Matplotlib에서 추세선 만들기
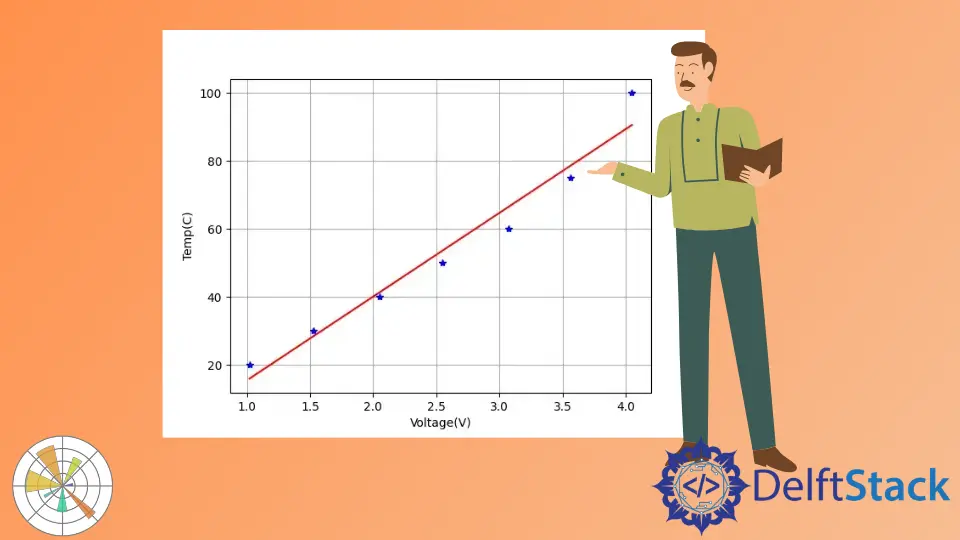
이 데모 기사에서는 추세선에 대한 짧은 데모를 살펴보고 Matplotlib의 그래프에 선형 추세선을 만드는 방법도 살펴봅니다.
polyfit()
메서드를 사용하여 Matplotlib에서 추세선 생성
추세선은 단순히 플롯의 데이터 포인트를 통해 그리는 선입니다. 추세를 추정하고 이를 사용하여 예측을 시도합니다.
Python에서 필요한 라이브러리를 가져오는 것부터 시작하겠습니다.
import matplotlib.pyplot as plot
import numpy as np
섭씨 온도의 데이터 벡터와 전압의 데이터 벡터가 있습니다. Excel 스프레드시트, 텍스트 파일 또는 CSV 파일이 있는 경우 코드로 쉽게 가져올 수 있습니다.
우리의 경우에는 7개의 데이터 포인트를 수동으로 입력했습니다. 전압의 함수로 온도를 플롯할 것입니다.
T = [20, 30, 40, 50, 60, 75, 100]
V = [1.02, 1.53, 2.05, 2.55, 3.07, 3.56, 4.05]
소스 코드:
import matplotlib.pyplot as plot
# Data vectors
T = [20, 30, 40, 50, 60, 75, 100]
V = [1.02, 1.53, 2.05, 2.55, 3.07, 3.56, 4.05]
# Plot data
plot.plot(V, T, "b*")
plot.xlabel("Voltage(V)")
plot.ylabel("Temp(C)")
plot.grid()
plot.show()
출력:
이제 우리는 추세선을 만들 것입니다. numpy
의 polyfit()
메서드를 사용하여 추세선을 계산해 보겠습니다. 이 메서드는 다항식 맞춤선을 생성합니다.
polyfit()
메서드는 x축, y축 또는 X 및 Y 좌표의 측면을 허용합니다. 세 번째 매개변수는 순서를 받아들이고 1
을 전달합니다. 1
은 분명히 선형이므로 2
는 2차가 됩니다.
coeff = np.polyfit(V, T, 1) # 1=linear
해당 계수의 첫 번째 요소를 m
변수에 저장하면 b
는 y 절편입니다.
m = coeff[0]
b = coeff[1]
다음 구문을 사용하여 추세선을 그립니다. 우리는 linspace()
메서드를 호출해야 하며 이 메서드는 100개의 데이터 포인트가 있는 초기 및 종료 지점을 취합니다.
Ttrend
변수는 방정식을 저장하고 이 방정식은 추세선을 만드는 데 도움이 됩니다.
Vtrend = np.linspace(V[0], V[-1], 100)
Ttrend = m * Vtrend + b
소스 코드:
import matplotlib.pyplot as plot
import numpy as np
# Data vectors
T = [20, 30, 40, 50, 60, 75, 100]
V = [1.02, 1.53, 2.05, 2.55, 3.07, 3.56, 4.05]
# Plot data
plot.plot(V, T, "b*")
plot.xlabel("Voltage(V)")
plot.ylabel("Temp(C)")
plot.grid()
# Compute the trendline
coeff = np.polyfit(V, T, 1) # 1=linear
m = coeff[0]
b = coeff[1]
Vtrend = np.linspace(V[0], V[-1], 100)
Ttrend = m * Vtrend + b
plot.plot(Vtrend, Ttrend, "r")
plot.show()
출력:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn