HTTP Post Request in Node.js
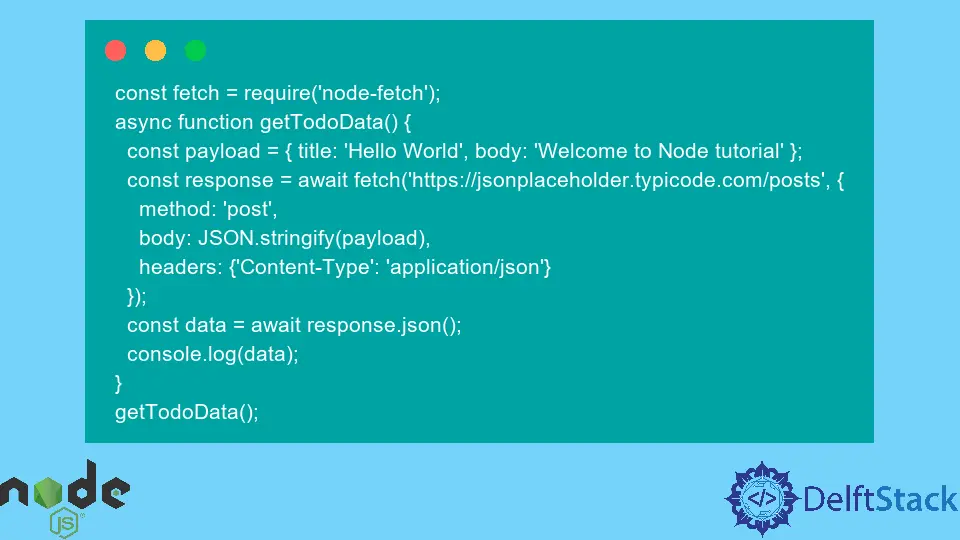
In this article, we’ll learn how to make post requests using third-party packages using Node.js.
HTTP Post Request in Node.js
The HTTP POST
method creates or adds a resource on the server. The main difference between POST
and PUT
requests is that a new resource is added/created to the server via a POST
request, whereas an existing resource is updated/replaced via a PUT
request.
For example, browsers use the HTTP POST
request method when sending HTML form data to the server or when sending data via jQuery/AJAX requests. Unlike GET and HEAD requests, HTTP POST
requests can change the server’s state.
Different Ways to Make HTTP Requests in Node.js
Use Axios Library
You can send asynchronous HTTP requests to REST
endpoints using Axios. Performing CRUD operations becomes an easy task using Axios.
Use the following command to install the Axios library.
$ npm i axios
A POST
request is created with the post()
method. When it’s passed as the second parameter to the post()
function, Axios automatically serializes JavaScript objects to JSON.
We don’t need to serialize POST bodies to JSON.
Complete Source Code:
const axios = require('axios');
async function submitRequest() {
const payload = {title: 'Hello World', body: 'Welcome to Node tutorial'};
const res =
await axios.post('https://jsonplaceholder.typicode.com/posts', payload);
const data = res.data;
console.log(data);
}
submitRequest();
In the above example, once the user submits the form, a POST call is sent to the node server with the specified URL(dummy in this post) and parameters. If the server processes this data without interruption, it returns a successful message.
Based on the output of the server response, you can print the message on the console or notify the user with the message.
Output:
{
title: 'Hello World',
body: 'Welcome to Node tutorial',
id: 101
}
Use Node-fetch Library
You can send asynchronous HTTP requests to REST
endpoints using the node-fetch library. You can find more information about the node-fetch here
.
Install the node-fetch library using the following command.
$ npm i node - fetch
Complete Source Code:
const fetch = require('node-fetch');
async function getTodoData() {
const payload = {title: 'Hello World', body: 'Welcome to Node tutorial'};
const response = await fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'post',
body: JSON.stringify(payload),
headers: {'Content-Type': 'application/json'}
});
const data = await response.json();
console.log(data);
}
getTodoData();
Output:
{
title: 'Hello World',
body: 'Welcome to Node tutorial',
id: 101
}
Use SuperAgent Library
Let’s use the SuperAgent library to make an HTTP post request in Node.js. You can find more information about the SuperAgent library here
.
Install the superagent
library using the following command.
$ npm i superagent
Complete Source Code:
const superagent = require('superagent');
async function getTodoData() {
const payload = {title: 'Hello World', body: 'Welcome to Node tutorial'};
const res =
await superagent.post('https://jsonplaceholder.typicode.com/posts')
.send(payload);
console.log(res.body);
}
getTodoData();
Output:
{
title: 'Hello World',
body: 'Welcome to Node tutorial',
id: 101
}
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn