AJAX Call in Node.js
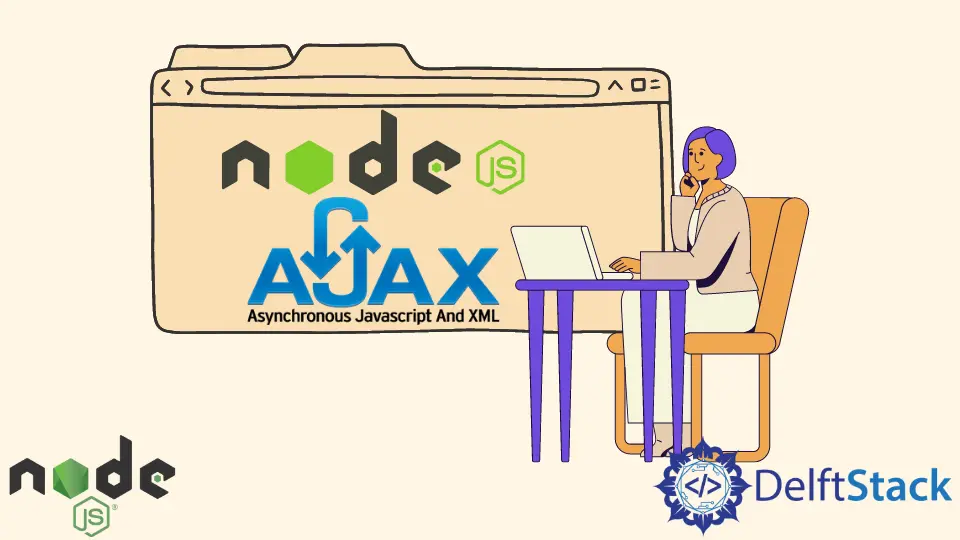
Representational State Transfer
is referred to as REST
. An API or web API (Application Programming Interface) that complies with the limits and restrictions of the REST
architectural style and allows interaction with RESTful web services is known as a REST
API or RESTful
API.
In today’s post, we’ll learn how to make requests using third-party packages using Node.js.
AJAX Call in Node.js
The node-fetch
is a lightweight package/module that allows us to use the fetch()
function in Node.js. Its functionality is very similar to window.fetch()
in Vanilla JavaScript.
The node-fetch
is a free third-party package, a Promise-based HTTP client for the browser and Node.js. It is available on NPM
.
You can send asynchronous HTTP requests to REST
endpoints using node-fetch
. Performing CRUD operations becomes an easy task using node-fetch
.
We can use this in Vanilla JavaScript or with a library like Node.js or React. You can find more information about node-fetch
here.
The HTTP GET
method fetches resources from the server. For example, browsers use the HTTP GET
request method when fetching the TODO
data list from the server or fetching information of specific TODO
requests.
GET
and HEAD
requests do not change the server’s state.
GET
APIs are idempotent, which means making multiple identical requests always produce the same result every time until another API such as POST
or PUT
is made to the server, which changes the resource’s state on the server.
Install the node-fetch
library using the following command $ npm i node-fetch
.
A GET
request is created with the get
method.
const fetch = require('node-fetch');
async function getTodoData() {
const payload = {title: 'Hello World', body: 'Welcome to Node tutorial'};
const response = await fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'post',
body: JSON.stringify(payload),
headers: {'Content-Type': 'application/json'}
});
const data = await response.json();
console.log(data);
}
getTodoData();
In the example above, once the user runs the file, a GET
call is sent to the Node server using the fetch
module with the specified URL (dummy in this post). If the server processes this data without interruption, it returns a successful message.
Based on the output of the server response, you can print the message on the console or notify the user with the appropriate message.
Attempt to run the above code snippet in replit
, which supports Node.js; it will display the result below.
{ title: 'Hello World', body: 'Welcome to Node tutorial', id: 101 }
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn