How to Convert Matrix to Array in NumPy
- Understanding NumPy and Its Importance
- Method 1: Using numpy.array()
- Method 2: Using numpy.reshape()
- Method 3: Using numpy.flatten()
- Conclusion
- FAQ
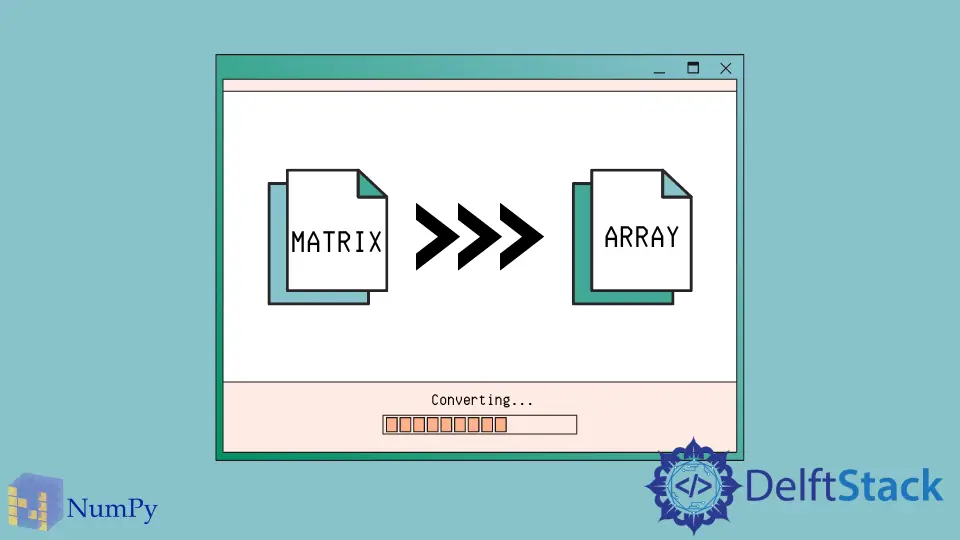
Converting a matrix to an array in Python using NumPy is a fundamental skill for anyone working with numerical data. Whether you’re analyzing datasets, performing mathematical computations, or simply manipulating data structures, understanding how to transform matrices into arrays can significantly enhance your coding efficiency.
In this tutorial, we’ll explore various methods to achieve this conversion seamlessly. We’ll cover the basics of NumPy, provide clear code examples, and explain the steps in a friendly, easy-to-understand manner. By the end of this guide, you’ll be equipped with the knowledge to convert matrices to arrays like a pro!
Understanding NumPy and Its Importance
NumPy is an essential library for numerical computing in Python, providing support for large, multi-dimensional arrays and matrices. It offers a wide range of mathematical functions to operate on these data structures efficiently. When you work with matrices, you often need to convert them to arrays for various operations, as arrays offer more flexibility and functionality.
The conversion process is straightforward, thanks to NumPy’s built-in methods. In this article, we will primarily focus on using the numpy.array()
function and the numpy.reshape()
method to convert matrices into arrays.
Method 1: Using numpy.array()
The most direct way to convert a matrix to an array in NumPy is by using the numpy.array()
function. This method takes an existing matrix as input and returns a new array object.
Here’s how you can do it:
import numpy as np
matrix = [[1, 2, 3], [4, 5, 6]]
array = np.array(matrix)
print(array)
Output:
[[1 2 3]
[4 5 6]]
In this example, we first import the NumPy library and create a simple matrix represented as a list of lists. The numpy.array()
function is then called with the matrix as an argument. This function converts the matrix into a NumPy array, which is printed to the console.
The output shows that the original structure of the matrix is preserved in the resulting array. This method is particularly useful when you need to perform operations on the data, as arrays provide various functionalities that matrices do not. Using numpy.array()
is efficient and straightforward, making it an ideal choice for beginners and experienced programmers alike.
Method 2: Using numpy.reshape()
Another effective way to convert a matrix to an array is by utilizing the numpy.reshape()
method. This method allows you to change the shape of an existing array without changing its data.
Here’s how you can implement reshape
:
import numpy as np
matrix = np.array([[1, 2, 3], [4, 5, 6]])
array = matrix.reshape(-1)
print(array)
Output:
[1 2 3 4 5 6]
In this example, we first create a NumPy array from a matrix. The reshape(-1)
method is then called on this array. The argument -1
tells NumPy to automatically determine the new shape based on the original array’s size. This effectively flattens the 2D matrix into a 1D array.
The output confirms that the matrix has been successfully converted into a one-dimensional array. This method is particularly useful when you want to streamline your data for processing or analysis. Reshaping is a powerful feature in NumPy that allows for greater flexibility in data manipulation, making it a favorite among data scientists and analysts.
Method 3: Using numpy.flatten()
The numpy.flatten()
method is another excellent way to convert a matrix into an array. This method returns a copy of the array collapsed into one dimension, which is particularly useful when you want to simplify the data structure.
Here’s how to use flatten()
:
import numpy as np
matrix = np.array([[1, 2, 3], [4, 5, 6]])
array = matrix.flatten()
print(array)
Output:
[1 2 3 4 5 6]
In this case, we begin by creating a NumPy array from a matrix. By invoking the flatten()
method, we receive a new 1D array that contains all the elements of the original matrix.
The output illustrates that the matrix has been successfully converted into a one-dimensional array. One of the advantages of using flatten()
is that it does not alter the original array, allowing you to maintain your data’s structure while creating a simplified version for specific tasks. This method is particularly handy when you need to process or analyze data in a linear format.
Conclusion
Converting a matrix to an array in NumPy is a straightforward process that can greatly enhance your data manipulation capabilities. In this tutorial, we explored three effective methods: using numpy.array()
, numpy.reshape()
, and numpy.flatten()
. Each method has its unique advantages, depending on your specific needs. By mastering these techniques, you can streamline your data processing tasks and improve the efficiency of your Python code. Whether you’re a beginner or an experienced developer, these tools will empower you to work with numerical data more effectively.
FAQ
-
What is the difference between a matrix and an array in NumPy?
A matrix is a specialized 2D array, while an array can be multi-dimensional. Arrays offer more flexibility in operations. -
Can I convert a multi-dimensional matrix to a 1D array?
Yes, using methods likereshape()
orflatten()
, you can easily convert multi-dimensional matrices to one-dimensional arrays. -
Does the
numpy.array()
method preserve the original matrix structure?
Yes,numpy.array()
maintains the original structure of the matrix when converting it into an array. -
Are there any performance differences between using
flatten()
andreshape()
?
flatten()
creates a copy of the data, whilereshape()
returns a view when possible, which can be more memory efficient. -
Is it necessary to import NumPy to convert a matrix to an array?
Yes, you need to import the NumPy library to access its array manipulation functions.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn