How to Throw an Error in Node.js
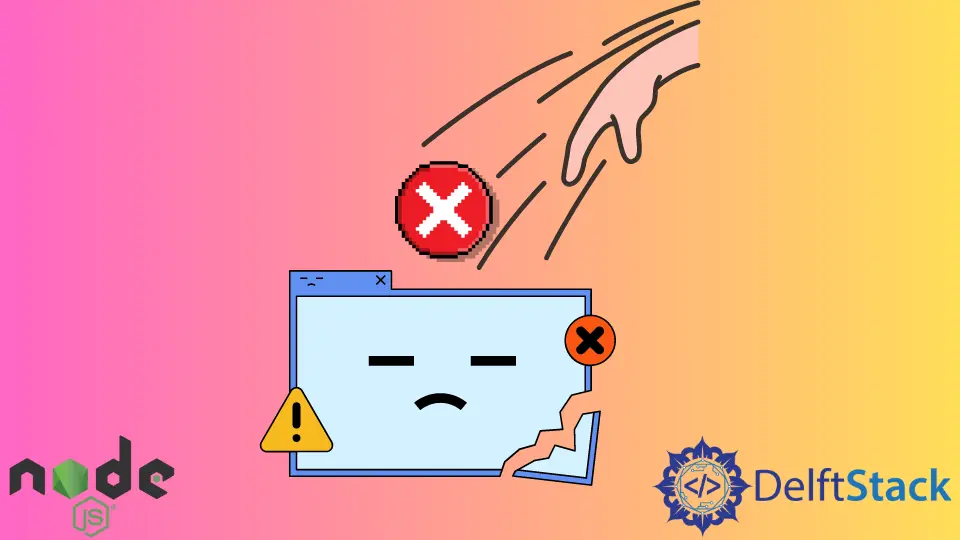
Today’s post will teach us how to throw an error in Node.js.
Throw an Error in Node.js
Errors are statements that do not permit the system to run properly.
Errors in Node.Js are treated through exceptions, which are created with the help of the throw
keyword. This could assist users in creating custom exceptions.
As soon as this line of JavaScript code gets executed, the normal flow of the program stops, and control is held in the nearest exception handler. The program terminates if no catch
block exists between the calling functions.
Syntax:
throw expression;
The value can typically be any JavaScript value in the client-side code, including a number, an object, or a string. But in Node.js, we do not throw strings; we only throw Error
objects.
The Error Object
An error object is an object that is an instance of the Error
object or extends the Error
class provided in the main Error
module. Error objects are generated when runtime errors occur.
We can also use the error object as a base object for custom exceptions.
throw new Error('You reached the end!');
class MyCustomError extends Error {
// ...
}
throw new MyCustomError();
Handle Exceptions
The exception handler is the try...catch
statement. Any exceptions thrown in the lines of code contained in the try
block are handled in the appropriate catch
block.
If an uncaught exception is thrown while your program is running, your program will fail.
try {
/* Your Regular Code */
} catch (e) {
/* Catch your exception here */
}
e
is the exception value in this example. You can add multiple handlers that can detect different types of errors.
Based on the type of exception, you can then modify your program. To fix the program failure issue, wait for the uncaughtException
event on the process object.
process.on('uncaughtException', err => {
console.error('An uncaught error is caught here', err);
process.exit(1);
});
You do not need to import the main process
module for this, as it will be included automatically.
Handle Exceptions With promises
Promises will let you chain one-of-a-kind operations collectively and handle errors at the end. Promise chains are awesome at dealing with error.
When a promise rejects, the control jumps to the nearest rejection handler. That is very convenient in practice.
As you may see, the .catch
doesn’t have to be instant. It may seem after one or maybe numerous .then
.
fooFunction()
.then(barFunction)
.then(fooBarFunction)
.catch(err => console.error(err));
Handle Exceptions With async/await
Using async/await
, you need to catch errors using try...catch
.
async function fooFunction() {
try {
await fooBarFunction();
} catch (err) {
console.error(err.message);
}
}
Let’s understand it with the simple example below:
fooBarFunction =
async () => {
throw new Error('Hello DelftStack Users!')
}
async function fooFunction() {
try {
console.log('Before Error') await fooBarFunction();
} catch (err) {
console.error(err.message);
}
} fooFunction()
In the above example, we call fooFunction()
, which first prints the message and then calls fooBarFunction()
internally. In fooBarFunction()
, we simply throw an error which is caught by fooFunction()
.
Attempt to run the above code snippet in Replit, which supports Node.js. It’s going to display the below result:
Output:
Before Error
Hello DelftStack Users!
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn