How to Connect MongoDB With PowerShell
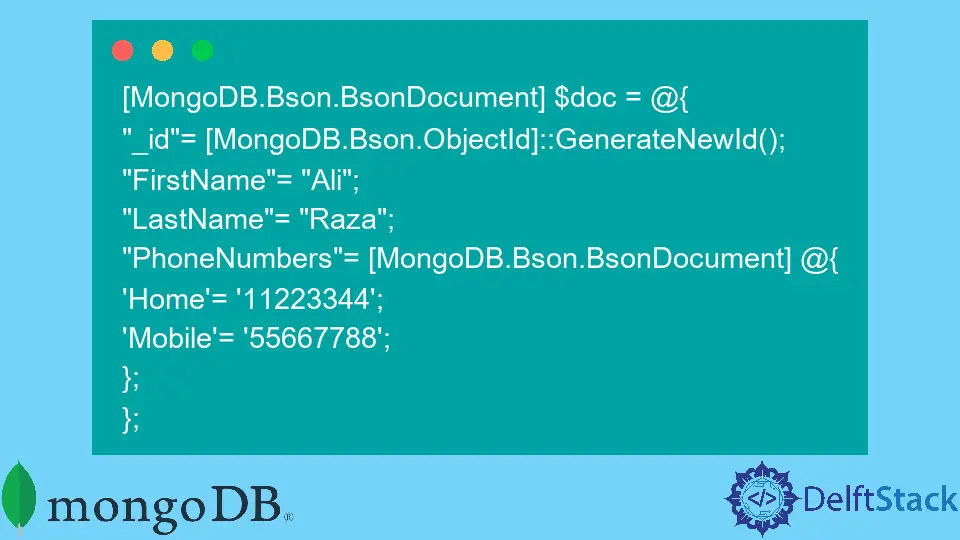
MongoDB introduces a NoSQL solution for data storage and management, consisting of documents represented in JSON style. This document-oriented language is suitable to be run with PowerShell, which offers a command-line shell solution on Microsoft Windows.
This article will look at different ways to connect MongoDB with PowerShell and use it for MongoDB tasks.
Connect MongoDB With PowerShell Using Mdbc
Mdbc
is a PowerShell module used for making MongoDB operations PowerShell-friendly to easily run them on PowerShell. Mdbc
is based on the official MongoDB C# driver.
To learn about Mdbc
, you first need to be familiar with the concept of cmdlets. Cmdlets are lightweight commands that run on PowerShell.
There are also cmdlets for MongoDB, which are standard PowerShell commands that make it easy to perform data cleansing, normalization, backup, and other MongoDB operations.
To run MongoDB on PowerShell using Mdbc
, you need to follow a sequence of simple steps explained below.
Get and Install Mdbc
Mdbc
for PowerShell Core and version 5.1, .NET 4.7.2 is published as the PSGallery
module Mdbc
. To install this module, you have to run the following command:
Install-Module Mdbc
For PowerShell v3-v5.1, .NET 4.7.2, Mdbc
is published as the NuGet
package Mdbc
. You have to download it directly or use the NuGet
tools.
If you are downloading it directly, you have to save it as a .zip
file, unzip it, and use the package subdirectory tools/Mdbc
.
Now, copy the Mdbc
directory to any PowerShell module directories. To get the PowerShell module path run this command $env:PSModulePath
.
Note: The version of PowerShell you are using must be accurate in the range for this to work. You can check the version by running
$PSVersionTable
.
Import the Mdbc
Module
Now, run the following command in PowerShell to import the Mdbc
module.
Import-Module Mdbc
Take a Look at the Help
You can run the following commands to look at help tutorials regarding Mdbc
.
help about_Mdbc
help Connect-Mdbc -Full
Get-Command -Module Mdbc
Make Sure mongod
Is Running
To ensure that mongod
is running, try running some MongoDB commands. For example, run the following to connect to a new collection test
.
Connect-Mdbc . test test -NewCollection
Now, add two documents to the collection as test data.
@{_id = 1; value = 42}, @{_id = 2; value = 3.14} | Add-MdbcData
Using the following command, retrieve the documents as PowerShell objects and display them as a table.
Get-MdbcData -As PS | Format-Table -AutoSize | Out-String
To retrieve a specific document by its id, use the following command as a query expression.
$data = Get-MdbcData (New-MdbcQuery _id -EQ 1)
$data
To update the document’s value, use the following:
$data._id | Update-MdbcData (New-MdbcUpdate -Set @{value = 100})
To check if the value has been updated successfully, use the following query:
$doc = Get-MdbcData @{_id = 1}
Try to remove the document by running the following:
$doc | Remove-MdbcData
To confirm that the document has been removed successfully, run the following command to count the number of documents. The expected output is 1 because there is only one document remaining.
Get-MdbcData -Count
Use MongoDB With PowerShell Directly
Previously, we discussed connecting MongoDB with PowerShell using Mdbc
. Let us see how we can do this without it.
You need to download the latest MongoDB engine and the C# driver to run it with PowerShell. The next step will be to create two folders in your computer’s C:
drive, C:\data\db
.
Now, you can start the mongod.exe
file in your MongoDB installation’s bin
folder. When you run mongod
, it will monopolize the PowerShell prompt.
Note: You can also run MongoDB as a service by installing it using
.\mongod.exe --install --serviceName "Mongod" --serviceDisplayName "Mongo service deamon" --serviceDescription "MongoDB service" --logpath c:\data\Mongo.log
For more information, you can type the following command in PowerShell:
mongod.exe –help
Now, you can open your firewall for port 27017
to allow connections to the MongoDB database. The MongoDB engine will start, and you can now open PowerShell, load the C# drivers and connect to the MongoDB database.
The code is as follows:
$mongoDriverPath = "c:\Program Files (x86)\MongoDB\CSharpDriver 1.7"
Add-Type -Path "$($mongoDriverPath)\MongoDB.Bson.dll"
Add-Type -Path "$($mongoDriverPath)\MongoDB.Driver.dll"
You must remember that this code snippet will have to be modified according to the path on your computer.
Let us test the connection by creating a database and collection using the following commands:
$db = [MongoDB.Driver.MongoDatabase]::Create('mongodb://localhost/profiles');
$coll = $db['example1'];
MongoDB also allows importing data using a CSV, TSV, or JSON file. To find more information about this, you can run the following command (adjusting it according to your path).
PS C:\Program Files\MongoDB\Server\3.4\bin> .\mongoimport.exe --help
Let us now see how we can create and insert a BSON document into our database.
[MongoDB.Bson.BsonDocument] $doc = @{
"_id"= [MongoDB.Bson.ObjectId]::GenerateNewId();
"FirstName"= "Ali";
"LastName"= "Raza";
"PhoneNumbers"= [MongoDB.Bson.BsonDocument] @{
'Home'= '11223344';
'Mobile'= '55667788';
};
};
To insert this document, use the following:
C:\> $coll.Insert( $doc)
The following command can be run to run an update query:
$query = [MongoDB.Driver.Builders.Query]::Matches( 'FirstName', "Ali")
$update = [MongoDB.Driver.Builders.Update]::Set("Email", 'ali123@abc.org'))
$coll.Update( $query , $update )
Or, if you want to retrieve the data in a read operation, use the Find
command like this:
$query = [MongoDB.Driver.Builders.Query]::Matches( 'FirstName', "Ali")
$coll.Find( $query )
Conclusion
MongoDB provides an efficient document-oriented NoSQL solution to perform database operations quickly and easily. MongoDB can be run on PowerShell using various methods, two of which we have explained in this article.
We hope you were able to grasp the concepts we delivered!
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub