How to Print to Console in MySQL
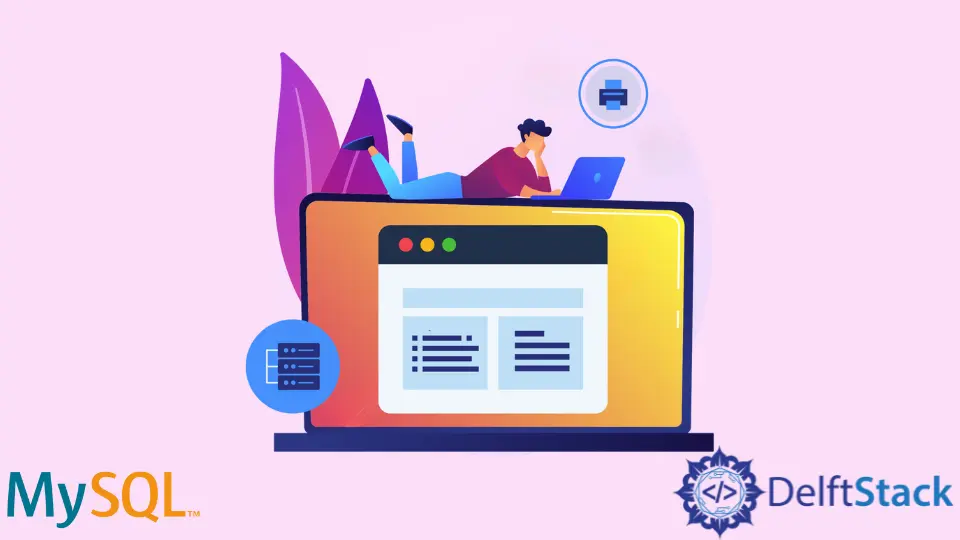
This article will discuss how to print a message or data to the console in MySQL.
MySQL Print to Console
When working with MySQL databases, we usually need to print some data or a message to the console. MySQL can use the SELECT
command to achieve such tasks.
MySQL’s SELECT
statement is mainly used to retrieve data from a database. We can also use it to print something in the console.
The return value or data will be presented in a table called result-set, and usually, we can see a column header and a single row when we print something using the SELECT
statement.
Print a Message
Let’s try this with an example.
SELECT 'Hello World' AS Message;
In the above statement, the message we want to print is "Hello world"
, and we have published it using the SELECT
method. Also, as below, we have added Message
as the column header so that the output will be more precise.
Output:
+-------------+
| Message |
+-------------+
| Hello World |
+-------------+
As in above, we can see the result-set table with our message.
Print a Variable
We can print a variable with a value to the console using the SELECT
statement. To do this, firstly, we should create a procedure, then declare a variable with the data type and assign a value using the SET
statement.
When declaring a variable, we should do it inside the BEGIN
and END
blocks; otherwise, some syntax errors can occur. Then we can print a message using the SELECT
command.
Refer to the below example.
DELIMITER //
-- Creating the procedure
CREATE procedure myProcedure()
BEGIN
-- Declaring the variable and assigning the value
declare myvar VARCHAR(20);
SET myvar = 'Hello world';
-- Printing the value to the console
SELECT concat(myvar) AS Variable;
END //
DELIMITER ;
-- Calling the procedure
CALL myProcedure()
The process mentioned above is in this code, and we have assigned Hello world
for the myvar
variable. After running the code, we can get a result like this.
Output:
+-------------+
| Variable |
+-------------+
| Hello World |
+-------------+
Also, this method can print concatenated messages to the console. For example, If we want to print "Sam is 10 years old"
, and 10
is the variable, we can print it using the concatenation.
Let’s convert the above scenario into a code as below.
DELIMITER //
-- Creating the procedure
CREATE procedure myProcedure()
BEGIN
-- Declaring the variable and assigning the value
declare myvar INT DEfAULT 0;
SET myvar = 10;
-- Printing the value to the console
SELECT concat('Sam is ',myvar,' years old') AS Variable;
END //
DELIMITER ;
-- Calling the procedure
CALL myProcedure()
In the above code chunk, we have declared a variable called myvar
inside the procedure: myProcedure
. The myvar
variable is an integer since it stores age, and age is a number.
Then we set 10
as the value of the myvar
variable and concatenated a message with the variable within the SELECT
statement. After that, the procedure is called, and when we run the code, we’ll get the following result.
Output:
+--------------------+
| Variable |
+--------------------+
| Sam is 10 years old|
+--------------------+
Conclusion
In this article, we learned about an essential feature that MySQL gives us: print to console and how to perform it within the technology. As understood, we can use the SELECT
statement to achieve the task, and we looked at several use cases where we need to print messages to the console through some examples.
There are more use cases and different methods to do this, but the techniques discussed in this article are the fundamental and easiest ways to perform the task.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.