Multithreading in Node.js
- Understanding Multithreading in Node.js
- Setting Up the Environment
- Using Worker Threads for Multithreading
- Managing Multiple Workers
- Conclusion
- FAQ
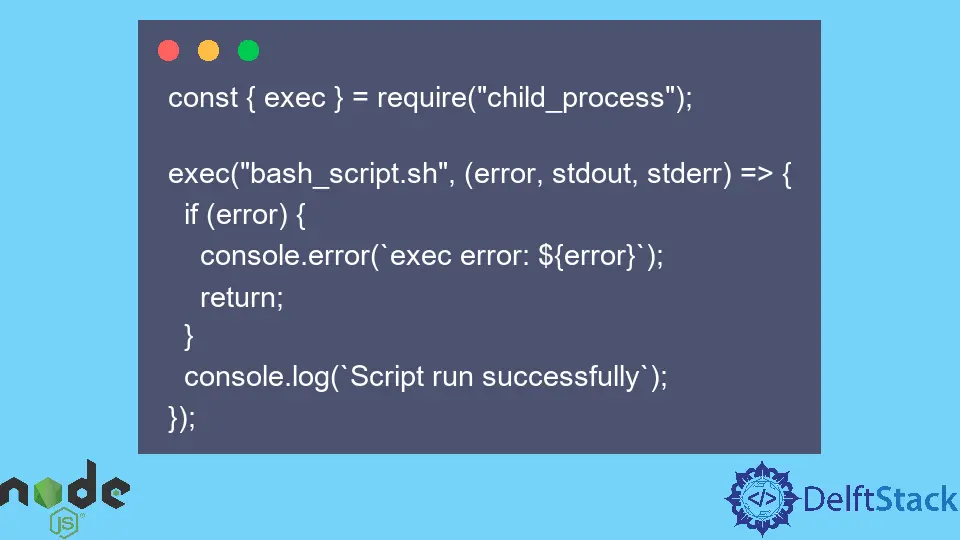
In this guide, we will learn about multithreading in Node.js. As web applications become more complex and demanding, the need for efficient handling of concurrent tasks is paramount. Node.js, traditionally known for its single-threaded event loop, has evolved to support multithreading through various modules and techniques. Understanding how to leverage multithreading can significantly enhance the performance of your applications, allowing them to handle multiple tasks simultaneously without compromising speed or efficiency. Whether you’re working on a large-scale application or a small project, mastering multithreading in Node.js can set you apart as a developer. Let’s dive into the intricacies of this powerful feature.
Understanding Multithreading in Node.js
Node.js is built on a single-threaded architecture, which means it processes requests one at a time. However, this doesn’t mean it cannot handle multiple operations simultaneously. The introduction of the Worker Threads module allows developers to create multiple threads, enabling true parallel execution of JavaScript code. This is particularly useful for CPU-intensive operations that can block the event loop, causing performance bottlenecks.
With multithreading, tasks can be distributed across multiple threads, allowing for better resource utilization and faster execution times. This guide will explore how to implement multithreading in Node.js using the Worker Threads module, providing you with practical examples to enhance your understanding.
Setting Up the Environment
Before diving into multithreading, ensure you have Node.js installed on your machine. You can check your Node.js version by running the following command in your terminal:
node -v
If you need to install Node.js, visit the official Node.js website and download the latest version. Once installed, you can start creating a new Node.js application by initializing a new project:
mkdir my-multithreading-app
cd my-multithreading-app
npm init -y
This sets up a new project where you can experiment with multithreading.
Using Worker Threads for Multithreading
The Worker Threads module is the primary way to achieve multithreading in Node.js. This module allows you to create threads that can run JavaScript operations in parallel. Here’s a simple example of how to use Worker Threads.
First, create a file called worker.js
:
const { parentPort } = require('worker_threads');
parentPort.on('message', (num) => {
let result = 0;
for (let i = 0; i < num; i++) {
result += i;
}
parentPort.postMessage(result);
});
In this code, we set up a worker that listens for messages from the parent thread. When it receives a number, it calculates the sum of all integers up to that number and sends the result back.
Next, create a file called main.js
:
const { Worker } = require('worker_threads');
function runService(num) {
return new Promise((resolve, reject) => {
const worker = new Worker('./worker.js');
worker.postMessage(num);
worker.on('message', resolve);
worker.on('error', reject);
worker.on('exit', (code) => {
if (code !== 0) {
reject(new Error(`Worker stopped with exit code ${code}`));
}
});
});
}
runService(100000000).then(result => {
console.log(`Result: ${result}`);
}).catch(err => {
console.error(err);
});
In main.js
, we create a new Worker instance, passing the path to worker.js
. We then send a number to the worker and listen for the result. If the worker exits with an error, we handle it gracefully.
Output:
Result: 4999999950000000
This example demonstrates how to offload CPU-intensive tasks to a worker thread, allowing the main thread to remain responsive. By using Worker Threads, you can significantly improve the performance of your Node.js applications.
Managing Multiple Workers
In many cases, you may want to leverage multiple workers to handle various tasks concurrently. This can be particularly beneficial when dealing with large datasets or complex computations. Here’s how to manage multiple workers effectively.
Modify the main.js
file to create multiple workers:
const { Worker } = require('worker_threads');
function runService(num) {
return new Promise((resolve, reject) => {
const worker = new Worker('./worker.js');
worker.postMessage(num);
worker.on('message', resolve);
worker.on('error', reject);
worker.on('exit', (code) => {
if (code !== 0) {
reject(new Error(`Worker stopped with exit code ${code}`));
}
});
});
}
async function runMultipleServices() {
const numbers = [100000000, 200000000, 300000000];
const promises = numbers.map(num => runService(num));
const results = await Promise.all(promises);
console.log(results);
}
runMultipleServices().catch(err => {
console.error(err);
});
In this updated code, we define an async
function runMultipleServices
that creates an array of numbers. We then use Promise.all()
to run multiple workers concurrently, fetching results from each worker.
Output:
[ 4999999950000000, 29999999950000000, 449999999850000000 ]
This approach allows you to efficiently manage multiple workers, making your application capable of handling more tasks at once. By distributing workloads across multiple threads, you can achieve significant performance gains.
Conclusion
Multithreading in Node.js opens up a world of possibilities for optimizing application performance. By utilizing the Worker Threads module, developers can offload CPU-intensive tasks, allowing for a more responsive and efficient application. As we’ve explored, setting up multithreading is straightforward and can lead to remarkable improvements in processing speed. Whether you’re building a small application or a large-scale service, understanding multithreading in Node.js is essential for any modern developer.
FAQ
-
What is multithreading in Node.js?
Multithreading in Node.js refers to the ability to execute multiple threads simultaneously, allowing for parallel processing of tasks. -
How does Node.js handle multithreading?
Node.js uses the Worker Threads module to create and manage multiple threads, enabling true parallel execution of JavaScript code. -
When should I use multithreading in Node.js?
You should consider using multithreading for CPU-intensive tasks that may block the event loop, such as complex calculations or data processing. -
Can I use multiple workers in Node.js?
Yes, you can create multiple worker instances to handle various tasks concurrently, improving the overall performance of your application. -
Is multithreading in Node.js suitable for all applications?
While multithreading can enhance performance, it may not be necessary for all applications. It is most beneficial for CPU-bound tasks rather than I/O-bound tasks.
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn