How to Show Indexes in MongoDB
-
Use
getIndexes()
to Show Indexes From a Specific Collection in MongoDB -
Use
forEach()
to Show Indexes From All Collections in a Database in MongoDB - Show Indexes From All Collections of All Databases in MongoDB
-
Show the
text
Indexes Only From All Collections of All Databases in MongoDB
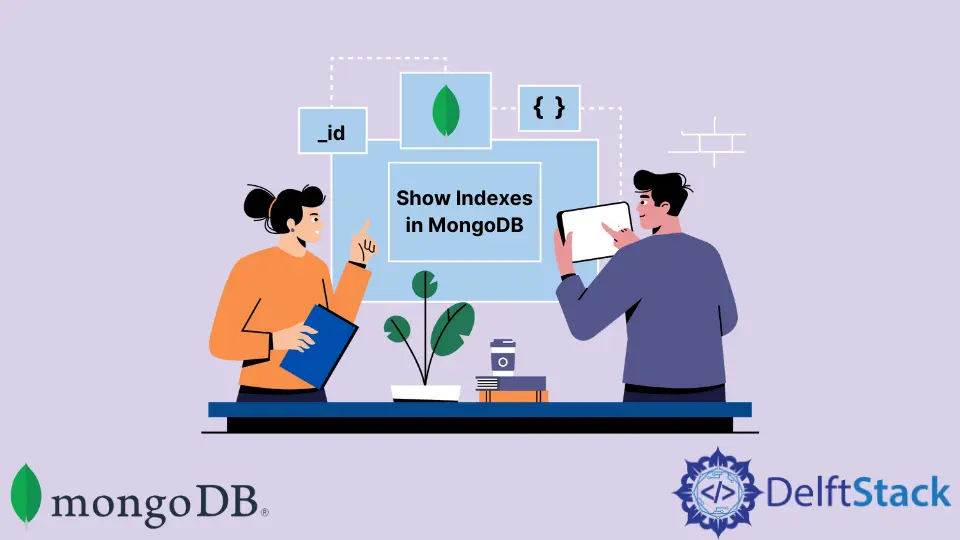
Today, we will learn how to show indexes from one collection, all collections of a database, and all collections of all databases. We will also see how to get the specific type of indexes from all collections of all databases.
Depending on the project requirements, we can use one of the following ways to show indexes in MongoDB. In this tutorial, we will go from specific to general solutions.
Use getIndexes()
to Show Indexes From a Specific Collection in MongoDB
Example Code:
// MongoDB Version 5.0
> db.Client.getIndexes();
OUTPUT:
[
{
"v" : 2,
"key" : {
"_id" : 1
},
"name" : "_id_"
},
{
"v" : 2,
"key" : {
"shopPosId" : 1
},
"name" : "shopPosId_1"
}
]
In the above output, we can see two indexes where the index for the shopPosId
field is user-created while _id
is the default index. If you have not created any index for a specific collection, you will only see the _id
as an index.
To get instant mongo shell assistance, we can also use any of the commands given below.
// MongoDB Version 5.0
> help;
> db.help();
> db.test.help();
We can also use the stats().indexSizes
to show indexes with respective sizes from a particular collection.
Example Code:
// MongoDB Version 5.0
> db.Client.stats().indexSizes
OUTPUT:
{ "_id_" : 36864, "shopPosId_1" : 20480 }
Use forEach()
to Show Indexes From All Collections in a Database in MongoDB
Example Code:
// MongoDB Version 5.0
> db.getCollectionNames().forEach(function(collection) {
all_indexes = db.getCollection(collection).getIndexes();
print("All Indexes for the " + collection + " collection:");
printjson(all_indexes);
});
OUTPUT:
All Indexes for the Client collection:
[
{
"v" : 2,
"key" : {
"_id" : 1
},
"name" : "_id_"
},
{
"v" : 2,
"key" : {
"shopPosId" : 1
},
"name" : "shopPosId_1"
}
]
All Indexes for the Shop collection:
[ { "v" : 2, "key" : { "_id" : 1 }, "name" : "_id_" } ]
All Indexes for the collection1 collection:
[ { "v" : 2, "key" : { "_id" : 1 }, "name" : "_id_" } ]
All Indexes for the collection2 collection:
[ { "v" : 2, "key" : { "_id" : 1 }, "name" : "_id_" } ]
All Indexes for the employee collection:
[
{
"v" : 2,
"key" : {
"_id" : 1
},
"name" : "_id_"
},
{
"v" : 2,
"key" : {
"_fts" : "text",
"_ftsx" : 1
},
"name" : "emp_code_text",
"weights" : {
"emp_code" : 1
},
"default_language" : "english",
"language_override" : "language",
"textIndexVersion" : 3
}
]
All Indexes for the printjson collection:
[ { "v" : 2, "key" : { "_id" : 1 }, "name" : "_id_" } ]
All Indexes for the student_courses collection:
[ { "v" : 2, "key" : { "_id" : 1 }, "name" : "_id_" } ]
This example code shows all the indexes from all collections in a current database. In this code snippet, the getCollectionNames()
method retrieves all the collection names from the selected database that are passed to an anonymous function one at a time using forEach()
.
Inside the forEach()
, we get all the indexes for the specified collection and save them into the all_indexes
object. Finally, we use the printjson()
to print the all_indexes
object on the console (which is the mongo shell in our case).
Show Indexes From All Collections of All Databases in MongoDB
Example Code:
// MongoDB Version 5.0
> db.adminCommand("listDatabases").databases.forEach(function(database){
let mdb = db.getSiblingDB(database.name);
mdb.getCollectionInfos({ type: "collection" }).forEach(function(collection){
let currentCollection = mdb.getCollection(collection.name);
let all_indexes = currentCollection.getIndexes();
print("All indexes on the " + database.name + "." + collection.name + ":");
printjson(all_indexes)
});
});
OUTPUT:
All indexes on the admin.system.version:
[ { "v" : 2, "key" : { "_id" : 1 }, "name" : "_id_" } ]
All indexes on the config.system.sessions:
[
{
"v" : 2,
"key" : {
"_id" : 1
},
"name" : "_id_"
},
{
"v" : 2,
"key" : {
"lastUse" : 1
},
"name" : "lsidTTLIndex",
"expireAfterSeconds" : 1800
}
]
All indexes on the local.startup_log:
[ { "v" : 2, "key" : { "_id" : 1 }, "name" : "_id_" } ]
All indexes on the test.Client:
[
{
"v" : 2,
"key" : {
"_id" : 1
},
"name" : "_id_"
},
{
"v" : 2,
"key" : {
"shopPosId" : 1
},
"name" : "shopPosId_1"
}
]
All indexes on the test.Shop:
[ { "v" : 2, "key" : { "_id" : 1 }, "name" : "_id_" } ]
All indexes on the test.collection1:
[ { "v" : 2, "key" : { "_id" : 1 }, "name" : "_id_" } ]
All indexes on the test.collection2:
[ { "v" : 2, "key" : { "_id" : 1 }, "name" : "_id_" } ]
All indexes on the test.employee:
[
{
"v" : 2,
"key" : {
"_id" : 1
},
"name" : "_id_"
},
{
"v" : 2,
"key" : {
"_fts" : "text",
"_ftsx" : 1
},
"name" : "emp_code_text",
"weights" : {
"emp_code" : 1
},
"default_language" : "english",
"language_override" : "language",
"textIndexVersion" : 3
}
]
All indexes on the test.printjson:
[ { "v" : 2, "key" : { "_id" : 1 }, "name" : "_id_" } ]
All indexes on the test.student_courses:
[ { "v" : 2, "key" : { "_id" : 1 }, "name" : "_id_" } ]
The example code given above will iterate over all of the databases. In each database, it iterates over all the collections.
And in every collection, it gets all the indexes and prints them with their respective database and collection names.
Following is a brief explanation of all the methods we used for the code snippet above.
adminCommand()
- It provides the helper to execute the given database commands against theadmin
database ignoring the database context in which it executes.getSiblingDB()
- We use this method to return the database object without modifying thedb
variable in the shell environment.getCollectionInfos()
- It is used to return the array of documents containing the view or collection information, for instance, options and name for the selected database (current database). Remember that the results we get are based on the user’s privileges.getCollection()
- It returns a collection object for this code although it can return a view object as well. It is functionally equivalent to thedb.collection_name
syntax but we are using this method to get access to different collection methods, for instance,getIndexes()
which is described below.getIndexes()
- We use this method to get an array holding a documents’ list that identifies and describes all the existing indexes on a particular collection, including the hidden indexes.
Is there a way to see hidden indexes for a particular collection? YES. We can see them using the listIndexes
, as given below.
Here, the Client
is the collection name. You can replace this with your collection name.
Example Code:
// MongoDB Version 5.0
> db.runCommand (
{
listIndexes: "Client"
}
);
OUTPUT:
{
"cursor" : {
"id" : NumberLong(0),
"ns" : "test.Client",
"firstBatch" : [
{
"v" : 2,
"key" : {
"_id" : 1
},
"name" : "_id_"
},
{
"v" : 2,
"key" : {
"shopPosId" : 1
},
"name" : "shopPosId_1"
}
]
},
"ok" : 1
}
The output above contains the firstBatch
, which describes the index information, including the keys and options used to create an index. As of starting MongoDB 4.4, the index option hidden is only present if its value is true
.
Show the text
Indexes Only From All Collections of All Databases in MongoDB
Example Code:
// MongoDB Version 5.0
> db.adminCommand("listDatabases").databases.forEach(function(database){
let mdb = db.getSiblingDB(database.name);
mdb.getCollectionInfos({ type: "collection" }).forEach(function(collection){
let currentCollection = mdb.getCollection(collection.name);
currentCollection.getIndexes().forEach(function(index){
let indexValues = Object.values(Object.assign({}, index.key));
if (indexValues.includes("text")) {
print("Text index: " + index.name + " on the " +
database.name + "." + collection.name);
printjson(index);
};
});
});
});
OUTPUT:
Text index: emp_code_text on the test.employee
{
"v" : 2,
"key" : {
"_fts" : "text",
"_ftsx" : 1
},
"name" : "emp_code_text",
"weights" : {
"emp_code" : 1
},
"default_language" : "english",
"language_override" : "language",
"textIndexVersion" : 3
}
This code example is similar to the previous one except for the difference that we are targeting the text
type index only from all collections of all databases.