MySQL COUNT IF Method
-
Understanding the
COUNT IF
Method in MySQL -
Use the
COUNT IF
Method in MySQL - Additional Tips and Techniques
- Conclusion
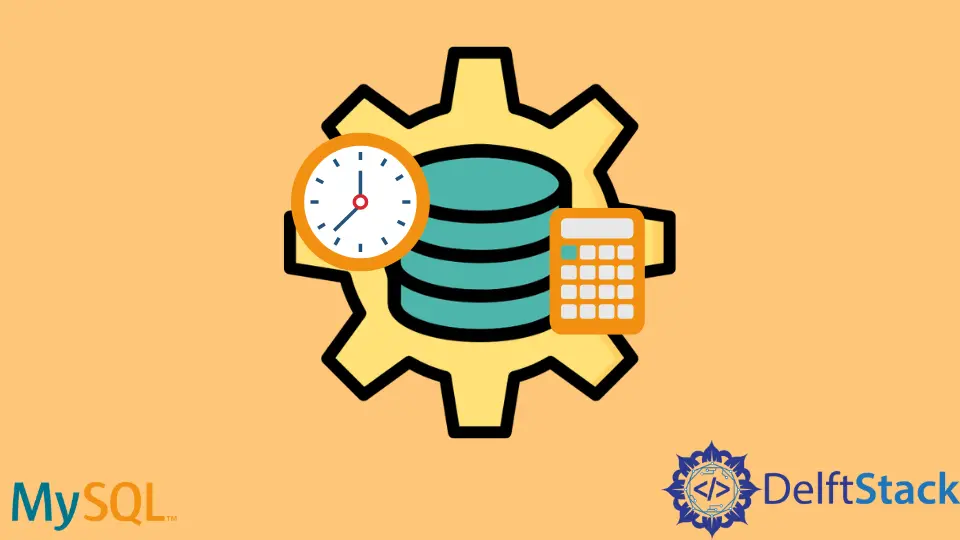
The COUNT IF
method in MySQL is a powerful tool for retrieving specific information from a database. It allows you to count the number of rows that meet specific criteria, providing valuable insights into your data.
In this tutorial, we will explore the syntax, usage, and examples of the COUNT IF
method in MySQL.
Understanding the COUNT IF
Method in MySQL
The COUNT IF
method is not a standalone function in MySQL. Instead, it is a combination of two functions: COUNT()
and IF()
.
The COUNT()
function is used to count the number of rows in a table, while the IF()
function is used to apply a condition. When used together, they enable you to count the number of rows that satisfy a particular condition.
The syntax of the COUNT IF
method in MySQL is as follows:
SELECT COUNT(IF(condition, 1, NULL)) AS count_result
FROM table_name;
Here, condition
represents the specific condition that you want to evaluate. If the condition is met, IF()
returns 1
; otherwise, it returns NULL
.
The COUNT()
function then counts the number of non-null values, effectively giving you the count of rows that meet the specified condition.
Basic Example
Let’s illustrate the usage of the COUNT IF
method with a practical example. Suppose we have a table named students
with columns student_id
, name
, and grade
that have the following values:
-- Create the students table
CREATE TABLE students (
student_id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
grade INT
);
-- Insert sample data
INSERT INTO students (name, grade) VALUES
('John Doe', 85),
('Jane Smith', 75),
('Michael Johnson', 92),
('Sarah Williams', 78),
('David Brown', 88);
We want to count the number of students who scored above 80
.
SELECT COUNT(IF(grade > 80, 1, NULL)) AS high_scorers
FROM students;
In this example, if a student’s grade is above 80
, the IF()
function returns 1
; otherwise, it returns NULL
. The COUNT()
function then counts the non-null values, giving us the number of high scorers.
Output:
high_scorers
3
Use the COUNT IF
Method in MySQL
The COUNT()
method in MySQL gives the total number of rows in the table as the output. But in this example, we are interested in understanding how we can count information based on a particular IF
condition from our data.
The IF
command gives us the total number of distinct non-null values only if the values satisfy the expression or the condition mentioned in the IF
query fragment.
Let us understand how this method works. Before starting, we create a dummy dataset to work on by creating a table named student_details
and inserting values into it.
-- Create the table student_details
CREATE TABLE student_details(
stu_id int,
stu_firstName varchar(255) DEFAULT NULL,
stu_lastName varchar(255) DEFAULT NULL,
primary key(stu_id)
);
-- insert rows to the table student_details
INSERT INTO student_details(stu_id,stu_firstName,stu_lastName)
VALUES(1,"Preet","Sanghavi"),
(2,"Rich","John"),
(3,"Veron","Brow"),
(4,"Geo","Jos"),
(5,"Hash","Shah"),
(6,"Sachin","Parker"),
(7,"David","Miller");
In our student_details
table, let us count the total number of distinct stu_id
with their stu_firstName
ending in "reet"
. This task can be achieved using the following query.
SELECT DISTINCT
COUNT(DISTINCT IF(stu_firstName like '%reet',
stu_id,
NULL)) AS count_student_ids
FROM student_details;
This code counts distinct numbers of stu_id
from the student_details
table given the condition that the stu_firstName
should end in "reet"
in the IF
clause. We use the alias count_student_ids
with the AS
keyword in MySQL.
Output:
count_student_ids
1
COUNT IF
technique is the SUM CASE
technique, which can help us achieve similar results.Therefore, with the help of the COUNT IF
technique, we can efficiently count the total number of occurrences of an entity based on a particular condition from a table in MySQL.
Additional Tips and Techniques
Using Multiple Conditions
You can use multiple conditions within the IF()
function to perform more complex evaluations. For example, if you want to count students who scored above 80
and are in grade 10
:
SELECT COUNT(IF(grade > 80 AND grade_level = 10, 1, NULL)) AS high_scorers_10th_grade
FROM students;
This query is asking MySQL to count the number of students who have a grade above 80
and are in the 10th grade. The result of this count will be labeled as high_scorers_10th_grade
in the output.
This SQL statement provides a specific count of high-achieving students who are currently in the 10th grade. It demonstrates how to use conditional logic within the IF()
function to filter and count data based on multiple criteria.
Applying Aggregate Functions
The result of the COUNT IF
method can be further utilized with other aggregate functions. For instance, if you want to find the average grade of high scorers:
SELECT AVG(grade) AS average_grade
FROM students
WHERE grade > 80;
This query retrieves the average grade of students from the students
table, considering only those whose grades exceed 80
.
This can be particularly useful for identifying high-performing students or for generating reports on specific segments of the student population. The result will be a single value representing the average grade of this selected group.
Utilizing Aliases
You can use aliases to provide meaningful names to your result columns. This makes your queries more readable and helps in understanding the output.
SELECT COUNT(IF(grade > 80, 1, NULL)) AS high_scorers,
COUNT(IF(grade <= 80, 1, NULL)) AS low_scorers
FROM students;
This query provides a concise summary of the distribution of student performance. It reports the number of high scorers and low scorers, providing valuable insights into the student body’s academic achievements.
The result will be a row with two values: the count of high scorers and the count of low scorers.
Conclusion
The COUNT IF
method in MySQL is a versatile tool for extracting specific information from your database. By combining the COUNT()
and IF()
functions, you can perform conditional counting, enabling you to gain valuable insights into your data.
Understanding the syntax and usage of the COUNT IF
method opens up a world of possibilities for data analysis and reporting. Whether you’re working with large datasets or need to generate summary reports, this method will be a valuable addition to your SQL toolkit.