How to Search in the Specified Array in MongoDB
- Understanding the $in Operator
- Searching in a Simple Array
- Searching in a Nested Array
- Conclusion
- FAQ
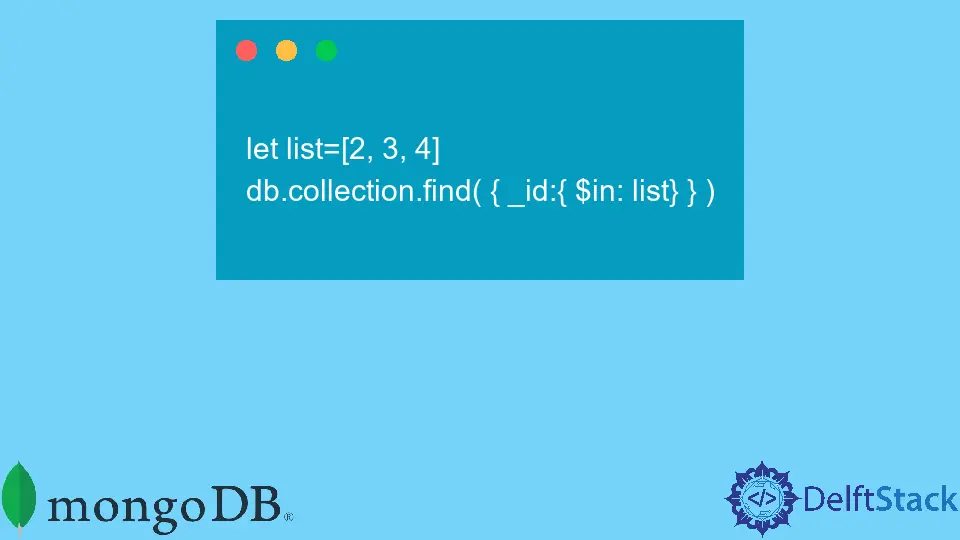
Searching through arrays in MongoDB can seem daunting at first, but with the right tools, it becomes a breeze. One of the most powerful features MongoDB offers for this purpose is the $in
operator. This operator allows you to search for specific values within an array, making it incredibly useful for filtering documents based on array contents. Not only can you use $in
for simple arrays, but it also shines when dealing with nested array objects.
In this article, we will explore how to effectively utilize the $in
operator to search within specified arrays in MongoDB, complete with practical examples and detailed explanations.
Understanding the $in Operator
The $in
operator is a versatile tool that allows you to specify an array of values to match against elements in a field. When you use $in
, MongoDB searches for documents where the specified field contains at least one of the values in the provided array. This makes it particularly effective for filtering data in collections that contain arrays.
For instance, if you have a collection of users and you want to find users who belong to specific roles, you can use $in
to filter the documents. The syntax is straightforward:
{ "fieldName": { "$in": [value1, value2, ...] } }
This simple structure allows for powerful queries that can yield significant insights from your data.
Searching in a Simple Array
To demonstrate how to use the $in
operator, let’s consider a simple example. Imagine you have a collection called products
, and each product document has a field called tags
, which is an array of strings. If you want to find all products that have either the tag “electronics” or “furniture”, you can execute the following query:
db.products.find({
"tags": { "$in": ["electronics", "furniture"] }
})
Output:
{ "_id": 1, "name": "Laptop", "tags": ["electronics", "computers"] }
{ "_id": 3, "name": "Chair", "tags": ["furniture", "home"] }
In this example, the query will return any product documents that contain either “electronics” or “furniture” in their tags
array. This is an efficient way to filter documents based on multiple criteria, allowing you to retrieve relevant data quickly.
The $in
operator is particularly useful when dealing with large datasets. Instead of writing multiple queries or using complex logic, you can simply provide an array of values you want to match. This not only simplifies your code but also enhances performance by reducing the number of operations the database needs to perform.
Searching in a Nested Array
Now, let’s take it a step further and see how the $in
operator works with nested arrays. Suppose you have a collection called orders
, where each order document contains a field called items
, and each item is itself an object with a category
field. If you want to find all orders that contain items in either the “electronics” or “clothing” categories, you can perform the following query:
db.orders.find({
"items.category": { "$in": ["electronics", "clothing"] }
})
Output:
{ "_id": 1, "customer": "John", "items": [{ "category": "electronics" }, { "category": "furniture" }] }
{ "_id": 2, "customer": "Jane", "items": [{ "category": "clothing" }, { "category": "accessories" }] }
In this case, the query searches through the items
array in each order document and checks if any of the item categories match the specified values. The result will include orders that contain at least one item in the desired categories. This ability to query nested arrays makes MongoDB a robust choice for applications that require complex data structures.
Using $in
with nested arrays can significantly simplify your queries. It allows you to target specific subfields within documents, making it easier to extract the data you need without having to write overly complex queries.
Conclusion
Searching within specified arrays in MongoDB is made simple with the $in
operator. Whether you’re dealing with simple arrays or nested objects, this powerful tool allows you to filter documents efficiently and effectively. By leveraging the $in
operator, you can streamline your queries, enhance performance, and gain valuable insights from your data. As you continue to explore MongoDB’s capabilities, you’ll find that mastering operators like $in
can significantly improve your data handling and querying skills.
FAQ
-
What is the $in operator in MongoDB?
The $in operator is used to search for documents where a specified field matches any value in a given array. -
Can I use $in with nested arrays?
Yes, you can use $in to search within nested arrays by specifying the path to the nested field in your query. -
Is $in efficient for large datasets?
Yes, the $in operator is efficient because it allows you to filter documents based on multiple criteria in a single query. -
How do I combine $in with other operators?
You can combine $in with other operators like $and and $or to create more complex queries. -
Can I use $in with different data types?
Yes, $in can be used with various data types, including strings, numbers, and objects, as long as the field types match.