How to Fill Between Multiple Lines in Matplotlib
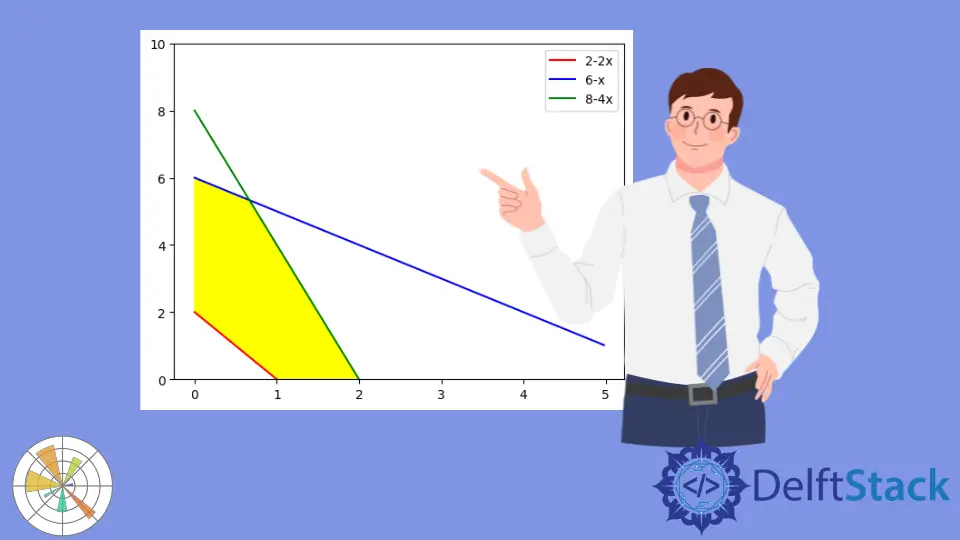
We can fill an area between multiple lines in Matplotlib using the matplotlib.pyplot.fill_between()
method. The fill_between()
function fills the space between two lines at a time, but we can select one pair of lines to fill the area between multiple lines.
import numpy as np
import matplotlib.pyplot as plt
x = np.arange(0, 5, 0.02)
y1 = 8 - 4 * x
y2 = 6 - x
y3 = 2 - 2 * x
plt.plot(x, y1, color="red", label="8-4x")
plt.plot(x, y2, color="blue", label="6-x")
plt.plot(x, y3, color="green", label="2-2x")
plt.legend()
plt.show()
Output:
In the figure, we need to fill the polygon such that the following conditions are satisfied:
y <= 8- 4x
y <= 6 - x
y >= 2 - 2x
y >= 0
Fill Between Multiple Lines in Matplotlib
import numpy as np
import matplotlib.pyplot as plt
x = np.arange(0, 5, 0.02)
y1 = 2 - 2 * x
y2 = 6 - x
y3 = 8 - 4 * x
y4 = np.minimum(y2, y3)
plt.plot(x, y1, color="red", label="2-2x")
plt.plot(x, y2, color="blue", label="6-x")
plt.plot(x, y3, color="green", label="8-4x")
plt.ylim(0, 10)
plt.fill_between(x, y1, y4, color="yellow")
plt.legend()
plt.show()
Output:
To satisfy the given condition above, we set the y-axis limit between 0
to 10
so that y >= 0
as the first step.
As the lines y=6-x
and y=8-4x
intersect with each other, ensuring that y <= 8- 4x
and y <= 6 - x
are both satisfied, we use the minimum()
method to select the points that follow the two conditions.
Finally, we fill the area between y1
and y4
to supply the polygon region specified by the requirement.
Alternatively, we can find the intersection points of lines, which act as the areas’ corner points. Once we know the corner points, we can fill the polygon using the matplotlib.pyplot.fill()
method.
import matplotlib.pyplot as plt
x = [2, 4, 2, 0]
y = [4, 2, 0, 2]
plt.fill(x, y)
plt.show()
Output:
This method fills the polygon with corner points, (2,4)
, (4,2)
, (2,0)
and (0,2)
.
The list x
represents the x co-ordinates of the corner points of the polygon, and the y
represents the y co-ordinates of corner points of the polygon.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn