How to Get NumPy Array Length
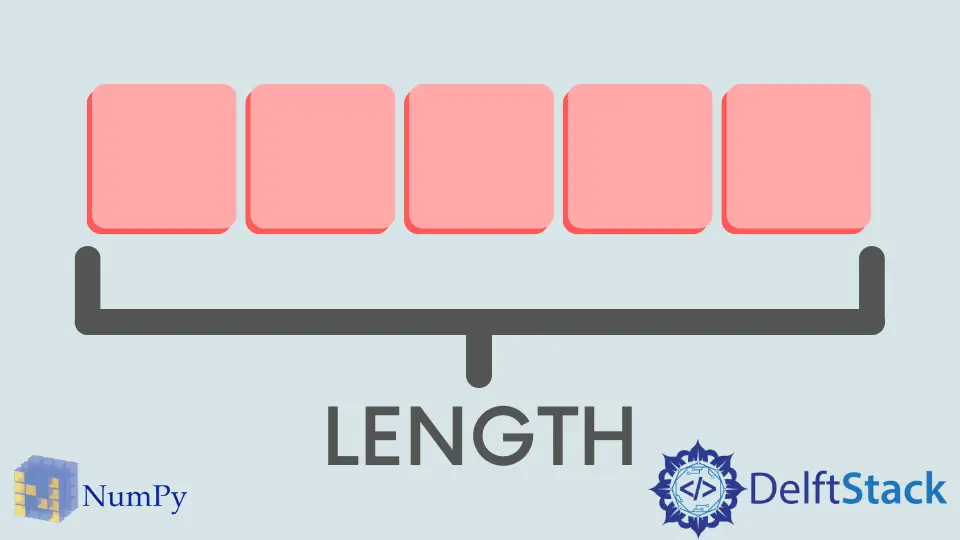
When working with data in Python, especially in the realm of scientific computing, NumPy is an invaluable library. One common task is determining the length of a NumPy array, which can be crucial for various operations, such as data analysis or manipulation. Understanding how to get the length of a NumPy array can streamline your coding process and enhance your efficiency.
In this article, we will explore two primary methods to achieve this: using the numpy.size
property and the numpy.shape
property. Each method has its nuances, and by the end of this guide, you will be equipped with the knowledge to choose the best approach for your needs. Let’s dive in!
Using numpy.size Property
The numpy.size
property is a straightforward way to determine the total number of elements in a NumPy array. This property returns an integer value representing the count of all elements across all dimensions of the array. It is particularly useful when you want a quick overview of the array’s size without delving into its structure.
Here’s how you can use the numpy.size
property:
import numpy as np
array = np.array([[1, 2, 3], [4, 5, 6]])
length = np.size(array)
print(length)
Output:
6
In this example, we first import the NumPy library and create a 2D array. The np.size(array)
function then calculates the total number of elements in the array, which in this case is 6. This is because there are two rows and three columns, leading to a total of 6 elements. The simplicity of using numpy.size
makes it an excellent choice for quick checks on array sizes, especially during exploratory data analysis.
One thing to note is that numpy.size
counts all elements regardless of the array’s shape. This means that whether your array is one-dimensional or multi-dimensional, numpy.size
will always provide you with the total element count. This property is particularly handy when working with larger datasets, as it allows you to quickly gauge the data’s scale without needing to inspect its structure in detail.
Using numpy.shape Property
The numpy.shape
property offers a different perspective by providing the dimensions of the array. Instead of just giving you the total number of elements, numpy.shape
returns a tuple representing the size of each dimension. This is particularly useful when you want to understand the structure of your array, such as how many rows and columns it contains.
Here’s how you can use the numpy.shape
property:
import numpy as np
array = np.array([[1, 2, 3], [4, 5, 6]])
shape = np.shape(array)
print(shape)
Output:
(2, 3)
In this example, after creating the same 2D array, we call np.shape(array)
. The output (2, 3)
indicates that the array has 2 rows and 3 columns. This method is particularly advantageous when you need to perform operations that are sensitive to the array’s dimensions, such as matrix multiplication or reshaping.
Moreover, the numpy.shape
property can be incredibly informative when working with higher-dimensional arrays. For instance, if you have a 3D array, numpy.shape
will provide you with a tuple showing the size of each dimension, allowing you to understand the data’s layout at a glance. This makes numpy.shape
a fundamental tool in any data scientist’s toolkit, as it helps ensure that your data manipulation aligns with the array structure.
Conclusion
In summary, knowing how to get the length of a NumPy array is essential for efficient data manipulation in Python. The two primary methods, numpy.size
and numpy.shape
, offer different insights: numpy.size
gives you a total element count, while numpy.shape
provides the dimensions of the array. Depending on your specific needs, you can choose the method that best suits your task. Mastering these properties will undoubtedly enhance your data analysis skills and streamline your coding process.
FAQ
-
How do I get the length of a one-dimensional NumPy array?
You can use eithernumpy.size
ornumpy.shape
to get the length of a one-dimensional NumPy array. For a one-dimensional array, the two methods will yield the same result. -
Can I use numpy.size for multi-dimensional arrays?
Yes,numpy.size
works for multi-dimensional arrays and will return the total number of elements across all dimensions. -
Is numpy.shape useful for understanding array dimensions?
Absolutely!numpy.shape
returns a tuple that shows the size of each dimension, making it very useful for understanding the structure of your array. -
Are there any performance differences between numpy.size and numpy.shape?
Both methods are efficient, butnumpy.size
may be slightly faster as it directly counts elements, whilenumpy.shape
constructs a tuple of dimensions. -
Can I use these methods on empty arrays?
Yes, bothnumpy.size
andnumpy.shape
can be used on empty arrays, returning 0 and an empty tuple, respectively.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn