How to Create Trendline in Matplotlib
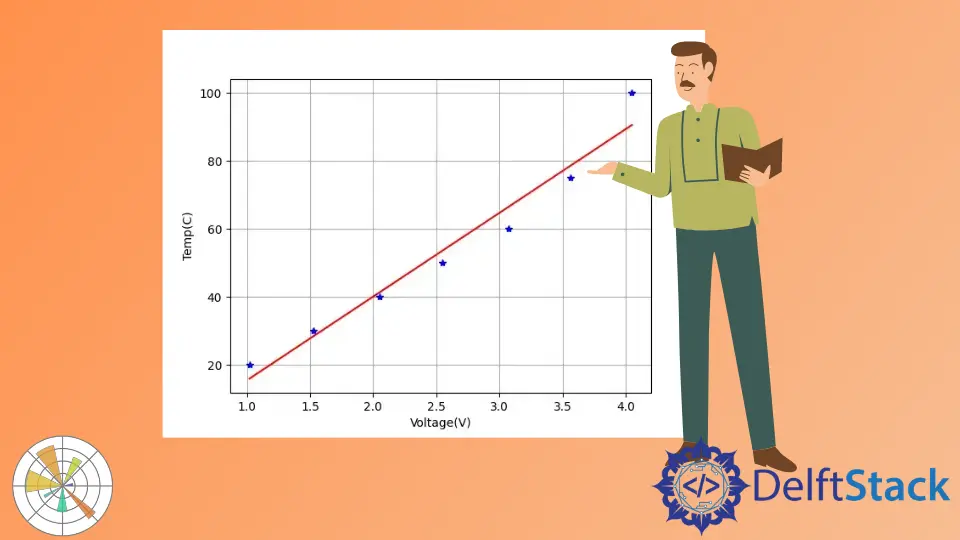
In this demo article, we will have a look at the short demonstration about trendlines and also have a look at how to create a linear trendline onto a graph in Matplotlib.
Use the polyfit()
Method to Create Trendline in Matplotlib
The trendline is simply a line that we draw through the data points in the plot. To estimate the trend and we use them to try to make predictions.
Let’s start with importing the required libraries in Python.
import matplotlib.pyplot as plot
import numpy as np
We have a data vector of the temperature in Celsius and a data vector of a voltage. You could easily import it into your code if you have an excel spreadsheet, a text file, or a CSV file.
In our case, we just typed seven data points manually. We are going to plot temperature as a function of voltage.
T = [20, 30, 40, 50, 60, 75, 100]
V = [1.02, 1.53, 2.05, 2.55, 3.07, 3.56, 4.05]
Source Code:
import matplotlib.pyplot as plot
# Data vectors
T = [20, 30, 40, 50, 60, 75, 100]
V = [1.02, 1.53, 2.05, 2.55, 3.07, 3.56, 4.05]
# Plot data
plot.plot(V, T, "b*")
plot.xlabel("Voltage(V)")
plot.ylabel("Temp(C)")
plot.grid()
plot.show()
Output:
Now we’ll create a trendline. Let’s compute the trend line using the polyfit()
method from numpy
, and this method will create a polynomial fit line.
The polyfit()
method accepts the x-axis, the y-axis, or the side of the X and Y coordinates. The third parameter accepts the order, and we’ll pass it 1
, 1
obviously is linear, so 2
would be quadratic.
coeff = np.polyfit(V, T, 1) # 1=linear
Store the first element of that coefficient into the m
variable, then b
is the y-intercept.
m = coeff[0]
b = coeff[1]
Plot a trendline using the following syntax. We need to call the linspace()
method, and this method takes some initial and ending points with hundred data points.
The Ttrend
variable stores an equation, and this equation will help create a trendline.
Vtrend = np.linspace(V[0], V[-1], 100)
Ttrend = m * Vtrend + b
Source Code:
import matplotlib.pyplot as plot
import numpy as np
# Data vectors
T = [20, 30, 40, 50, 60, 75, 100]
V = [1.02, 1.53, 2.05, 2.55, 3.07, 3.56, 4.05]
# Plot data
plot.plot(V, T, "b*")
plot.xlabel("Voltage(V)")
plot.ylabel("Temp(C)")
plot.grid()
# Compute the trendline
coeff = np.polyfit(V, T, 1) # 1=linear
m = coeff[0]
b = coeff[1]
Vtrend = np.linspace(V[0], V[-1], 100)
Ttrend = m * Vtrend + b
plot.plot(Vtrend, Ttrend, "r")
plot.show()
Output:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn