myMatrix = np.array([[0, 0, 1, 1], [0, 1, 0, 1], [1, 0, 1, 0], [1, 1, 0, 0]])
print(myArray)
print(myMatrix)
print(myArray == 0)
print(myMatrix == 0)
print(f"Number of Zeroes in Array --> {np.count_nonzero(myArray == 0)}")
print(f"Number of Zeroes in Matrix --> {np.count_nonzero(myMatrix == 0)}")
Output:
[1 2 0 3 4 0 5 6 0]
[[0 0 1 1]
[0 1 0 1]
[1 0 1 0]
[1 1 0 0]]
[False False True False False True False False True]
[[ True True False False]
[ True False True False]
[False True False True]
[False False True True]]
Number of Zeroes in Array --> 3
Number of Zeroes in Matrix --> 8
The core of this solution is the principle that anything in computer science that is False
can be represented as 0
, and True
can be represented as a non-zero value.
myArray == 0
statement returns an array in which all the elements that satisfy the property are True
and those which don’t are False
. And the condition itself checks whether an element is zero or not. So, all the zero elements will turn into True
and now, we have to count them. And for that, we use the count_nonzero()
method.
Here is the link to the official documentation of the function.
Count Zeroes in a NumPy Array Using where()
The where()
function filters the elements from an array based on a specified condition and returns the filtered array. It returns the indexes of the filtered elements. Using this function, we will construct an array that only has zeroes, and the length of this new array will give me the count of zeroes.
Now, let’s see the solution.
import numpy as np
myArray = np.array([1, 2, 0, 3, 4, 0, 5, 6, 0])
myMatrix = np.array([[0, 0, 1, 1], [0, 1, 0, 1], [1, 0, 1, 0], [1, 1, 0, 0]])
print(myArray)
print(myMatrix)
print(myArray[np.where(myArray == 0)])
print(myMatrix[np.where(myMatrix == 0)])
print(f"Number of Zeroes in Array --> {myArray[np.where(myArray == 0)].size}")
print(f"Number of Zeroes in Matrix --> {myMatrix[np.where(myMatrix == 0)].size}")
Output:
[1 2 0 3 4 0 5 6 0]
[[0 0 1 1]
[0 1 0 1]
[1 0 1 0]
[1 1 0 0]]
[0 0 0]
[0 0 0 0 0 0 0 0]
Number of Zeroes in Array --> 3
Number of Zeroes in Matrix --> 8
In the above code snippet, we filtered out all the elements that were zero. The where()
function returned the indexes of those elements. We further used those indexes to get the original elements. Obviously, they all will be zero. Lastly, we counted the number of those zeroes and printed the counts.