How to Add Row to Matrix in NumPy
-
Use the
numpy.vstack()
Function to Add a Row to a Matrix in NumPy -
Use the
numpy.append()
Function to Add a Row to a Matrix in NumPy -
Use the
numpy.r_()
Function to Add a Row to a Matrix in NumPy -
Use the
numpy.insert()
Function to Add a Row to a Matrix in NumPy
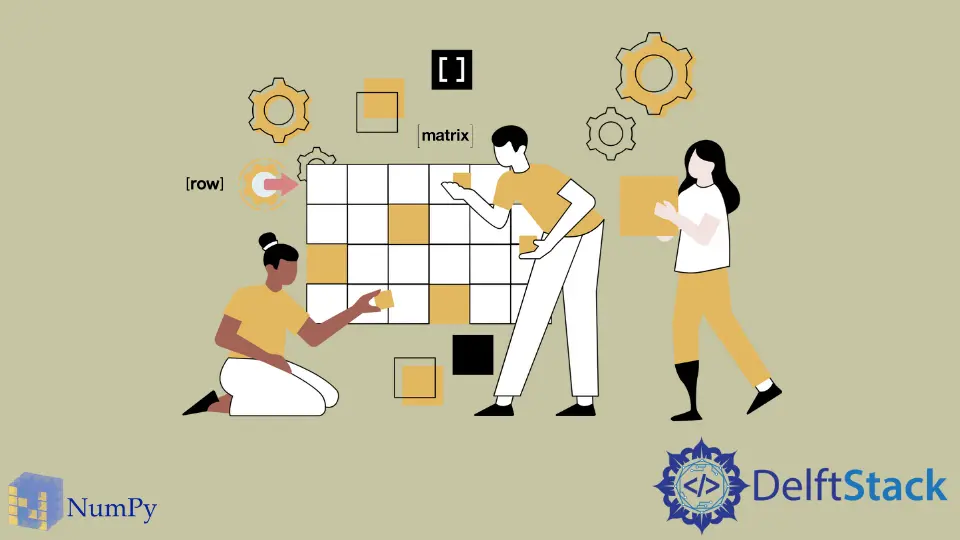
Matrices are often used in mathematics and statistics for data representation and solving multiple linear equations. In programming, a 2-Dimensional array is treated as a matrix.
In Python, the numpy module is used to work with arrays. It has many functions and classes available for performing different operations on matrices.
In this tutorial, we will learn how to add a row to a matrix in numpy.
Use the numpy.vstack()
Function to Add a Row to a Matrix in NumPy
The vstack()
function stacks arrays vertically. Stacking two 2D arrays vertically is equivalent to adding rows to a matrix.
The following code shows this.
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
row = np.array([7, 8, 9])
arr = np.vstack([arr, row])
print(arr)
Output:
[[1 2 3]
[4 5 6]
[7 8 9]]
Use the numpy.append()
Function to Add a Row to a Matrix in NumPy
The append()
function from the numpy module can add elements to the end of the array. By specifying the axis
as 0, we can use this function to add rows to a matrix.
For example,
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
row = np.array([7, 8, 9])
arr = np.append(arr, [row], axis=0)
print(arr)
Output:
[[1 2 3]
[4 5 6]
[7 8 9]]
Use the numpy.r_()
Function to Add a Row to a Matrix in NumPy
The r_()
function from the numpy module concatenates arrays by combining them vertically.
Check the code below to see how we can use this to add rows to a matrix.
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
row = np.array([7, 8, 9])
arr = np.r_[arr, [row]]
print(arr)
Output:
[[1 2 3]
[4 5 6]
[7 8 9]]
Alternatively, we can use the concatenate()
function, too. The concatenate()
function combines two or more arrays so it can be used to achieve the desired result.
For example,
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
row = np.array([7, 8, 9])
arr = np.concatenate((arr, [row]), axis=0)
print(arr)
Output:
[[1 2 3]
[4 5 6]
[7 8 9]]
Use the numpy.insert()
Function to Add a Row to a Matrix in NumPy
The insert()
function adds objects along the specified axis and the position. It can be used to insert a row in a matrix at our desired specific position.
For example,
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
row = np.array([7, 8, 9])
row_n = arr.shape[0] # last row
arr = np.insert(arr, row_n, [row], axis=0)
print(arr)
Output:
[[1 2 3]
[4 5 6]
[7 8 9]]
In the above code, we add the row at the end of the matrix. The shape()
function returns the array’s dimensions, which reveals the total number of rows in the matrix.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn