How to Create Empty NumPy Array
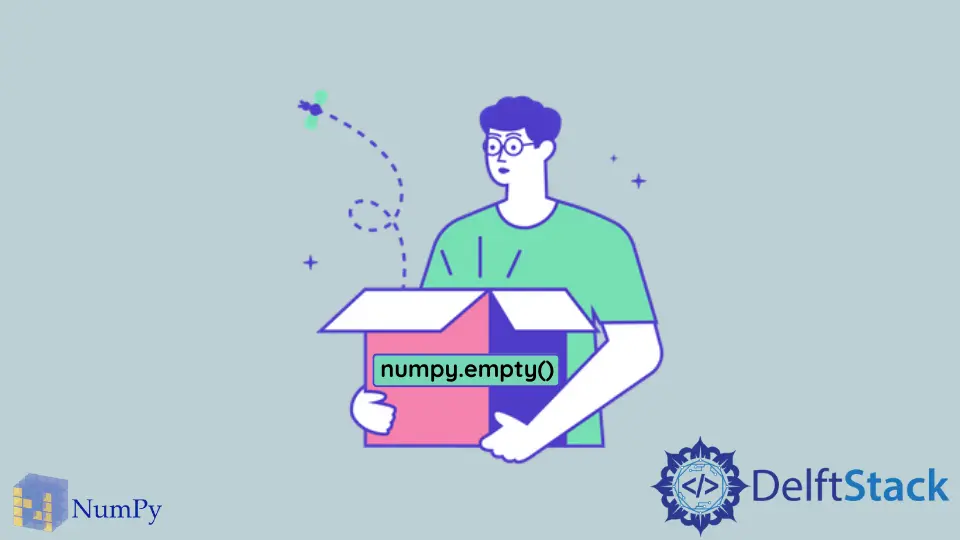
Creating an empty NumPy array is a fundamental task in Python programming, especially in the realm of data science and numerical computing. NumPy, a powerful library for numerical operations, provides several functions to create arrays efficiently. Among these, two popular methods stand out: numpy.zeros()
and numpy.empty()
. Understanding how to use these functions can help streamline your data manipulation processes and enhance your coding efficiency.
In this article, we will delve into both methods, providing clear examples and explanations to ensure you grasp the concepts thoroughly. Whether you are a beginner or looking to refine your skills, this guide will equip you with the knowledge to create empty arrays in NumPy seamlessly.
Method 1: Using numpy.zeros()
The numpy.zeros()
function is a straightforward way to create an empty array filled with zeros. This method is particularly useful when you need to initialize an array of a specific shape and size, with all elements set to zero. Here’s how to use it:
import numpy as np
# Create an empty NumPy array of shape (3, 4)
empty_array_zeros = np.zeros((3, 4))
print(empty_array_zeros)
Output:
[[0. 0. 0. 0.]
[0. 0. 0. 0.]
[0. 0. 0. 0.]]
The numpy.zeros()
function takes a tuple as an argument, representing the desired shape of the array. In the example above, we created a 3x4 array, meaning it has three rows and four columns. All the elements are initialized to zero, which is often a preferred state when preparing data structures for further computation. This method is particularly beneficial in scenarios where you plan to fill the array with values later on, as it provides a clear starting point.
Additionally, you can specify the data type of the array using the dtype
parameter. For instance, if you want the array to contain integers instead of floats, you can modify the function call like this:
empty_array_zeros_int = np.zeros((3, 4), dtype=int)
print(empty_array_zeros_int)
Output:
[[0 0 0 0]
[0 0 0 0]
[0 0 0 0]]
By specifying the dtype
, you ensure that the array is created with the desired type from the get-go, which can save you time and potential errors later in your code.
Method 2: Using numpy.empty()
Another powerful function in the NumPy library is numpy.empty()
. Unlike numpy.zeros()
, which initializes the array elements to zero, numpy.empty()
creates an array without initializing its values. This means that the array will contain whatever values were already in memory at that location. This method is faster than numpy.zeros()
since it skips the initialization step. Here’s how to use it:
import numpy as np
# Create an empty NumPy array of shape (3, 4)
empty_array_empty = np.empty((3, 4))
print(empty_array_empty)
Output:
[[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]
[ 0. 0. 0. 0.]]
In this example, we again create a 3x4 array. However, the values that appear in the output are not guaranteed to be zeros; they are simply whatever values were in memory at the time of creation. This can lead to unexpected results if you attempt to use the array without assigning new values to it. Therefore, while numpy.empty()
is faster and more memory-efficient, it requires you to be cautious about the values it contains.
You can also specify the data type with numpy.empty()
, just like with numpy.zeros()
. Here’s an example:
empty_array_empty_int = np.empty((3, 4), dtype=int)
print(empty_array_empty_int)
Output:
[[ 0 0 0 0]
[ 0 0 0 0]
[ 0 0 0 0]]
Using numpy.empty()
is particularly advantageous when you are planning to fill the array with values immediately after creation, as it saves time by not initializing the elements beforehand. However, it’s crucial to remember that the contents of the array are undefined until you explicitly assign values to them.
Conclusion
Creating empty NumPy arrays is a fundamental skill for anyone working with data in Python. By utilizing the numpy.zeros()
and numpy.empty()
functions, you can efficiently create arrays tailored to your specific needs. While numpy.zeros()
provides a safe starting point with initialized zeros, numpy.empty()
offers speed and efficiency when initialization is not necessary. Understanding the differences and appropriate use cases for each method will enhance your data manipulation capabilities and streamline your coding process. Whether you’re preparing data for analysis or initializing variables for computation, mastering these techniques will undoubtedly make your programming journey smoother.
FAQ
-
What is the difference between numpy.zeros() and numpy.empty()?
numpy.zeros() initializes the array elements to zero, while numpy.empty() creates an array without initializing its values. -
Can I specify the data type when creating an empty array?
Yes, both numpy.zeros() and numpy.empty() allow you to specify the data type using the dtype parameter. -
When should I use numpy.empty() instead of numpy.zeros()?
Use numpy.empty() when you need a faster array creation and plan to fill the array with values immediately after. -
What happens if I use numpy.empty() and do not assign values?
The contents of the array will be undefined and could contain any values that were previously in memory. -
Is it possible to create multi-dimensional empty arrays?
Yes, both numpy.zeros() and numpy.empty() can create multi-dimensional arrays by specifying the shape as a tuple.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn