How to Swap Rows and Columns in MATLAB
- How to Swap Rows and Columns in MATLAB Using Indexing
- How to Swap Rows and Columns in MATLAB Using a User-Defined Function
-
How to Swap Rows and Columns in MATLAB Using
randperm()
andsize()
Functions -
How to Swap Rows and Columns in MATLAB Using the
transpose()
Function - Conclusion
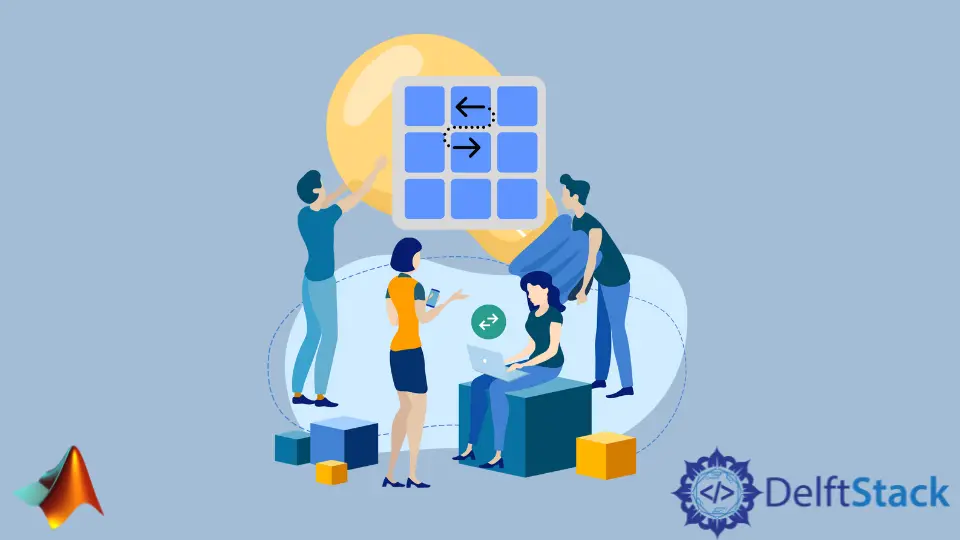
MATLAB, a powerful numerical computing environment, provides various methods for manipulating matrices, including swapping rows and columns. Swapping rows and columns is a common operation in data manipulation, and MATLAB offers flexible and efficient approaches to achieve this.
In this article, we will explore different methods, from basic indexing to more advanced techniques, to help you understand how to swap rows and columns in MATLAB.
How to Swap Rows and Columns in MATLAB Using Indexing
Before diving into swapping rows and columns, it’s important to understand how MATLAB indexes matrices.
MATLAB follows a 1-based indexing system, meaning the first element in a matrix is accessed using the index 1, not 0. Additionally, MATLAB supports a versatile indexing syntax that allows you to access and manipulate elements or submatrices with ease.
Swap Rows With Indexing
To swap rows in MATLAB using indexing, you can leverage square brackets and the colon operator. Here’s an example:
A = [1 2 3 4; 5 6 7 8; 9 10 11 12]
A([1, 3], :) = A([3, 1], :)
In this case, B(:, [2, 4])
selects all rows from columns 2 and 4. The subsequent assignment, = B(:, [4, 2])
, replaces the content of columns 2 and 4 with each other.
Again, the colon :
ensures that all rows are included in the swapping operation.
Output:
How to Swap Rows and Columns in MATLAB Using a User-Defined Function
In MATLAB, custom functions can be created to encapsulate logic for swapping rows and columns, enhancing code modularity and readability.
Let’s create a MATLAB function named swapRC
that takes a matrix, a dimension indicator (1 for rows, 2 for columns), and indices of elements to be swapped within that dimension:
% Define the swapRC function
function matrix = swapRC(matrix, dimension, a, b)
if dimension == 1
% Swap rows
rowA = matrix(a, :);
matrix(a, :) = matrix(b, :);
matrix(b, :) = rowA;
elseif dimension == 2
% Swap columns
colA = matrix(:, a);
matrix(:, a) = matrix(:, b);
matrix(:, b) = colA;
end
end
The swapRC
function is designed to swap rows or columns of a matrix based on the specified dimension.
When the dimension is 1 (rows), it swaps the content of the specified rows. When the dimension is 2 (columns), it swaps the content of the specified columns.
The temporary variables (rowA
and colA
) facilitate the swapping process, ensuring that matrix modifications are done correctly.
Swap Rows Using the User-Defined Function
Let’s now use the swapRC
function to swap rows within a matrix:
C = [1 2 3 4; 5 6 7 8; 9 10 11 12]
swapRC(C, 1, 1, 3)
In this example, size(E, 1)
returns the number of rows in matrix E
. randperm()
then generates a random permutation of row indices, and this permutation is used to rearrange the rows of matrix E
.
The colon :
ensures that all columns are included in the swapping operation.
Output:
Swap Columns With randperm()
and size()
For randomly swapping columns, we can use a similar approach:
F = [1 2 3 4; 5 6 7 8; 9 10 11 12]
SwappedColumns = F(:, randperm(size(F, 2)))
Here, F(:, randperm(size(F, 2)))
follows a similar logic. size(F, 2)
determines the number of columns in matrix F
. randperm()
generates a random permutation of column indices, and this permutation is used to rearrange the columns of matrix F
using the colon :
to include all rows.
Output:
This method introduces randomness into the swapping process, which can be useful in scenarios where a random order is desired.
How to Swap Rows and Columns in MATLAB Using the transpose()
Function
MATLAB’s transpose()
function provides a convenient way to swap rows and columns in a matrix. This operation involves interchanging rows with columns, effectively transforming a matrix into its transpose.
If A
is an m-by-n matrix, the result of transpose(A)
is an n-by-m matrix.
Let’s create a MATLAB script that demonstrates how to swap rows and columns using the transpose()
function:
matrix = [1 2 3; 4 5 6; 7 8 9];
disp('Original Matrix:');
disp(matrix);
swappedMatrix = transpose(matrix);
disp('Matrix after Swapping:');
disp(swappedMatrix);
Here, we apply the transpose()
function to the original matrix
to swap its rows with columns, creating a new matrix named swappedMatrix
. To visually inspect the result of this operation, we proceed to display the swappedMatrix
using the disp()
function again.
Output:
If you want to swap specific rows and columns using transpose()
, you can do so by transposing a submatrix. Here’s an example:
matrix = [1 2 3; 4 5 6; 7 8 9];
disp('Original Matrix:');
disp(matrix);
subMatrix = matrix([2 3], [1 2]);
swappedMatrix = transpose(subMatrix);
matrix([2 3], [1 2]) = swappedMatrix;
disp('Matrix after Swapping Selected Rows and Columns:');
disp(matrix);
In this example, we first extract the submatrix that needs swapping, transpose it, and then update the original matrix with the transposed values. This allows for more targeted swapping operations.
Output:
The transpose()
function in MATLAB provides a simple and effective way to swap rows and columns in a matrix. Whether applied to the entire matrix or a selected subset, the function’s inherent behavior of interchanging rows and columns makes it a versatile tool for matrix manipulation.
Conclusion
MATLAB provides multiple methods for swapping rows and columns in matrices, each with its advantages. Understanding these techniques allows you to choose the approach that best fits your specific needs, providing flexibility and efficiency in matrix manipulations.
Whether opting for simplicity with basic indexing, modularity with user-defined functions, randomness with randperm()
and size()
, or elegance with transpose()
, MATLAB offers a versatile toolkit for matrix operations.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook