在 NodeJS 中格式化日期
-
在 NodeJS 中使用
new Date()
物件建立一個新的日期物件 -
在 NodeJS 中使用
getter
函式獲取特定的日期物件 -
在 NodeJS 中使用
get
函式的YYYY-MM-DD hh:mm:ss
組合 -
在 NodeJS 中使用
require()
方法列印當前本地日期時間 -
使用
YYYY-MM-DD hh:mm:ss
函式格式化日期物件 -
在 NodeJS 中使用
toString()
方法將日期物件格式化為字串
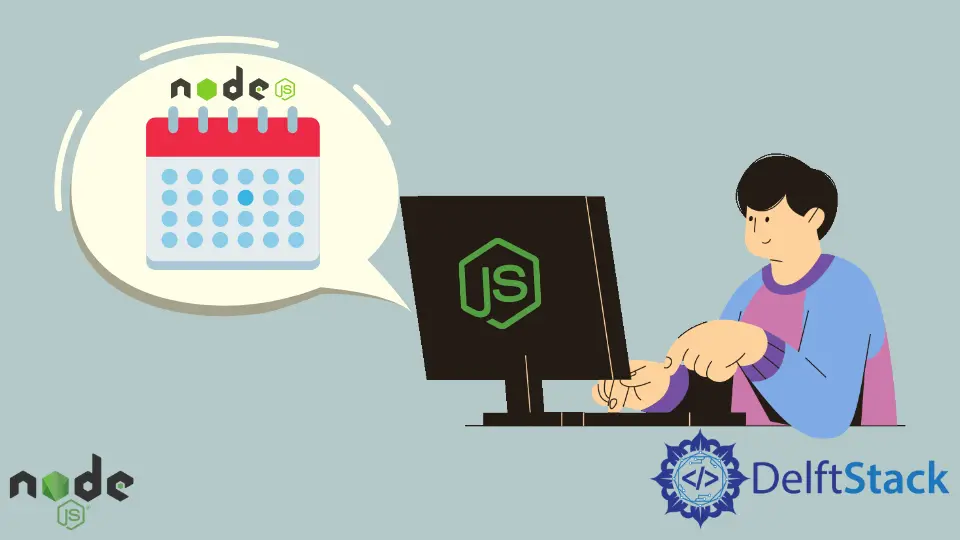
JavaScript date
物件讓我們可以在瀏覽器和 NodeJS 中處理日期。該物件表示自 ECMAScript
紀元以來的毫秒數,即自 1970 年 1 月 1 日 UTC 以來。
在 NodeJS 中使用 new Date()
物件建立一個新的日期物件
new Date()
物件建立一個具有當前時間和日期的新物件。但是,我們也可以建立具有特定時間和日期的物件。
const d_t = new Date();
const d_t = new Date(milliseconds);
const d_t = new Date(dateString);
const d_t = new Date(year, month, day);
const d_t = new Date(year, month, day, hour, minute, second, millisecond);
一旦我們建立了 date
物件,我們可能希望從上述某些物件中獲取特定的詳細資訊。
在 NodeJS 中使用 getter
函式獲取特定的日期物件
這些函式被稱為 getter
函式,它們允許我們獲取特定的詳細資訊,例如 year
、month
、day
或 hour
。
const d_t = new Date();
let year = d_t.getFullYear();
let month = d_t.getMonth();
let day = d_t.getDate();
let hour = d_t.getHours();
let minute = d_t.getMinutes();
console.log(year);
console.log(month);
console.log(day);
console.log(hour);
console.log(minute);
輸出:
2022
1
7
10
5
getFullYear()
方法返回四位數字格式的年份。相反,getMonth
、getDate
、getHours
和 getMinutes
返回月份、日期、小時和分鐘。
在 NodeJS 中使用 get
函式的 YYYY-MM-DD hh:mm:ss
組合
const d_t = new Date();
let year = d_t.getFullYear();
let month = ("0" + (d_t.getMonth() + 1)).slice(-2);
let day = ("0" + d_t.getDate()).slice(-2);
let hour = d_t.getHours();
let minute = d_t.getMinutes();
let seconds = d_t.getSeconds();
// prints date & time in YYYY-MM-DD HH:MM:SS format
console.log(year + "-" + month + "-" + day + " " + hour + ":" + minute + ":" + seconds);
輸出:
2022-02-07 10:18:44
在 NodeJS 中,庫是處理 DateTime
的最佳方式。庫提供更多功能,例如解析、驗證、操作、格式化和日期/時間計算。
這些庫包括不再維護的 Day.js
、Luxon
、SugarJs
和 MomentJS
。
Day.js
可能是最常用的庫之一,擁有 37,781 個 GitHub 星。
Day.js
是一個極簡的 Node js 庫,它為現代瀏覽器解析、驗證、操作和顯示日期和時間。我們可以使用下面的 npm install 命令安裝 Day.js
。
npm install dayjs
在 NodeJS 中使用 require()
方法列印當前本地日期時間
我們現在可以使用 require()
方法制作我們的主檔案並在下面列印當前的本地日期時間。
const dayjs = require('dayjs');
let today = dayjs();
console.log(today.format());
輸出:
2022-02-07T11:27:36-08:00
使用 YYYY-MM-DD hh:mm:ss
函式格式化日期物件
const dayjs = require('dayjs');
let today = dayjs();
console.log("ISO")
console.log(today.format());
console.log("\nDate")
console.log(today.format("YYYY-MM-DD h:mm:ss"));
輸出:
ISO
2022-02-07T11:33:13-08:00
Date
2022-02-07 11:33:13
在 NodeJS 中使用 toString()
方法將日期物件格式化為字串
我們還可以使用內建的 toString()
方法將此日期物件格式化為字串格式,如下所示。
const dayjs = require('dayjs');
let today = dayjs();
console.log("ISO")
console.log(today.format());
console.log("\nDate")
console.log(today.format("YYYY-MM-DD h:mm:ss").toString());
輸出:
ISO
2022-02-07T11:45:25-08:00
Date
2022-02-07 11:45:25
我們還可以將日期
物件格式化為其他格式,例如 JSON。訪問 DateTime
並執行算術運算。
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn