How to Sleep in Node.js
-
Use the
setTimeout()
Method to Schedule the Execution of Codes in Node.js -
Use the
setInterval()
Method to Schedule the Execution of Codes in Node.js -
Use the
await()
Keyword to Pause Execution of Codes in Node.js
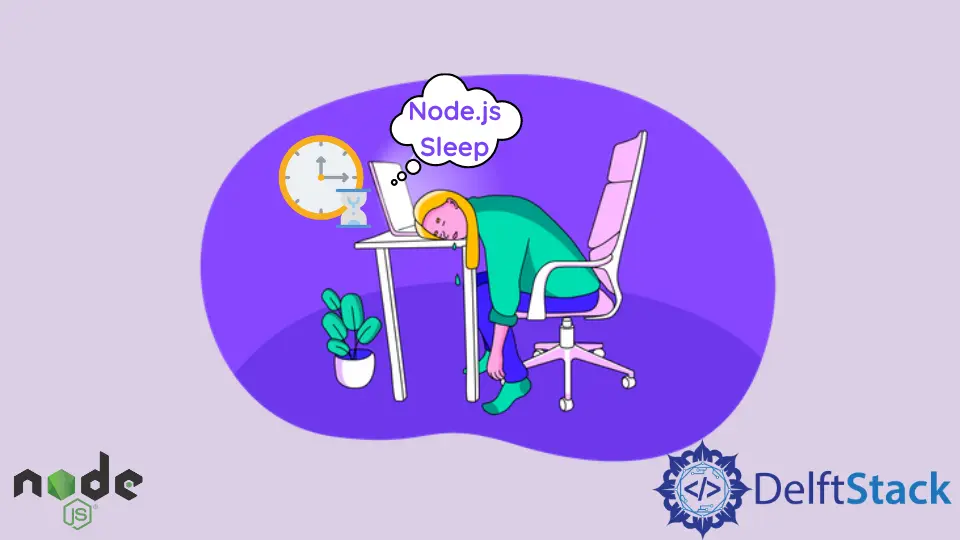
In Node.js (or programming in general), there are scenarios where we need a certain code or script executed periodically. Node.js provides us with two built-in functions that we can use to schedule the execution of functions in Node.js and JavaScript.
These methods are below.
setTimeout()
setInterval()
Use the setTimeout()
Method to Schedule the Execution of Codes in Node.js
The setTimeout()
method is an asynchronous method that allows us to execute a function once after given time intervals. The setTimeout()
function accepts many parameters, two of which are compulsory while the rest are optional.
- The function to be executed after the set time interval
- The number in milliseconds that the timer will wait before executing the specified function
- Some code that will run after the set time is over any other arguments specified in the function to be executed.
This function returns an integer known as the timeout ID
that can reference the object created by the setTimeout()
function.
Here is a simple example of using the setTimeout()
function to execute functions at a different time interval specified as milliseconds.
setTimeout(() => {console.log('This is the first function')}, 2000);
setTimeout(() => {console.log('This is the second function')}, 4000);
setTimeout(() => {console.log('This is the final function')}, 5000);
Sample output:
This is the first function
This is the second function
This is the final function
In the above example, there is a wait time of 2 seconds before the first and second functions are executed and a wait time of 1 second before the last function is executed.
Now, since the setTimeout()
is an asynchronous function, we could instead execute the final function. At the same time, the second function waits in the background and has the first function executed last with a waiting time of 2 seconds between each function.
setTimeout(() => {console.log('This is the first function')}, 6000);
setTimeout(() => {console.log('This is the second function')}, 4000);
setTimeout(() => {console.log('This is the final function')}, 2000);
Sample output:
This is the final function
This is the second function
This is the first function
The setTimeout()
is really useful when used alongside callback functions to set callback functions to execute after some time. Here is a simple callback function set to execute after 4 seconds.
const displayFunction =
() => {
console.log('Code to be executed by the display function!');
// some more code
}
// function may contain more parameters
setTimeout(displayFunction, 4000);
Sample output:
Code to be executed by the display function!
A more precise and preferable approach of rewriting the code using an arrow function is shown below.
setTimeout(() => {console.log('Code to be executed by the function!')}, 4000)
Sample output:
Code to be executed by the function!
Use the setInterval()
Method to Schedule the Execution of Codes in Node.js
The timers module also provides us with the setInterval()
method, whose functionality is similar to the setTimeout()
method. However, unlike the setTimeout()
method, the setInterval()
method allows us to execute a script repeatedly in given intervals until it is explicitly stopped.
The setInterval()
method accepts two compulsory parameters: the function to be executed and the execution time interval. We can also add additional optional parameters to the function.
The method returns an object known as a timer ID that can be used to cancel the timer. Here is a simple example of implementing the setInterval()
method.
setInterval(() => {
console.log('Function repeatedly executed after every 2 seconds!');
}, 2000)
Sample output:
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
^C
This function will repeatedly execute unless interrupted using keyboard interrupt by pressing CTRL+C. A more conventional way of stopping the setInterval()
method after executing a given number of times is by using the clearInterval()
function.
We can do this by passing the ID returned by the setInterval()
method as a parameter to the clearInterval()
as shown below.
const id = setInterval(() => {
console.log('Function repeatedly executed after every 2 seconds!');
}, 2000)
clearInterval(id);
We can also use the setTimeout()
method alongside the clearInterval()
method to stop the setInterval()
method after a given number of seconds.
Here is a simple example of the same.
const id = setInterval(() => {
console.log('Function repeatedly executed after every 2 seconds!');
}, 2000)
setTimeout(
() => {
clearInterval(id),
console.log('Function stopped after 10 seconds!')
},
10000)
Sample output:
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function stopped after 10 seconds!
Use the await()
Keyword to Pause Execution of Codes in Node.js
Unlike other programming languages such as C that provide a sleep
function, which allows us to sleep a given thread while waiting for another to execute, JavaScript doesn’t have this function.
However, using an asynchronous promise-based function, we can use the keyword await()
to pause the execution of a piece of code until that promise is fulfilled first.
We will name this function sleep()
; however, that does not stop you from naming it to any other name that you may find appropriate.
Under the async
function, we have used the keyword await()
to suspend the second console.log()
function until the promise has been resolved; this takes an average of 4 seconds. The value returned by the promise is considered as a return value from the await()
expression.
async function my_asyncFunction() {
console.log('Some Code we want to be executed');
await sleep(4000);
console.log('Some other code we want to be executed after some seconds');
}
function sleep(ms) {
return new Promise((resolve) => {
setTimeout(resolve, ms);
});
}
my_asyncFunction();
Sample output:
Some Code we want to be executed
Some other code we want to be executed after some seconds
We can’t use the await()
expression outside the async
functions in regular Javascript code as of yet. Any attempt to use that syntax returns a syntax error.
async function my_asyncFunction() {
console.log('Some Code we want to be executed');
console.log('Some other code we want to be executed after some seconds');
}
await sleep(4000);
function sleep(ms) {
return new Promise((resolve) => {
setTimeout(resolve, ms);
});
}
my_asyncFunction();
Sample output:
E:\Tonyloi>await.js:7
await sleep(4000);
^^^^^
SyntaxError: await is only valid in async functions and the top level bodies of modules
at Object.compileFunction (node:vm:352:18)
at wrapSafe (node:internal/modules/cjs/loader:1031:15)
at Module._compile (node:internal/modules/cjs/loader:1065:27)
at Object.Module._extensions..js (node:internal/modules/cjs/loader:1153:10)
at Module.load (node:internal/modules/cjs/loader:981:32)
at Function.Module._load (node:internal/modules/cjs/loader:822:12)
at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:81:12)
at node:internal/main/run_main_module:17:47
However, we can use the await expression outside the async
function in JavaScript module syntax.
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn