How to Format Dates in NodeJS
-
Use the
new Date()
Object to Create a New Date Object in NodeJS -
Use the
getter
Function to Get Specific Date Object in NodeJS -
the
YYYY-MM-DD hh:mm:ss
Combination Using theget
Function in NodeJS -
Use the
require()
Method to Print the Current Local Date-Time in NodeJS -
Format a Date Object Using the
YYYY-MM-DD hh:mm:ss
Function -
Use the
toString()
Method to Format Date Object Into a String in NodeJS
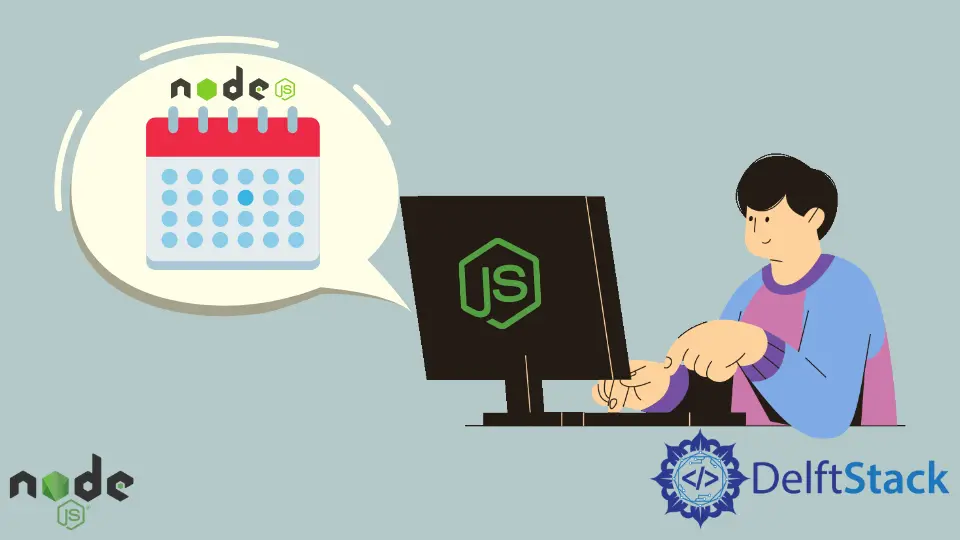
The JavaScript date
object lets us work with dates in the browser and NodeJS. This object represents the number of milliseconds since the ECMAScript
epoch, i.e., since 1 January 1970 UTC.
Use the new Date()
Object to Create a New Date Object in NodeJS
The new Date()
object creates a new object with the current time and date. However, we can also create an object with a certain time and date.
const d_t = new Date();
const d_t = new Date(milliseconds);
const d_t = new Date(dateString);
const d_t = new Date(year, month, day);
const d_t = new Date(year, month, day, hour, minute, second, millisecond);
Once we have created date
objects, we may desire specific details from some of the above objects.
Use the getter
Function to Get Specific Date Object in NodeJS
These functions are known as getter
functions, and they allow us to get specific details such as the year
, month
, day
, or hour
.
const d_t = new Date();
let year = d_t.getFullYear();
let month = d_t.getMonth();
let day = d_t.getDate();
let hour = d_t.getHours();
let minute = d_t.getMinutes();
console.log(year);
console.log(month);
console.log(day);
console.log(hour);
console.log(minute);
Output:
2022
1
7
10
5
The getFullYear()
method returns the year four-digit format. In contrast, getMonth
, getDate
, getHours
, and getMinutes
return the month, date, hours, and minutes.
the YYYY-MM-DD hh:mm:ss
Combination Using the get
Function in NodeJS
const d_t = new Date();
let year = d_t.getFullYear();
let month = ("0" + (d_t.getMonth() + 1)).slice(-2);
let day = ("0" + d_t.getDate()).slice(-2);
let hour = d_t.getHours();
let minute = d_t.getMinutes();
let seconds = d_t.getSeconds();
// prints date & time in YYYY-MM-DD HH:MM:SS format
console.log(year + "-" + month + "-" + day + " " + hour + ":" + minute + ":" + seconds);
Output:
2022-02-07 10:18:44
In NodeJS, a library is the best way of DateTime
handling. Libraries provide more functionality such as parsing, validating, manipulating, formatting, and date/time calculations.
Those libraries include Day.js
, Luxon
, SugarJs
, and MomentJS
, which are no longer maintained.
Day.js
is perhaps one of the most used libraries with 37,781 GitHub stars.
Day.js
is a minimalist Node js library that parses, validates, manipulates, and displays dates and times for modern browsers. We can install Day.js
using the npm install command below.
npm install dayjs
Use the require()
Method to Print the Current Local Date-Time in NodeJS
We can now make our main file using the require()
method and print the current local date-time below.
const dayjs = require('dayjs');
let today = dayjs();
console.log(today.format());
Output:
2022-02-07T11:27:36-08:00
Format a Date Object Using the YYYY-MM-DD hh:mm:ss
Function
const dayjs = require('dayjs');
let today = dayjs();
console.log("ISO")
console.log(today.format());
console.log("\nDate")
console.log(today.format("YYYY-MM-DD h:mm:ss"));
Output:
ISO
2022-02-07T11:33:13-08:00
Date
2022-02-07 11:33:13
Use the toString()
Method to Format Date Object Into a String in NodeJS
We can also format this date object into a string format using the built-in toString()
method, as shown below.
const dayjs = require('dayjs');
let today = dayjs();
console.log("ISO")
console.log(today.format());
console.log("\nDate")
console.log(today.format("YYYY-MM-DD h:mm:ss").toString());
Output:
ISO
2022-02-07T11:45:25-08:00
Date
2022-02-07 11:45:25
We can also format date
objects to other formats such as JSON. Access DateTime
and perform arithmetic.
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn