Callback Functions in Node.js
- Use Callback Function to Read a File Synchronously (Blocking Code) in Node.js
- Use Callback Function to Read a File Asynchronously (Non-Blocking Code) in Node.js
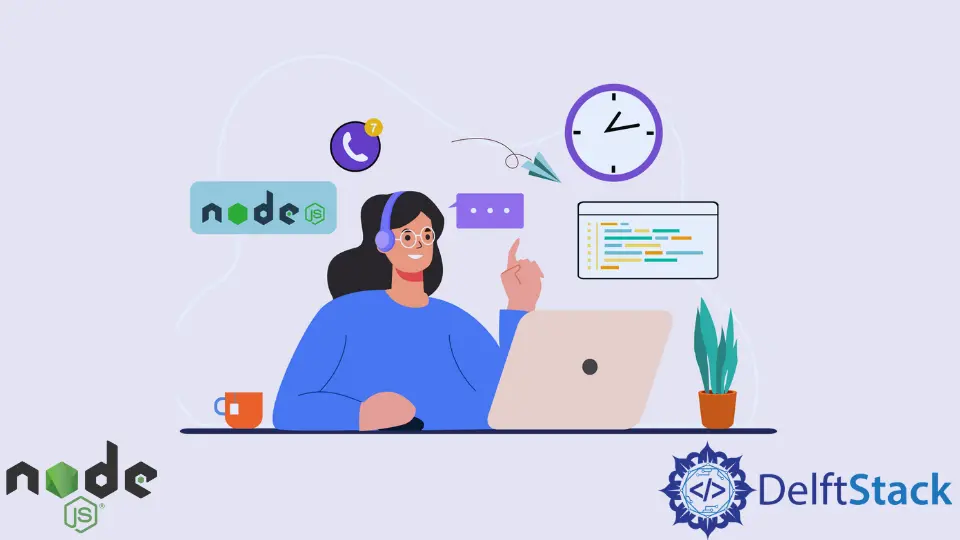
A callback function is a function that is executed after a set of tasks have been executed, averting the blockage of the whole program. Callback functions are an important aspect of writing asynchronous and non-blocking programs in Node.js.
In JavaScript, callback functions are formally referred to as functions that pass as arguments to other functions. Since JavaScript functions are executed in the order they are called, using callbacks allows us to control better how functions execute.
Suppose we have two closely related functions so that the result from one function is used as a parameter in the other function. In such a scenario, the initial function will always block the second function until the result from the initial function is ready.
However, using callback functions, we can ensure that the initial function will always run the last function as a callback function after it’s done executing its statements, as shown below.
function print(callback) {
callback();
}
Use Callback Function to Read a File Synchronously (Blocking Code) in Node.js
Node.js callbacks form the basis of executing asynchronous operations, making Node.js suitable for developing I/O intensive applications. Such applications often involve fetching huge amounts of data from the server.
Loading large data is a long process, and therefore sitting and waiting for the program to load the data fully before executing the rest is not a good practice.
In the example below, we use the fs
module in Node.js to read data synchronously before executing the rest of the code.
// Write JavaScript code
var fs = require('fs');
var filedata = fs.readFileSync('usersinfo.txt');
console.log(filedata.toString());
console.log('Other parts of the program that execute afterwards');
console.log('End of program execution');
Sample output:
First name: john
Last name: Doe
Occupation: Microservices Developer
Other parts of the program that execute afterward
End of program execution
Use Callback Function to Read a File Asynchronously (Non-Blocking Code) in Node.js
Synchronously reading a file blocks the execution of other programs’ parts until data is fully read. In cases where the data is huge, we may have to wait a little longer.
However, as shown below, we can execute a function that reads that data asynchronously in the background using callback functions while executing other parts of the program.
var fs = require('fs');
fs.readFile('usersinfo.txt', function(err, data) {
if (err) return console.error(err);
console.log(data.toString());
});
console.log('Other parts of the program that execute afterward');
Sample output:
Other parts of the program that execute afterward
firstname: john
lastname: Doe
Occupation: Microservices Developer
Using the callback function concept in the programs above, we can execute other parts of the programs as the data is loaded in the background.
Furthermore, in the above code, we have also followed the standard convention of returning errors as the first argument in the callback function. The first object, if non-null, should be considered as the error object; otherwise, it should remain null.
It provides an easier way to know that an error has occurred. Traditionally since the call back function is meant to be executed after the main function is done performing other operations, it is a common practice to have it as the second parameter.
Virtually any asynchronous function using the callback function is expected to follow the syntax below in Node.js.
function asyncOperation(param1, param2, callback) {
// operations to be executed
if (err) {
console.log('An error has occured!');
return callback(new Error('An error'));
}
// ... more work ...
callback(null, param1, param2);
}
asyncOperation(parameters, function(err, returnValues) {
// This code gets run after the async operation
});
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn