Node.js でスリープ
-
Node.js で
setTimeout()
メソッドを使用してコードの実行をスケジュールする -
Node.js で
setInterval()
メソッドを使用してコードの実行をスケジュールする -
Node.js で
await()
キーワードを使用してコードの実行を一時停止する
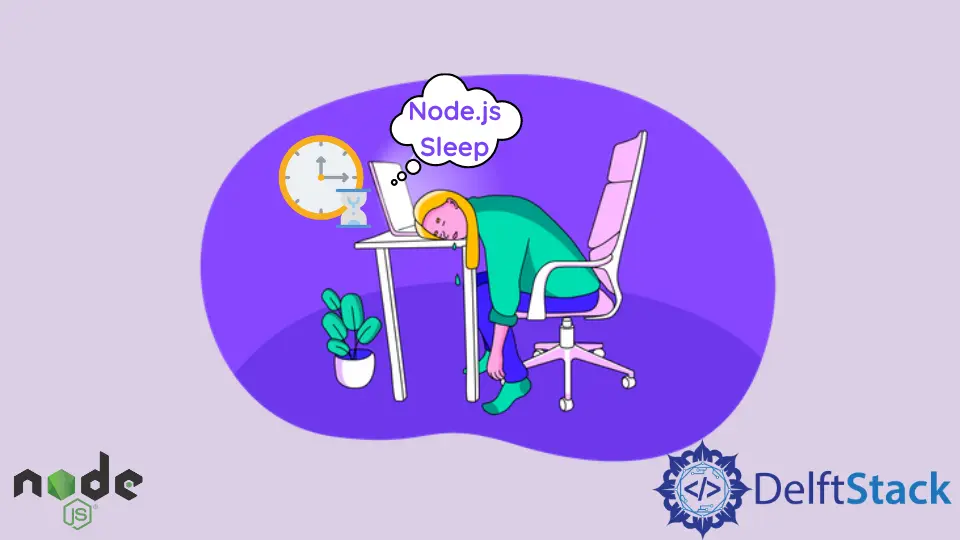
Node.js(または一般的なプログラミング)では、特定のコードまたはスクリプトを定期的に実行する必要があるシナリオがあります。Node.js には、Node.js と JavaScript で関数の実行をスケジュールするために使用できる 2つの組み込み関数が用意されています。
これらの方法は以下のとおりです。
setTimeout()
setInterval()
Node.js で setTimeout()
メソッドを使用してコードの実行をスケジュールする
setTimeout()
メソッドは、指定された時間間隔の後に関数を 1 回実行できるようにする非同期メソッドです。setTimeout()
関数は多くのパラメーターを受け入れます。そのうちの 2つは必須で、残りはオプションです。
- 設定した時間間隔後に実行する機能
- 指定された機能を実行する前にタイマーが待機するミリ秒単位の数
- 設定された時間の後に実行される一部のコードは、実行される関数で指定された他の引数を超えています。
この関数は、setTimeout()
関数によって作成されたオブジェクトを参照できる timeout ID
と呼ばれる整数を返します。
setTimeout()
関数を使用して、ミリ秒単位で指定された異なる時間間隔で関数を実行する簡単な例を次に示します。
setTimeout(() => {console.log('This is the first function')}, 2000);
setTimeout(() => {console.log('This is the second function')}, 4000);
setTimeout(() => {console.log('This is the final function')}, 5000);
サンプル出力:
This is the first function
This is the second function
This is the final function
上記の例では、最初と 2 番目の関数が実行されるまでに 2 秒の待機時間があり、最後の関数が実行されるまでに 1 秒の待機時間があります。
ここで、setTimeout()
は非同期関数であるため、代わりに final 関数を実行できます。同時に、2 番目の関数はバックグラウンドで待機し、最初の関数が最後に実行され、各関数間の待機時間は 2 秒です。
setTimeout(() => {console.log('This is the first function')}, 6000);
setTimeout(() => {console.log('This is the second function')}, 4000);
setTimeout(() => {console.log('This is the final function')}, 2000);
サンプル出力:
This is the final function
This is the second function
This is the first function
setTimeout()
は、コールバック関数と一緒に使用して、しばらくしてから実行するようにコールバック関数を設定する場合に非常に便利です。これは、4 秒後に実行するように設定された単純なコールバック関数です。
const displayFunction =
() => {
console.log('Code to be executed by the display function!');
// some more code
}
// function may contain more parameters
setTimeout(displayFunction, 4000);
サンプル出力:
Code to be executed by the display function!
矢印関数を使用してコードを書き直す、より正確で好ましいアプローチを以下に示します。
setTimeout(() => {console.log('Code to be executed by the function!')}, 4000)
サンプル出力:
Code to be executed by the function!
Node.js で setInterval()
メソッドを使用してコードの実行をスケジュールする
タイマーモジュールは、setInterval()
メソッドも提供します。このメソッドの機能は、setTimeout()
メソッドに似ています。ただし、setTimeout()
メソッドとは異なり、setInterval()
メソッドでは、スクリプトが明示的に停止されるまで、指定された間隔でスクリプトを繰り返し実行できます。
setInterval()
メソッドは、実行される関数と実行時間間隔の 2つの必須パラメーターを受け入れます。関数にオプションのパラメーターを追加することもできます。
このメソッドは、タイマーをキャンセルするために使用できるタイマーID と呼ばれるオブジェクトを返します。これは、setInterval()
メソッドを実装する簡単な例です。
setInterval(() => {
console.log('Function repeatedly executed after every 2 seconds!');
}, 2000)
サンプル出力:
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
^C
この関数は、CTRL + Cを押してキーボード割り込みを使用して中断されない限り、繰り返し実行されます。所定の回数実行した後に setInterval()
メソッドを停止するより一般的な方法は、clearInterval()
関数を使用することです。
これを行うには、以下に示すように、setInterval()
メソッドによって返された ID をパラメーターとして clearInterval()
に渡します。
const id = setInterval(() => {
console.log('Function repeatedly executed after every 2 seconds!');
}, 2000)
clearInterval(id);
setTimeout()
メソッドを clearInterval()
メソッドと一緒に使用して、指定された秒数後に setInterval()
メソッドを停止することもできます。
これは同じものの簡単な例です。
const id = setInterval(() => {
console.log('Function repeatedly executed after every 2 seconds!');
}, 2000)
setTimeout(
() => {
clearInterval(id),
console.log('Function stopped after 10 seconds!')
},
10000)
サンプル出力:
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function repeatedly executed after every 2 seconds!
Function stopped after 10 seconds!
Node.js で await()
キーワードを使用してコードの実行を一時停止する
別のスレッドが実行されるのを待っている間に特定のスレッドをスリープできる sleep
関数を提供する C などの他のプログラミング言語とは異なり、JavaScript にはこの関数がありません。
ただし、非同期の Promise ベースの関数を使用すると、キーワード await()
を使用して、その Promise が最初に実行されるまでコードの実行を一時停止できます。
この関数に sleep()
という名前を付けます。ただし、それでも、適切と思われる他の名前に名前を付けることはできます。
async
関数の下で、キーワード await()
を使用して、promise が解決されるまで 2 番目の console.log()
関数を一時停止しました。これには平均 4 秒かかります。promise によって返される値は、await()
式からの戻り値と見なされます。
async function my_asyncFunction() {
console.log('Some Code we want to be executed');
await sleep(4000);
console.log('Some other code we want to be executed after some seconds');
}
function sleep(ms) {
return new Promise((resolve) => {
setTimeout(resolve, ms);
});
}
my_asyncFunction();
サンプル出力:
Some Code we want to be executed
Some other code we want to be executed after some seconds
現在のところ、通常の Javascript コードでは async
関数の外で await()
式を使用することはできません。その構文を使用しようとすると、構文エラーが返されます。
async function my_asyncFunction() {
console.log('Some Code we want to be executed');
console.log('Some other code we want to be executed after some seconds');
}
await sleep(4000);
function sleep(ms) {
return new Promise((resolve) => {
setTimeout(resolve, ms);
});
}
my_asyncFunction();
サンプル出力:
E:\Tonyloi>await.js:7
await sleep(4000);
^^^^^
SyntaxError: await is only valid in async functions and the top level bodies of modules
at Object.compileFunction (node:vm:352:18)
at wrapSafe (node:internal/modules/cjs/loader:1031:15)
at Module._compile (node:internal/modules/cjs/loader:1065:27)
at Object.Module._extensions..js (node:internal/modules/cjs/loader:1153:10)
at Module.load (node:internal/modules/cjs/loader:981:32)
at Function.Module._load (node:internal/modules/cjs/loader:822:12)
at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:81:12)
at node:internal/main/run_main_module:17:47
ただし、JavaScript モジュール構文の async
関数の外で await 式を使用できます。
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn