How to Sort MySQL Data in Alphabetical Order
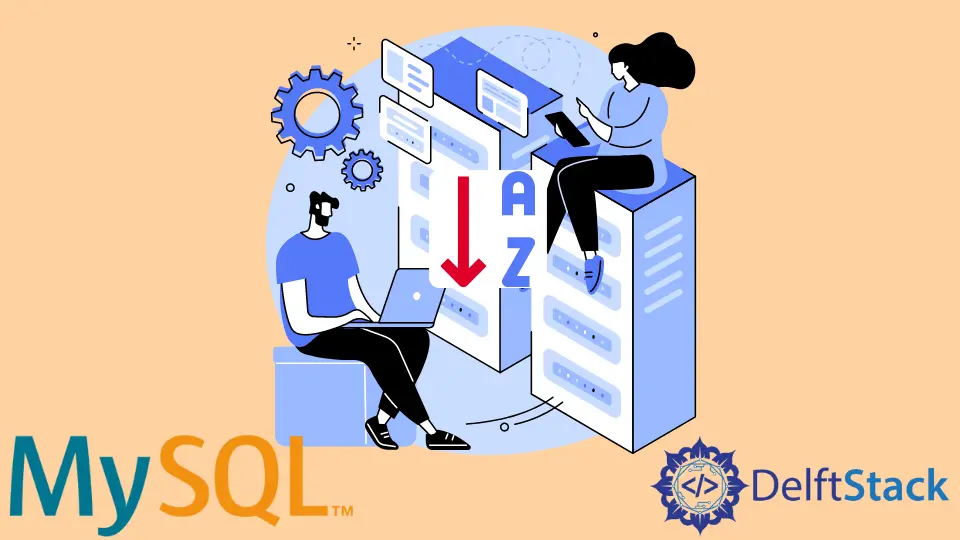
In this tutorial, we aim to explore how to sort data in alphabetical order in a MySQL database.
Sorting is ordering elements or values in an array or a column based on a particular criterion. In this tutorial, we will set the criterion in alphabetical order and fetch the names of a few students starting from A to Z.
Let us try to understand how to sort this data in alphabetical order.
Create a Table in MySQL
Before we begin, we will create a dummy dataset to work with. Here we will create a table, student_details
, along with a few rows.
-- create the table student_details
CREATE TABLE student_details(
stu_id int,
stu_firstName varchar(255) DEFAULT NULL,
stu_lastName varchar(255) DEFAULT NULL,
primary key(stu_id)
);
-- insert rows to the table student_details
INSERT INTO student_details(stu_id,stu_firstName,stu_lastName)
VALUES(1,"Preet","Sanghavi"),
(2,"Rich","John"),
(3,"Veron","Brow"),
(4,"Geo","Jos"),
(5,"Hash","Shah"),
(6,"Sachin","Parker"),
(7,"David","Miller");
The above code would give the following output:
stu_id stu_firstName stu_lastName
1 Preet Sanghavi
2 Rich John
3 Veron Brow
4 Geo Jos
5 Hash Shah
6 Sachin Parker
7 David Miller
Sort Data in Alphabetical Order in a MySQL Database
Now, we have understood how to create a table and view it. Let us try to understand exactly how to sort data in MySQL.
We mainly use the ORDER
keyword to sort data in alphabetical or numerical order. The syntax for this keyword can be better understood with the help of the following query:
select something from table_name ORDER BY something_else;
Let us explore this statement with our student_details
table and sort the data in the stu_firstName
column with the help of the ORDER
keyword. This can be done with the help of the following query:
select stu_firstName from student_details ORDER BY stu_firstName;
The output of the query mentioned above can be illustrated as follows:
stu_firstName
David
Geo
Hash
Preet
Rich
Sachin
Veron
Thus, we have successfully ordered names in ascending order from A to Z.
It is important to note that we can also sort in the reverse order with the help of the DESC
keyword. This can be understood with the help of the following query:
select stu_firstName from student_details ORDER BY stu_firstName DESC;
The output of the query above is the same as before and can be illustrated as follows:
stu_firstName
Veron
Sachin
Rich
Preet
Hash
Geo
David
As we can see, we have now reversed the ordering, starting with Z to A. Therefore, we have successfully learned different techniques to sort data in a MySQL database in alphabetical order.
Related Article - MySQL Query
- How to Enable Slow Query Log in MySQL
- How to Calculate Percentage in MySQL
- Where vs Having in MySQL
- Nested Select Statements in MySQL for Enhanced Query
- Tiny Integer in MySQL