How to Encode Base64 in Node.js
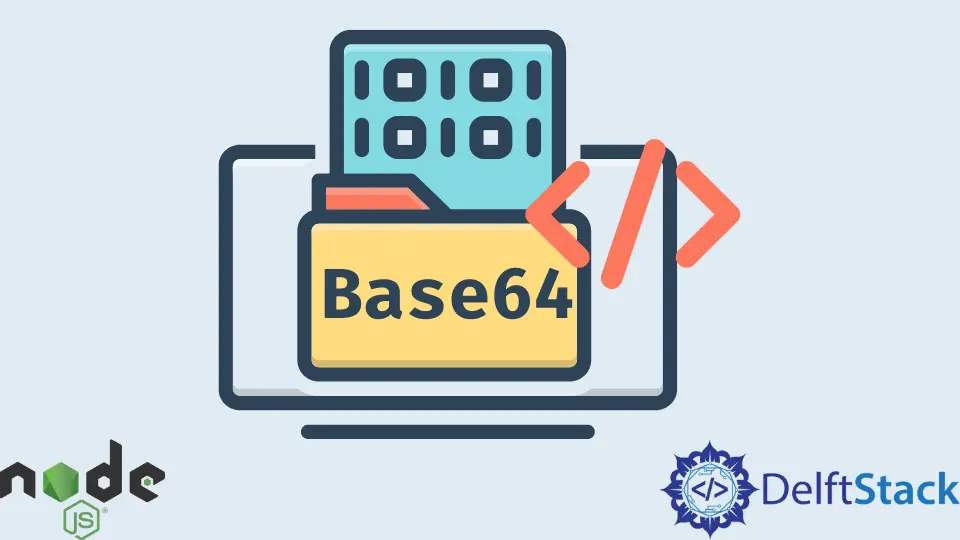
In this short article, we’ll learn how to convert strings or text into base64 in Node.js.
Base64 Encoding in Node.js
Buffer objects
are utilized to represent a fixed-length sequence of bytes. A large number of Node.js APIs support buffers.
A string can be converted into a sequence of bytes using the Buffer.from()
method, which takes the string to be converted and the string’s current encoding. You can specify this encoding as UTF8.
The JavaScript class Uint8Array
is a Buffer class
subclass, which adds new methods to meet various use situations. Simple Uint8Arrays
are accepted by Node.js APIs as long as buffers are supported.
Although the Buffer class
is accessible in the global scope, it is still advised to use an import or need a statement to explicitly reference it. Decoding is turning a buffer into a string using any method, and encoding is turning a string into a buffer.
The function toString()
method can then be used to return the converted bytes as a base64
. The encoding required during conversion is specified via a parameter accepted by this procedure.
The following are additional binary-to-text
encodings that Node.js supports.
base64
base64url
hex
The Base64 encoding accepts the URL and Filename Safe Alphabet described in RFC 4648 Section 5
when constructing a buffer from a string. Whitespace in the base64-encoded string, including spaces, tabs, and newlines.
Let’s understand it with a simple example.
Code:
console.log(Buffer.from('Hello DelftStack Readers!').toString('base64'));
console.log(Buffer.from('SGVsbG8gRGVsZnRTdGFjayBSZWFkZXJzIQ==', 'base64')
.toString('ascii'));
We passed the text to buffer and expected it to encode it into base64. In the next line of code, we are decoding the buffer from base64
to ascii
which is your plain text.
Try to run the above example in a compiler that supports Node.js,
Output:
SGVsbG8gRGVsZnRTdGFjayBSZWFkZXJzIQ==
Hello DelftStack Readers!
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn