How to Build a MongoDB REST API
- REST API
- Build a MongoDB REST API
- Install Application Dependencies
- Establish a MongoDB REST API Application
- Build a MongoDB REST API Endpoint
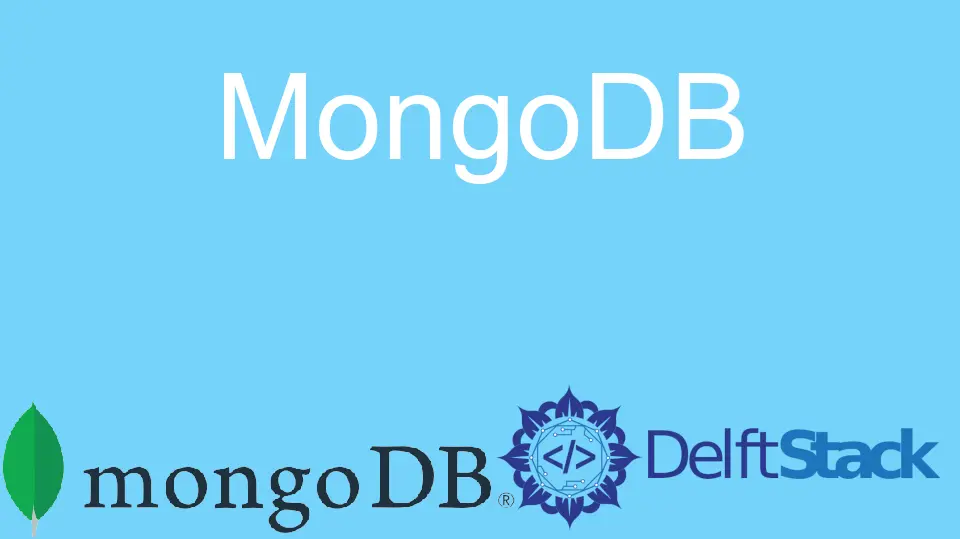
MongoDB is a flexible and scalable document-oriented database system widely used for high-volume data storage. It uses documents and collections instead of the conventional rational database approach that uses tables and rows.
MongoDB has no full-blown REST interface since the server uses the native binary protocol to boost efficiency. However, various applications allow the creation of a MongoDB REST API.
REST API
A REST API, also known as a RESTful application programming interface, adheres to the constraints of the REST architectural style. This ultimately allows for interaction with several other RESTful web services.
REST, in this case, stands for representational state transfer. REST is not a protocol or a standard but a set of architectural constraints that allows developers to implement it in several ways.
For an API to conform to REST constraints, it needs to follow the mentioned criteria:
-
Separated Client-Server
This specific design approach is based on the constraint that the client and server should be separated and catered to independently. Here, the communication between the client and server is limited to the request initiated by the clients and the response sent by the server as a reaction.
This enhances scalability as well as manageability by simplifying the server component.
-
Code on Demand
An API can be RESTful even without this constraint; however, it does help the process. It reduces the number of features that have to be pre-implemented.
-
Stateless
In REST APIs, the server keeps no information on the user that uses the API, meaning they are stateless. The requests sent by the client to the server will carry all required information to ensure the server comprehends and complies with the client’s request.
-
Uniform Interface
To decouple a client, REST API conforms to a uniform interface. This allows for independent application development without linking the application’s models, services, or actions connected to the API layer.
-
Layered
The architecture for a REST API has several layers that work together to create a hierarchy that helps create a scalable and adaptable application. Hide the server's crucial and more susceptible elements behind a firewall, ultimately prohibiting direct interaction with them.
Build a MongoDB REST API
Over time database technologies have become advanced and offer a wide range of capabilities. At the same time, some database systems work better with specific data than others.
MongoDB is an open-source solution with better scalability and performance when dealing with big unstructured data.
When creating a REST API, it is crucial to choose an appropriate database. MongoDB is a great option for your REST API due to its ability to retrieve and store documents making it ideal for Unstructured Data.
The first thing you can do to make sure your MongoDB REST API has a successful start is to prepare your workspace. The first step here is to ensure that your MongoDB instance is operational.
Once that is confirmed, the MongoDB client can connect to the server and further inspect your data or run your queries along with other things.
With the MongoDB REST API, the MongoDB database will need to be populated, which can be done with the mongo
package. This package includes the mongoimport
command that allows you to import data files for your database.
Through this command line, you can import three different kinds of data files, including JavaScript Object Notation (JSON), Comma-Separated Values (CSV), and Tab-Separated Values (TSV).
Furthermore, you will need to create an application folder for the app to reside in. A .json
file package will need to be generated to start the application containing all the node application metadata.
The file then proceeds to instruct npm
to install the package dependencies and the application scripts.
While building the REST API, it is essential to use a backend webserver. Using Express JS
as the backend server for the document store is a common way to do this.
This technique links the JSON-based REST API payloads to MongoDB’s Document Model. Use Express
to create the backend middle tier that runs on Node.js and allows REST API routes to be exposed to your application.
Moreover, the Node.js driver connects the MongoDB Atlas cluster to the Express.js
server.
MongoDB Atlas is a cloud database you will need to sign up for. It allows you to focus on building your MongoDB REST API.
Install Application Dependencies
MongoDB REST API requires a file serving its role as the application command center. When npm
needs to run the application, it will initially run the file.
This command center file can include third-party modules installed and both your modules installed from the npm
directory. The dependencies are the following:
- MongoDB: The official module for MongoDB that allows communication between MongoDB and Node.js applications.
- Express: Node.js framework
- Body-parser: Handles request bodies with Express
Establish a MongoDB REST API Application
You will need a connection string to connect with MongoDB REST API.
- Go to the Atlas dashboard and choose
Clusters
. - Go to the overview page, and click on the
Connect
button. - Add the string to your
.js
file, and you can establish a connection with some code adjustments.
Build a MongoDB REST API Endpoint
To proceed further, you need to establish and query endpoints for your data. You will need to construct an endpoint to add data.
To your .js
application, add API endpoint code to complete the creation of REST API.
MongoDB REST API is relatively simple to set up with the proper instructions. It allows you to store and retrieve data, which makes it perfect for Unstructured Data.
We hope this article helped you gain an understanding of MongoDB REST APIs and how to go about building them.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub