Node.js 中的回调函数
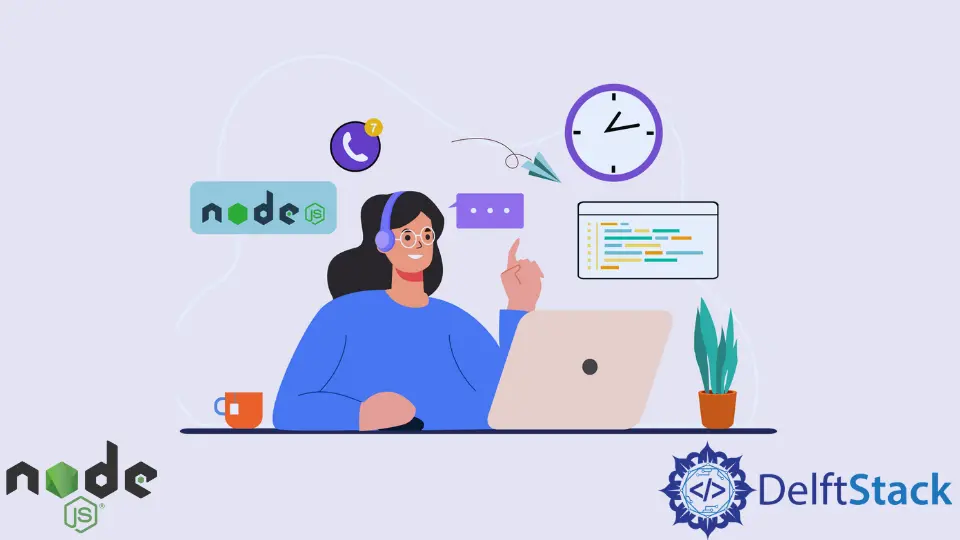
回调函数是在一组任务执行完毕后执行的函数,避免了整个程序的阻塞。回调函数是在 Node.js 中编写异步和非阻塞程序的一个重要方面。
在 JavaScript 中,回调函数被正式称为作为参数传递给其他函数的函数。由于 JavaScript 函数是按照调用顺序执行的,因此使用回调可以让我们更好地控制函数的执行方式。
假设我们有两个密切相关的函数,因此一个函数的结果被用作另一个函数的参数。在这种情况下,初始函数将始终阻塞第二个函数,直到初始函数的结果准备好。
但是,使用回调函数,我们可以确保初始函数在执行完其语句后始终将最后一个函数作为回调函数运行,如下所示。
function print(callback) {
callback();
}
在 Node.js 中使用回调函数同步读取文件(阻塞代码)
Node.js 回调构成了执行异步操作的基础,使 Node.js 适合开发 I/O 密集型应用程序。此类应用程序通常涉及从服务器获取大量数据。
加载大数据是一个漫长的过程,因此在执行其余部分之前坐等程序完全加载数据并不是一个好习惯。
在下面的示例中,我们使用 Node.js 中的 fs
模块在执行其余代码之前同步读取数据。
// Write JavaScript code
var fs = require('fs');
var filedata = fs.readFileSync('usersinfo.txt');
console.log(filedata.toString());
console.log('Other parts of the program that execute afterwards');
console.log('End of program execution');
样本输出:
First name: john
Last name: Doe
Occupation: Microservices Developer
Other parts of the program that execute afterward
End of program execution
在 Node.js 中使用回调函数异步读取文件(非阻塞代码)
同步读取文件会阻止其他程序部分的执行,直到数据被完全读取。在数据量很大的情况下,我们可能需要等待更长的时间。
但是,如下所示,我们可以执行一个函数,在执行程序的其他部分时使用回调函数在后台异步读取该数据。
var fs = require('fs');
fs.readFile('usersinfo.txt', function(err, data) {
if (err) return console.error(err);
console.log(data.toString());
});
console.log('Other parts of the program that execute afterward');
样本输出:
Other parts of the program that execute afterward
firstname: john
lastname: Doe
Occupation: Microservices Developer
使用上述程序中的回调函数概念,我们可以在后台加载数据时执行程序的其他部分。
此外,在上面的代码中,我们还遵循了将错误作为回调函数的第一个参数返回的标准约定。第一个对象,如果非空,应该被认为是错误对象;否则,它应该保持为空。
它提供了一种更简单的方法来了解发生了错误。传统上,由于回调函数是在主函数执行完其他操作后执行的,因此将其作为第二个参数是一种常见的做法。
实际上,任何使用回调函数的异步函数都应该遵循 Node.js 中的以下语法。
function asyncOperation(param1, param2, callback) {
// operations to be executed
if (err) {
console.log('An error has occured!');
return callback(new Error('An error'));
}
// ... more work ...
callback(null, param1, param2);
}
asyncOperation(parameters, function(err, returnValues) {
// This code gets run after the async operation
});
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn