How to Make HTTP Request to Get Data Using Node.js
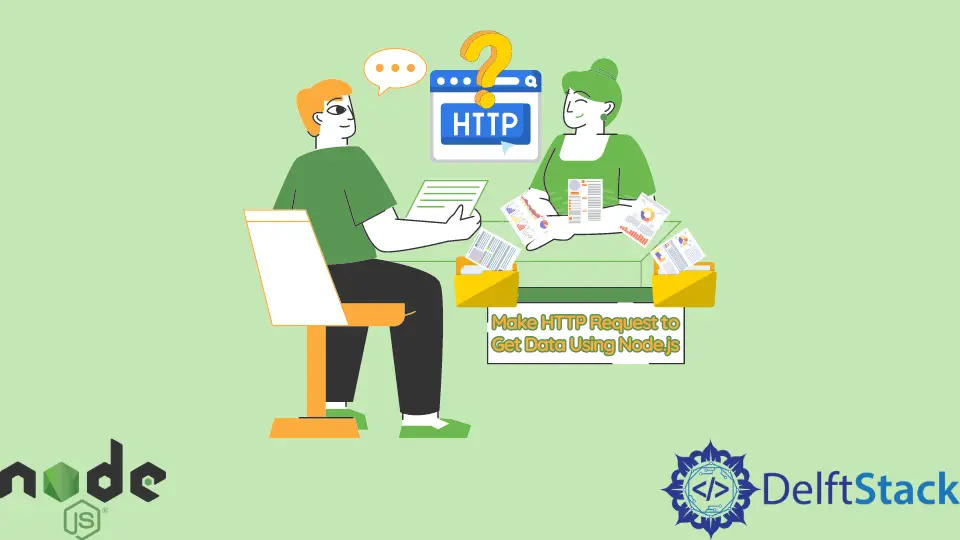
In today’s article, we’ll learn how to make the HTTP request and get the data using node.js.
HTTP Request to Get Data Using Node.js
We can use built-in modules in node.js to send HTTP requests. Callbacks are used by these standard modules to process the request and obtain the data.
Axios
, node-fetch
, super agent
, and other modules can also be used to send HTTP requests. The HTTPs module makes the call using the request
function.
The options object, which provides the hostname
, port
number, and path
of the server URL, and the kind of request, is passed as an argument to this request function.
The callback
function is the second argument. When the request is handled, this callback
function is activated. The HTTPs module has several events that can handle the requests and get the requested data.
For instance, to end a request, use req.end()
and req.on('error')
in the event of an error. After getting the request, the server processes the data and sends back the response in chunks.
When a response-related event occurs, such as res.on('data')
or res.on('end')
, we can retrieve the response. When the complete response is returned in the end event, we can print it to the console after concatenating the chunk of data in the data event.
Because https.request
is asynchronous
, it will return undefined if we attempt to read the server response before the request has finished. Let’s understand the above process in a simple example below.
Example Code:
const https = require('https');
const options = {
hostname: 'jsonplaceholder.typicode.com',
port: 443,
path: '/posts/1',
method: 'GET',
};
function submitRequest() {
const req = https.request(options, res => {
let outputData = ''
console.log(`statusCode: ${res.statusCode}`);
res.on('data', dataChunk => {
outputData += dataChunk;
});
res.on('end', () => {
console.log('outputData', outputData);
});
});
req.on('error', error => {
console.error('error', error);
});
req.end();
}
submitRequest()
In the code example above, we send a GET
request to the mock server by specifying the path
and a port
number. After getting the request and processing the data, the server fragments the response and sends it back.
We can concatenate the response data and print it to the console once the response has been entirely returned in the end method.
Run the above line of code in Replit
, which is compatible with node.js. It will display the following outcome. You can also find the demo for the above code here.
Output:
statusCode: 200
outputData {
"userId": 1,
"id": 1,
"title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit",
"body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto"
}
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn