Modulo in MATLAB
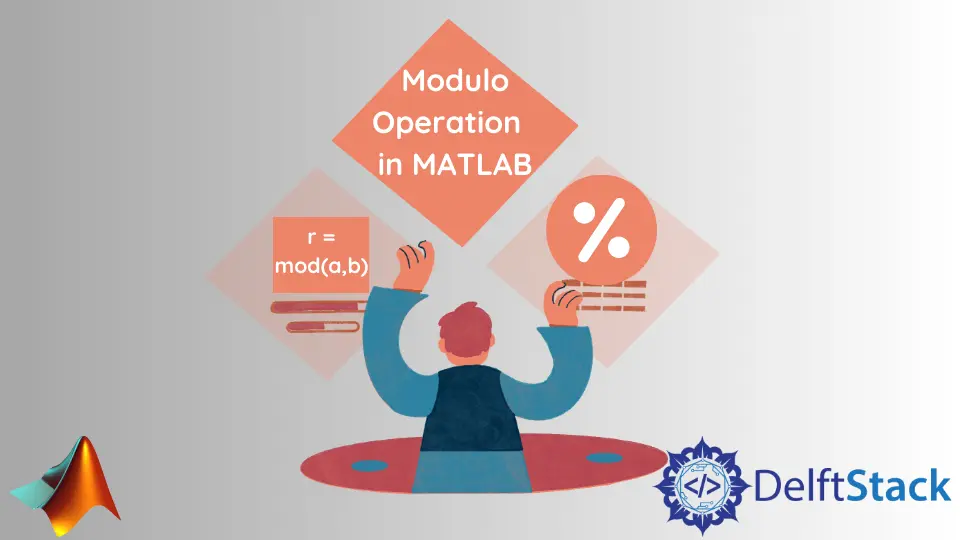
MATLAB, a powerful numerical computing environment, offers a multitude of functions and operations to simplify complex mathematical tasks. One such operation that proves invaluable in various applications is the modulo operation.
Often denoted by the symbol %
, the modulo operation calculates the remainder when one number is divided by another. In MATLAB, understanding how to use the modulo operation opens up new avenues for handling data, implementing algorithms, and solving mathematical problems.
In this article, we will delve into the basics of the modulo operation in MATLAB, exploring its syntax, applications, and practical examples.
How to Use the Modulo Operation in MATLAB
The modulo operation, denoted by %
and represented as mod
in MATLAB, computes the remainder when one integer (the dividend) is divided by another (the divisor). This operation is especially valuable in scenarios involving cyclic or periodic calculations, making it applicable in fields such as Modular Arithmetic and Cryptography.
The basic syntax for the modulo operation in MATLAB is:
remainder = mod(dividend, divisor);
Here, dividend
is the number to be divided, divisor
is the value by which the division occurs, and remainder
is the result, representing the remainder.
Example 1: Modulo Operation With Scalar Values
Let’s demonstrate the basic use of the modulo operation with scalar values.
result = mod(51, 7);
disp(result);
In this example, we utilize the mod
function to find the remainder when 51
is divided by 7
. The result is stored in the variable result
, and we use the disp
function to display the outcome.
In this case, the modulo operation yields a remainder of 2
.
Output:
2
Example 2: Modulo Operation With Vector Values
The modulo operation can also be applied to each element of a vector with a scalar divisor. Here, the mod
function is used to perform element-wise modulo operation on the vector.
vector = 2:9;
modResult = mod(vector, 7)
Here, we apply the mod
function to each element of the vector [2, 3, 4, 5, 6, 0, 1, 2]
when divided by 7
. The resulting remainders are stored in the modResult
variable, and we use disp
to showcase the outcome.
The vector represents the remainder after performing the modulo operation on each element.
Output:
modResult =
2 3 4 5 6 0 1 2
Example 3: Modulo Operation With Positive and Negative Values
The example below showcases the modulo operation with a vector dividend with negative values and a positive divisor.
values = [-6 -3 5 6];
modNegative = mod(values, 7)
This example demonstrates the modulo operation on a vector containing both positive and negative values. The mod
function calculates the remainders when each element is divided by 7
.
The results are stored in the modNegative
variable and displayed using disp
. The output represents the remainder in each case.
Output:
modNegative =
1 4 5 6
Example 4: Modulo Operation With Negative Divisor
Here, we’ll see how the modulo operation is performed when the divisor is a negative value.
values = [-6 -3 5 6];
modNegativeDivisor = mod(values, -7)
In this case, the modulo operation is applied to a vector with a negative divisor (-7
). The mod
function calculates the remainders, and the results are stored in the modNegativeDivisor
variable.
The output, displayed using disp
, showcases the remainders for each element in the vector.
Output:
modNegativeDivisor =
-6 -3 -2 -1
Example 5: Modulo Operation With Floating Point Values
We can also use the modulo operation with floating-point values. Let’s see how this works:
theta = [0.0 3.5 5.9 6.2 9.0 4*pi];
modFloating = mod(theta, 7*pi)
This example demonstrates the mod
operation on a vector of floating-point values using a floating-point divisor (7*pi
). The remainders are calculated and stored in the modFloating
variable, which is then displayed using disp
.
The output represents the remainder after performing the modulo operation on each floating-point element.
Output:
modFloating =
0.00000 3.50000 5.90000 6.20000 9.00000 12.56637
Other Uses of the Modulo Operation in MATLAB
Let’s explore the practical uses and applications of the modulo operation in MATLAB.
Checking for Even or Odd Numbers
The modulo operation is a handy tool for determining whether a number is even or odd.
number = 15;
isEven = mod(number, 2) == 0;
isOdd = mod(number, 2) == 1;
disp(['Is ', num2str(number), ' even? ', num2str(isEven), ', Is it odd? ', num2str(isOdd)]);
In this code, we use the modulo operation to check whether a given number (number
) is even or odd. The remainder, when the number is divided by 2
, is checked.
If the remainder is 0
, the number is even; otherwise, it’s odd. The results are then displayed using the disp
function.
Output:
Is 15 even? 0, Is it odd? 1
Wrap-Around Indexing
In scenarios where you need to perform circular or wrap-around indexing, the modulo operation ensures that indices stay within the array bounds. This is particularly useful in applications such as signal processing or circular buffers:
index = 8;
arrayLength = 5;
index = mod(index - 1, arrayLength) + 1;
disp(['Modified Index: ', num2str(index)]);
Here, the original index is adjusted using the modulo operation, which calculates the remainder when subtracting 1
and dividing by the array length. The adjusted index is then displayed.
Output:
Modified Index: 3
Binary Operations
The modulo operation is also employed in extracting individual binary digits from a decimal number:
decimalNumber = 27;
position = 4;
binaryDigit = mod(floor(decimalNumber ./ 2.^position), 2);
disp(['Binary digit at position ', num2str(position), ': ', num2str(binaryDigit)]);
This code extracts an individual binary digit from a decimal number (27
, in this case) at a specified position (4
). The modulo operation is used along with the floor
division to isolate the desired binary digit, which is then displayed.
Output:
Binary digit at position 4: 1
Generating Sequences
For generating periodic signals or sequences with a specified period, the modulo operation comes in handy:
n = 1:10;
period = 3;
sequence = mod(n, period);
disp(['Generated Sequence: ', num2str(sequence)]);
Here, the modulo operation is applied to generate a repeating sequence with a specified period (3
). The sequence is created by taking the remainder when dividing consecutive integers by the specified period.
The resulting sequence is then displayed.
Output:
Generated Sequence: 1 2 0 1 2 0 1 2 0 1
Checking for Multiples
In order to check if a number is a multiple of another, the modulo operation is applied:
number = 21;
multiple = 7;
isMultiple = mod(number, multiple) == 0;
disp(['Is ', num2str(number), ' a multiple of ', num2str(multiple), '? ', num2str(isMultiple)]);
This code checks whether a given number (21
, in this case) is a multiple of another number (7
). The modulo operation is used to find the remainder when dividing the number by the multiple.
If the remainder is 0
, isMultiple
is set to true, indicating that the number is a multiple. The result is then displayed.
Output:
Is 21 a multiple of 7? 1
Clock Arithmetic
In time-related calculations, modulo is frequently employed to ensure that hours stay within a 24-hour range:
hours = 20;
offset = 5;
hours = mod(hours + offset, 24);
disp(['Adjusted Hours: ', num2str(hours)]);
This code illustrates clock arithmetic, where the hours (20
in this case) are adjusted by adding an offset (5
) and applying the modulo operation to wrap around within a 24-hour clock.
The adjusted hours are then displayed.
Output:
Adjusted Hours: 1
This is crucial for applications like timekeeping.
Conclusion
In MATLAB, the modulo operation proves to be a versatile tool in MATLAB that facilitates various mathematical operations and simplifies complex programming tasks. Whether it’s for basic arithmetic, indexing, binary manipulations, or handling periodic data, mastering the use of the modulo operation enhances your capabilities as a MATLAB programmer.
The examples provided serve as a solid foundation for incorporating this operation into your MATLAB projects, allowing for more efficient and elegant solutions to various mathematical and programming challenges.
As you continue to explore MATLAB’s vast functionalities, keep the modulo operation in mind as a valuable asset for handling diverse mathematical scenarios.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook