How to Send File to Client in Node.js
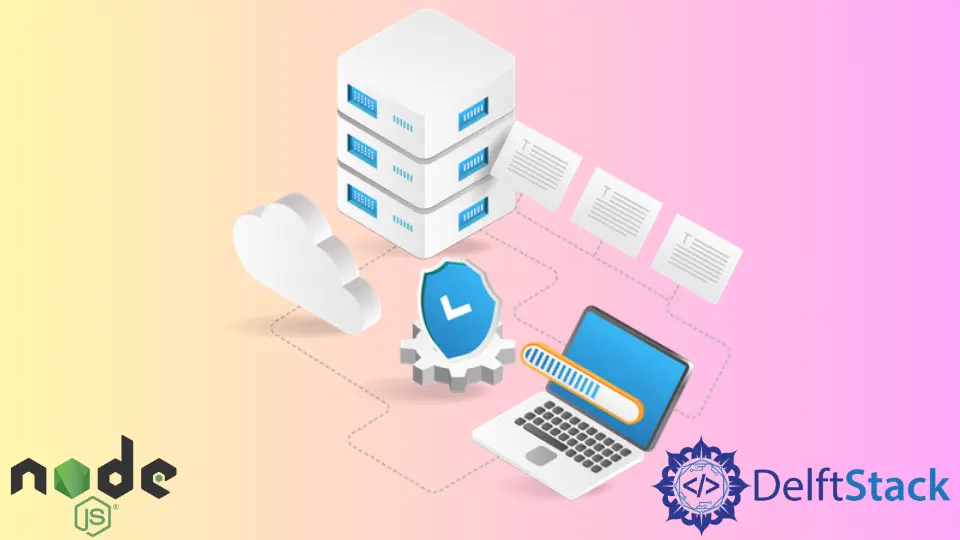
In this article, we’ll learn about sending files to the client in Node.js using Express.
Use Express to Send File in Node.js
Express.js or Express is a back-end web utility framework for Node.js. Express is a Node.js web application framework that provides a robust characteristic set for web and mobile applications.
The function res.sendFile()
passes the file in the specified path and sets the HTTP header
field for the content-type
response based on the filename extension.
Syntax:
res.sendFile(path[, options][, fn])
Parameters | Description |
---|---|
path |
A mandatory parameter that describes the file’s path that needs to be sent. |
options |
An optional parameter that contains various properties like maxAge , root , etc., of the file being sent. |
fn |
A callback function invoked when the file. |
Follow the instructions below for sending the file to the client.
-
Install the Express package.
$ npm install express
-
Create an
index.js
file and run the below command.
```cmd
node index.js
```
-
Create a
helloworld.txt
file.Hello World!
-
Run the
index.js file
by passing the code snippet below.
Complete Source Code - index.js
:
const express = require('express');
const app = express();
const path = require('path');
const PORT = 3001;
app.get('/', (req, res, next) => {
const fileName = 'helloworld.txt';
res.sendFile(fileName, {root: path.join(__dirname)}, (err) => {
if (err) {
next(err);
} else {
console.log('File Sent:', fileName);
}
});
});
app.listen(PORT, (err) => {
if (err) console.log(err);
console.log('Server listening on PORT', PORT);
});
In the above example, we created a server that listens on the specified port 3001
. Once the server listens to the specified port, it will execute the code inside the first matching route.
The response object will then be returned to the client with the sendFile()
method. If any error is encountered, it will pass the error to the error handler using the next()
method.
If everything is smooth, it will return the response object to the client with the file’s content.
Try to run the above code in replit, which supports Node.js, and it will display the below result.
Output:
Server listening on PORT 3001
File Sent: helloworld.txt
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn