How to Use an ORM in MongoDB
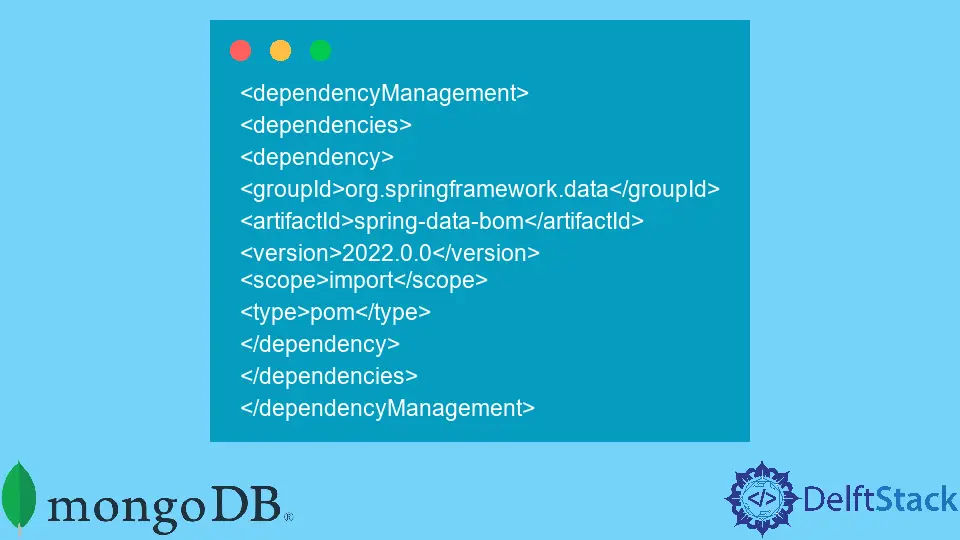
MongoDB introduces a NoSQL solution for data storage and management, consisting of documents represented in JSON style. Like other database systems, MongoDB can also work with an ORM.
In this article, we will explain ORMs’ concept in MongoDB and in general.
Object Relational Mapping (ORM)
Object Relational Mapping is a technique that makes it easier to work with data in relational database systems. It serves as a connecting bridge between your business model and your storage databases.
An ORM works by mapping the data stored as relations in the database to objects in your application’s language. This provides the advantage of the ease of programming to the one writing the code since the data can now be accessed using the same programming language naturally and fluidly.
Let us further explain how this is possible.
When working with an object-oriented language, the programmer has to store data in the business logic layer as objects. This data is simultaneously stored in a relational database in the form of tables or relations.
Translating this relational data to objects while coding might result in an ugly application. It is also more error-prone since the programmer has to work with two separate languages in one application and write raw queries.
Therefore, ORM provides the solution to this problem by simplifying this process.
Note: Removing an ORM from an application after it has been shipped will be very difficult (and expensive!). Therefore, it needs to be decided beforehand if an ORM will simplify the process, introduce performance issues, and proceed accordingly.
ORM in MongoDB
The question now arises since MongoDB is a NoSQL solution and does not use relations. Does an ORM still apply to it? The answer is yes, ORMs can also be used in MongoDB, but here they are more commonly known as ODMs.
ODM stands for Object Document Mapping, replacing the Relational
with Document
since MongoDB stores data in documents.
For NoSQL databases like MongoDB, using an ODM is not that great of an advantage since they are already simpler. But it brings in the added benefit of improving developer productivity, which is essential for the application’s overall performance.
However, ODMs are still extensively used with MongoDB. This is because ODMs can help use the logical relationships between data, which can otherwise not be easily represented since it is impossible to identify such relationships in NoSQL.
Without an ODM, the programmer must manually enforce those code relationships. Since MongoDB has a document-oriented architecture, it is very suitable for integrating with an ODM since the documents can also be referred to as objects.
Therefore, an ODM framework is used along with MongoDB to provide an abstraction over the data model layer.
Note: If you are new to using MongoDB, it is advised to skip using an ODM and work with lower levels of abstraction. This will help you get more familiar with the working of the database software and equip you with its usage in-depth, as opposed to hiding behind the high level of abstraction provided by an ODM.
Examples of ORMs for MongoDB
There are numerous ORM libraries available for MongoDB written for various languages.
Some of them are open-source as well. We will look at a few examples in this article.
Mongoid
Mongoid
is suitable for applications using the Ruby on Rails framework. Using the Mongoid
ODM as a MongoDB ORM for Ruby-based applications, programmers can work more easily with the database resources since they are already familiar with the language, as is the benefit of using an ODM.
As an example, here is a code fragment that inserts an instance into the database using Mongoid
.
Person.create!(
first_name: "Ali",
last_name: "Raza"
) # => Person instance
Following is an example of a MongoDB query written using Mongoid
.
Person.
where(:dob.gte => "1990-01-01").
in(first_name: [ "Ali", "Raza" ]).
union.
in(first_name: [ "Ahmad" ])
Spring Data MongoDB
The most popular choice among developers for a Java-based ORM for MongoDB is Spring Data MongoDB. It provides a consistent Spring-based model for programming new databases.
You can integrate Spring Data MongoDB into your Maven project by using the following dependencies in the pom.xml
file inside the <dependencyManagement>
tag.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-bom</artifactId>
<version>2022.0.0</version>
<scope>import</scope>
<type>pom</type>
</dependency>
</dependencies>
</dependencyManagement>
Note: These dependencies use the latest version when writing this article,
2022.0.0
.
MongoEngine
MongoEngine
is an ODM for MongoDB in Python. It uses a simple high-level API and is built on top of PyMongo
, the official MongoDB driver for Python.
Here is an example of how you can create a database schema in Python using MongoEngine
by defining a class that inherits from Document
.
from mongoengine import *
import datetime
class Page(Document):
title = StringField(max_length=200, required=True)
date_modified = DateTimeField(default=datetime.datetime.utcnow)
And here are some simple queries for accessing the database:
for page in Page.objects:
print page.title
myPage = Page.objects(title="Title")
Conclusion
In this article, we have described in detail the usage of ORMs in MongoDB, which are useful in minimizing the complexity of the development process. An ORM serves as a translator that converts your database documents into objects in the language you are coding, making the task simple for programmers.
We have also discussed the working of a few examples of MongoDB ORMs from a birds-eye view. Going into the details of each of them will not be possible within the scope of this article.
We hope you have learned about the concept of ORM in MongoDB. Keep learning!
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub