How to Reverse Colormap in MATLAB
- MATLAB Reverse Colormap
-
Use the
flipud()
Function to Reverse a Colormap -
Use the
flip()
Function to Reverse a Colormap - Conclusion
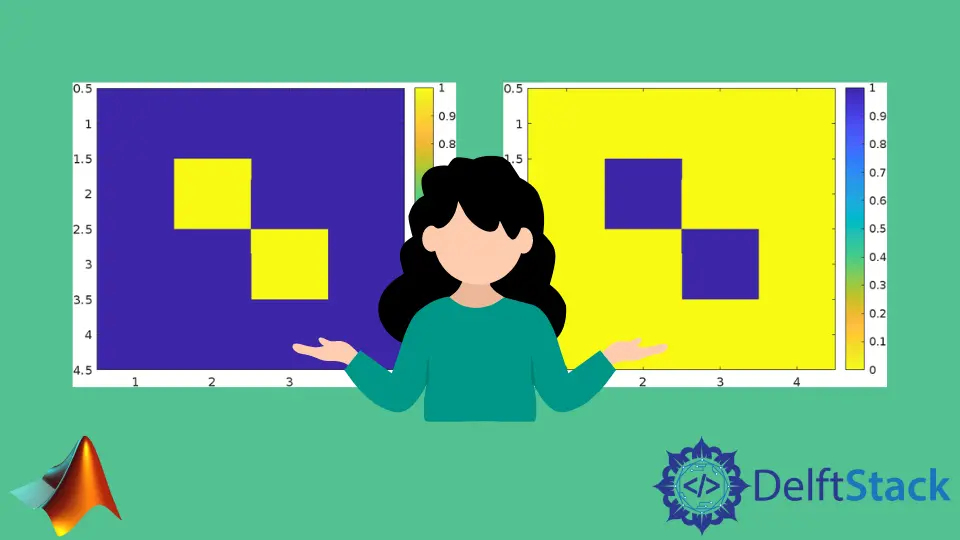
This tutorial will discuss reversing a colormap using the flipud()
and flip()
functions in MATLAB.
MATLAB Reverse Colormap
The colormap sets the colors of a graphics object like an image. A colormap is a matrix of values that uses RGB triplet values to assign colors to values of a matrix or array.
In Matlab, an image is stored in a matrix form and contains numbers that define its colors. When we use a function like imshow()
to show the matrix as an image, the function assigns color values to the image using a colormap.
Use the flipud()
Function to Reverse a Colormap
To reverse a colormap, we can use the flipud()
function to flip the values of a matrix or array. The flipud()
function in MATLAB is used to reverse the order of elements along the first dimension of an array.
When applied to a colormap, it effectively reverses the order of colors in the colormap. The colormap is also a matrix containing the values of colors so that we can use the flipud()
function to reverse the colors present in the colormap.
If we reverse a colormap, the colors present in an image and other objects that MATLAB is currently showing will be reversed. For example, let’s create a binary image and reverse its colormap using the flipud()
function.
Example Code:
clc
clear
v = [0 0 0 0;0 1 0 0;0 0 1 0;0 0 0 0];
figure(1)
imshow(v,'InitialMagnification','fit')
colorbar
figure(2)
imshow(v,'InitialMagnification','fit')
colorbar
colormap(flipud(gray))
Output:
In the above code, we created two figures of the binary image using the imshow()
function. The first figure shows the original image and color bar with the original colormap.
In the second figure, we have reversed the colormap of the first figure. In the output, the left figure shows the image with the original colormap, and the right figure shows the image with the reversed colormap.
We can see in the output that the colors are reversed. The white color is converted to black, and the black color is converted to white.
In the above code, we used the magnification property of the imshow()
function to fit the given image to the figure because the image is very small, but if the size of the input image is large or we don’t want to magnify the given image, we can neglect it.
In the above example, we used a binary image with only two colors but can also perform this operation with a colored image.
If we want to flip the colormap of a colored image, we have to change the last line of the above code in which we are flipping the gray colormap, but we have to use the colormap in place of gray.
For example, let’s use the imagesc()
function to create a color image from a matrix and reverse its colormap using the flipud()
function.
Example Code:
clc
clear
v = [0 0 0 0;0 1 0 0;0 0 1 0;0 0 0 0];
figure(1)
imagesc(v)
colorbar
figure(2)
imagesc(v)
colorbar
colormap(flipud(colormap))
Output:
The imagesc()
function displays an image with scaled colors. If the input of this function is a matrix, the function will assign each value a different color from a colormap and display it.
In the above code, we are flipping the previous colormap and assigning the new values to the new colormap.
In the above output, the left figure shows the image with the original colormap, and the right figure shows the image with the reversed colormap. We can see in the above output that the image’s color and the color bar have been changed.
Check this link for more details about the colormap. Check this link for more details about the flipud()
function.
Use the flip()
Function to Reverse a Colormap
The flip()
function in MATLAB is another option for reversing the order of elements along a specified dimension of an array. While flipud()
specifically flips an array along its first dimension, the flip()
function allows you to choose the dimension along which the flipping occurs.
Basic Syntax:
B = flip(A, dim);
In the syntax, the A
is the input array, and dim
is the dimension along which the flipping occurs. If dim
is not specified, the array is flipped along its first dimension by default.
Example Code:
clc
clear
v = [0 0 0 0; 0 1 0 0; 0 0 1 0; 0 0 0 0];
figure(1)
imagesc(v)
colorbar
reversedColormap = flip(colormap);
colormap(reversedColormap)
figure(2)
imagesc(v)
colorbar
In the above code, we define a 4x4 binary image matrix 'v'
. In Figure 1, we use the imagesc()
function to display the original image along with its associated color bar.
Next, we reverse the colormap using flip(colormap)
and apply it to Figure 1 with colormap(reversedColormap)
. Figure 2 then displays the same image matrix, but now with the reversed colormap, allowing us to observe the color transformation.
The color bars in both figures provide a visual comparison of the original and reversed colormaps.
Output:
The final output is described as having two figures. The left one shows the original image with its original colormap, while the right one displays the same image but with the reversed colormap.
Conclusion
In conclusion, this tutorial delves into reversing colormaps in MATLAB using flipud()
and flip()
functions. It highlights the role of colormaps in defining graphics object colors and demonstrates the practical application of flipud()
on a binary image.
The versatility of colormap reversal is showcased using the flip()
function with examples, emphasizing its flexibility along specified dimensions. Visual outputs and clear explanations enhance the learning experience.
Overall, the tutorial equips users with insights into colormap manipulation for effective data visualization, encouraging further exploration through MATLAB documentation links. The transformative impact of colormap reversal is summarized with a side-by-side comparison of original and reversed colormap images.