How to Call REST API in Node.js
- Understanding REST APIs
- Method 1: Using the Built-in HTTP Module
- Method 2: Using Axios for Simplicity
- Method 3: Using Fetch API with Node.js
- Conclusion
- FAQ
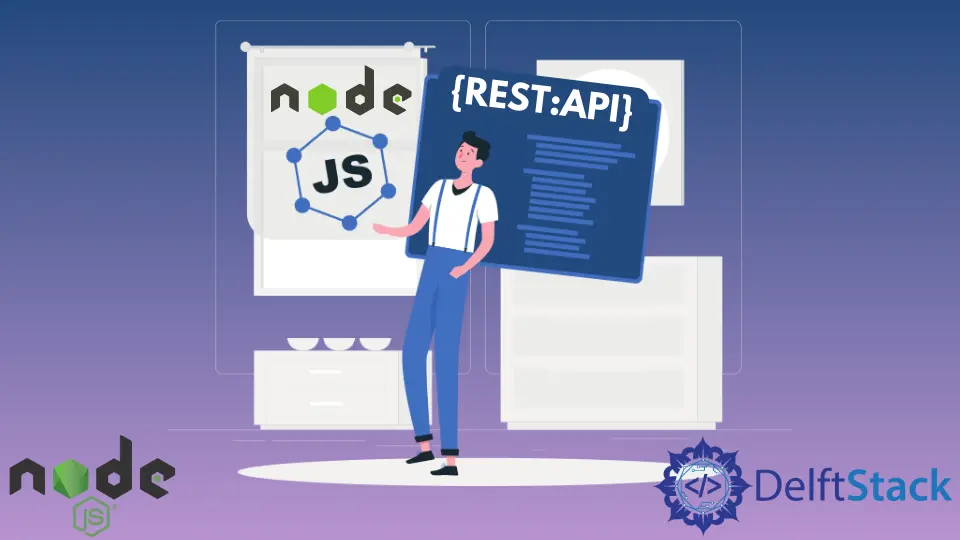
In today’s post, we’ll learn about how to call REST API in Node.js. As a popular JavaScript runtime, Node.js is widely used for building server-side applications, and interacting with REST APIs is a common task developers face. Whether you’re fetching data from a third-party service or integrating with your own backend, knowing how to make HTTP requests is essential. In this guide, we’ll explore various methods to call REST APIs using Node.js, complete with code examples and detailed explanations. By the end of this article, you’ll have a solid understanding of how to effectively interact with REST APIs in your Node.js applications.
Understanding REST APIs
Before diving into the code, let’s briefly discuss what REST APIs are. REST, which stands for Representational State Transfer, is an architectural style that defines a set of constraints for creating web services. REST APIs allow different applications to communicate over HTTP, using standard methods like GET, POST, PUT, and DELETE. They are stateless and provide a lightweight way to exchange data, often in JSON format. Understanding these fundamentals will help you better grasp how to interact with REST APIs in Node.js.
Method 1: Using the Built-in HTTP Module
Node.js comes with a built-in HTTP module that allows you to make HTTP requests without any external libraries. This method is straightforward and perfect for basic API interactions.
const http = require('http');
const options = {
hostname: 'jsonplaceholder.typicode.com',
port: 80,
path: '/posts/1',
method: 'GET',
};
const req = http.request(options, (res) => {
let data = '';
res.on('data', (chunk) => {
data += chunk;
});
res.on('end', () => {
console.log(JSON.parse(data));
});
});
req.on('error', (error) => {
console.error(`Error: ${error.message}`);
});
req.end();
Output:
{ userId: 1, id: 1, title: 'sunt aut facere repellat provident occaecati excepturi optio reprehenderit', body: 'quia et suscipit\nsuscipit...'}
The above code uses Node.js’s built-in HTTP module to make a GET request to a public API. We define an options object that specifies the hostname, port, path, and HTTP method. The http.request
method creates a request object, allowing us to listen for data events. As data is received, it accumulates in the data
variable. Once the response ends, we parse the JSON and log it to the console. This method is efficient for simple tasks but can become cumbersome for more complex interactions.
Method 2: Using Axios for Simplicity
While the built-in HTTP module is effective, many developers prefer using libraries like Axios for making HTTP requests due to its simplicity and additional features.
const axios = require('axios');
axios.get('https://jsonplaceholder.typicode.com/posts/1')
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(`Error: ${error.message}`);
});
Output:
{ userId: 1, id: 1, title: 'sunt aut facere repellat provident occaecati excepturi optio reprehenderit', body: 'quia et suscipit\nsuscipit...'}
In this example, we use Axios to make a GET request to the same API. Axios simplifies the process by returning a promise, which we can handle with .then()
and .catch()
. When the request is successful, the response data is logged to the console. Axios also automatically transforms response data into JSON, eliminating the need for manual parsing. This method is highly recommended for its ease of use, especially when working with larger applications or more complex API interactions.
Method 3: Using Fetch API with Node.js
The Fetch API is another popular way to make HTTP requests, though it’s more commonly associated with the browser. However, you can also use it in Node.js by installing the node-fetch
package.
const fetch = require('node-fetch');
fetch('https://jsonplaceholder.typicode.com/posts/1')
.then((response) => response.json())
.then((data) => {
console.log(data);
})
.catch((error) => {
console.error(`Error: ${error.message}`);
});
Output:
{ userId: 1, id: 1, title: 'sunt aut facere repellat provident occaecati excepturi optio reprehenderit', body: 'quia et suscipit\nsuscipit...'}
This code snippet demonstrates how to use the Fetch API in Node.js. Similar to Axios, Fetch returns a promise. We call the .json()
method on the response object to parse the JSON data. This method is sleek and modern, making it popular among developers who prefer a more native approach to handling HTTP requests. However, keep in mind that Fetch does not reject the promise on HTTP errors, so you may need to implement additional error handling depending on your needs.
Conclusion
In this article, we’ve explored various methods to call REST APIs in Node.js, including using the built-in HTTP module, Axios, and the Fetch API. Each method has its advantages and is suited for different use cases. Whether you prefer the simplicity of Axios or the native feel of the Fetch API, knowing how to interact with REST APIs is a crucial skill for any Node.js developer. By mastering these techniques, you’ll be well-equipped to build robust applications that communicate effectively with external services.
FAQ
-
What is a REST API?
A REST API is an architectural style for designing networked applications, allowing different systems to communicate over HTTP using standard methods. -
Why should I use Axios instead of the built-in HTTP module?
Axios simplifies the process of making HTTP requests by returning promises and automatically handling JSON data, making it easier to work with. -
Can I use the Fetch API in Node.js?
Yes, you can use the Fetch API in Node.js by installing thenode-fetch
package, allowing you to make HTTP requests in a modern way. -
What are the common HTTP methods used in REST APIs?
The common HTTP methods are GET, POST, PUT, DELETE, PATCH, and OPTIONS, each serving different purposes for interacting with resources. -
How do I handle errors when calling a REST API?
You can handle errors by using.catch()
with promises or by checking the response status in the case of the Fetch API or Axios.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn