计算 NumPy 数组中的零
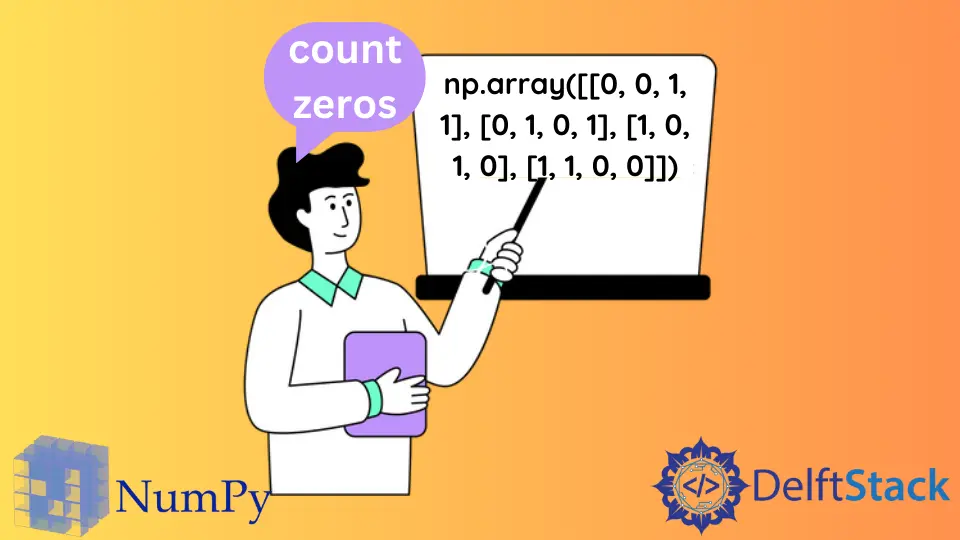
有时,我们必须对数组中的元素进行计数。在计数时,我们有时会关联一个条件并计算满足该条件的元素。它可以是大于条件,小于条件,等于条件等。
在本文中,我们将学习如何有效地计算 NumPy 数组中的零。
NumPy 附带了我们可以应用于多维 NumPy 数组和矩阵的各种方法。它还具有一些可用于计数零的功能。
本文将讨论两种这样的方法,count_nonzero()
和 where()
。
使用 count_nonzero()
计算 NumPy 数组中的零
顾名思义,此方法计算非零元素。我们将使用此函数来计数零。
count_nonzero()
返回一个整数值或一个整数值数组。
count_nonzero()
的语法如下。
count_nonzero(a, axis, keepdims)
它具有以下参数。
a
- 函数将在其中计数零的数组axis
- 这是一个可选参数,它是指将沿其计算非零元素的轴或轴元组。此参数的默认值为None
,这意味着将在展平的数组上进行计数。keepdims
- 这是一个可选的布尔参数。默认情况下,它是False
。如果设置为True
,则计数的轴将保留为尺寸为 1 的尺寸。
现在,让我们使用此方法计算零。有关第一种方法,请参考以下代码段。
import numpy as np
myArray = np.array([1, 2, 0, 3, 4, 0, 5, 6, 0])
myMatrix = np.array([[0, 0, 1, 1], [0, 1, 0, 1], [1, 0, 1, 0], [1, 1, 0, 0]])
print(myArray)
print(myMatrix)
print(f"Number of Non-Zeroes in Array --> {np.count_nonzero(myArray)}")
print(f"Number of Non-Zeroes in Matrix --> {np.count_nonzero(myMatrix)}")
print(f"Number of Zeroes in Array --> {myArray.size - np.count_nonzero(myArray)}")
print(f"Number of Zeroes in Matrix --> {myMatrix.size - np.count_nonzero(myMatrix)}")
输出:
[1 2 0 3 4 0 5 6 0]
[[0 0 1 1]
[0 1 0 1]
[1 0 1 0]
[1 1 0 0]]
Number of Non-Zeroes in Array --> 6
Number of Non-Zeroes in Matrix --> 8
Number of Zeroes in Array --> 3
Number of Zeroes in Matrix --> 8
在上面的代码片段中,我们要做的就是计算非零元素的数量,然后从数组或矩阵的总大小中减去非零元素的数量。
此解决方案可能不是此函数的最佳用途,但下面的方案是。
import numpy as np
myArray = np.array([1, 2, 0, 3, 4, 0, 5, 6, 0])
myMatrix = np.array([[0, 0, 1, 1], [0, 1, 0, 1], [1, 0, 1, 0], [1, 1, 0, 0]])
print(myArray)
print(myMatrix)
print(myArray == 0)
print(myMatrix == 0)
print(f"Number of Zeroes in Array --> {np.count_nonzero(myArray == 0)}")
print(f"Number of Zeroes in Matrix --> {np.count_nonzero(myMatrix == 0)}")
输出:
[1 2 0 3 4 0 5 6 0]
[[0 0 1 1]
[0 1 0 1]
[1 0 1 0]
[1 1 0 0]]
[False False True False False True False False True]
[[ True True False False]
[ True False True False]
[False True False True]
[False False True True]]
Number of Zeroes in Array --> 3
Number of Zeroes in Matrix --> 8
该解决方案的核心是以下原则:计算机科学中任何 false
的值都可以表示为 0
,而 true
的值可以表示为非零值。
myArray == 0
语句返回一个数组,其中满足该属性的所有元素均为 True,不满足该条件的元素均为 False。条件本身检查元素是否为零。因此,所有零元素都将变为 True
,现在,我们必须对它们进行计数。为此,我们使用 count_nonzero()
方法。
此处是该功能的官方文档的链接。
使用 where()
计算 NumPy 数组中的零
where()
函数根据指定条件过滤数组中的元素,并返回过滤后的数组。它返回已过滤元素的索引。使用此函数,我们将构建一个仅包含零的数组,而这个新数组的长度将为我提供零计数。
现在,让我们看看解决方案。
import numpy as np
myArray = np.array([1, 2, 0, 3, 4, 0, 5, 6, 0])
myMatrix = np.array([[0, 0, 1, 1], [0, 1, 0, 1], [1, 0, 1, 0], [1, 1, 0, 0]])
print(myArray)
print(myMatrix)
print(myArray[np.where(myArray == 0)])
print(myMatrix[np.where(myMatrix == 0)])
print(f"Number of Zeroes in Array --> {myArray[np.where(myArray == 0)].size}")
print(f"Number of Zeroes in Matrix --> {myMatrix[np.where(myMatrix == 0)].size}")
输出:
[1 2 0 3 4 0 5 6 0]
[[0 0 1 1]
[0 1 0 1]
[1 0 1 0]
[1 1 0 0]]
[0 0 0]
[0 0 0 0 0 0 0 0]
Number of Zeroes in Array --> 3
Number of Zeroes in Matrix --> 8
在上面的代码片段中,我们过滤掉了所有零元素。where()
函数返回这些元素的索引。我们进一步使用这些索引来获取原始元素。显然,它们都将为零。最后,我们计算了这些零的数量并打印了计数。