How to Check if String Contains a Substring in Bash
-
Method 1: Using the
[[
Conditional Expression -
Method 2: Using the
grep
Command -
Method 3: Using the
case
Statement -
Method 4: Using the
expr
Command - Conclusion
- FAQ
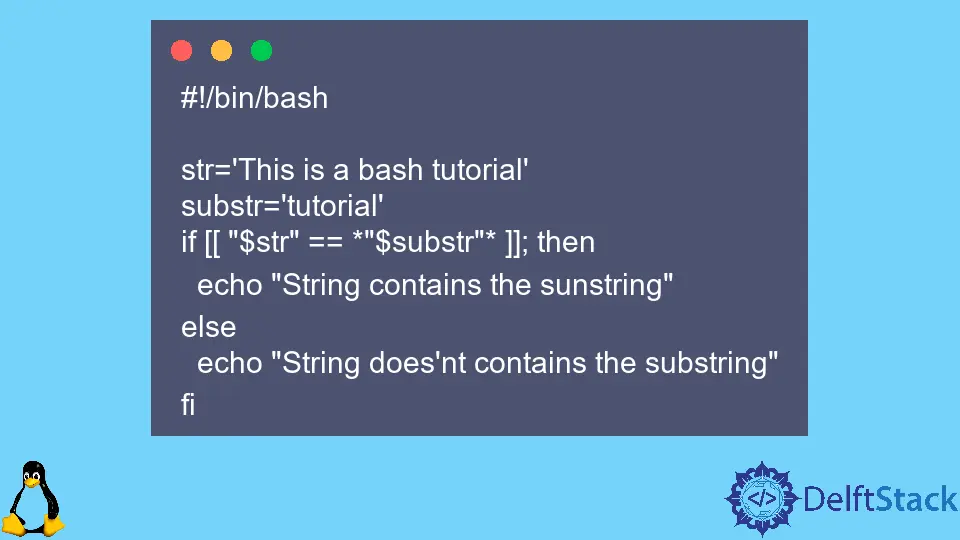
In the world of programming, checking if a string contains a substring is a common task, especially when working with data processing or text manipulation. When using Bash, a powerful shell scripting language, you might find yourself needing to determine if a specific substring exists within a larger string. This can be particularly useful in scenarios such as parsing logs, filtering outputs, or even when working with Git commit messages.
In this tutorial, we will explore several effective methods to achieve this in Bash, ensuring that you can easily integrate these techniques into your scripts. So, let’s dive in and learn how to check for substrings in Bash!
Method 1: Using the [[
Conditional Expression
One of the simplest ways to check if a string contains a substring in Bash is by using the [[
conditional expression. This method is straightforward and allows for easy readability. Here’s how you can do it:
string="Hello, welcome to the world of Bash scripting."
substring="Bash"
if [[ $string == *$substring* ]]; then
echo "Substring found!"
else
echo "Substring not found."
fi
Output:
Substring found!
In this example, we define a string and a substring. The [[
construct allows us to use pattern matching with the *
wildcard. If the substring exists within the string, the condition evaluates to true, and we print “Substring found!” Otherwise, we print “Substring not found.” This method is efficient and works well for most cases.
Method 2: Using the grep
Command
Another popular method for checking if a string contains a substring is by leveraging the grep
command. This approach is particularly useful when dealing with larger datasets or when you want to perform more complex pattern matching. Here’s how you can implement it:
string="Hello, welcome to the world of Bash scripting."
substring="Bash"
if echo "$string" | grep -q "$substring"; then
echo "Substring found!"
else
echo "Substring not found."
fi
Output:
Substring found!
In this method, we use the echo
command to send the string to grep
, which searches for the substring. The -q
flag tells grep
to operate quietly, meaning it will not output anything, but it will set the exit status based on whether the substring was found. This allows us to use the exit status in our conditional statement. If the substring is present, we print “Substring found!” Otherwise, we print “Substring not found.” This method is powerful and can be adapted for more complex searches.
Method 3: Using the case
Statement
The case
statement in Bash is another effective way to check for substrings. This method is particularly useful when you have multiple substrings to check against a single string. Here’s how it works:
string="Hello, welcome to the world of Bash scripting."
substring="Bash"
case "$string" in
*"$substring"*)
echo "Substring found!"
;;
*)
echo "Substring not found."
;;
esac
Output:
Substring found!
In this example, we use the case
statement to evaluate the string against patterns. The *
wildcard allows for flexible matching. When the substring is found, the corresponding block executes, printing “Substring found!” If it’s not found, the default case executes, resulting in “Substring not found.” This method is particularly neat and allows for easy expansion if you want to check for multiple substrings.
Method 4: Using the expr
Command
The expr
command is another option for checking if a string contains a substring. Although it’s less commonly used nowadays, it can still be effective in certain scenarios. Here’s how you can apply it:
string="Hello, welcome to the world of Bash scripting."
substring="Bash"
if expr "$string" : ".*$substring.*" > /dev/null; then
echo "Substring found!"
else
echo "Substring not found."
fi
Output:
Substring found!
In this method, we use expr
to evaluate if the substring exists within the string. The : ".*$substring.*"
pattern checks for the presence of the substring. The output is redirected to /dev/null
to suppress it, allowing us to focus on the exit status. If the substring is found, we print “Substring found!” Otherwise, we print “Substring not found.” While this method is effective, it’s worth noting that it may not be as intuitive as the other methods discussed.
Conclusion
In this tutorial, we explored various methods to check if a string contains a substring in Bash. From using the [[
conditional expression to leveraging the grep
command, case
statements, and even the expr
command, you now have a toolkit of techniques to choose from based on your specific needs. Whether you’re parsing log files, filtering outputs, or handling Git commits, these methods will enhance your Bash scripting skills. Remember, the right method often depends on the context of your task, so feel free to experiment and find what works best for you!
FAQ
-
How can I check for a case-insensitive substring in Bash?
You can convert both the string and the substring to lowercase usingtr
and then perform the check. -
Is it possible to check for multiple substrings at once?
Yes, you can use acase
statement or loop through an array of substrings to check each one. -
What is the best method for large strings?
Usinggrep
is often more efficient for larger strings or files due to its optimized search capabilities. -
Can I use regular expressions to find substrings in Bash?
Yes, you can usegrep
with regular expressions for more complex matching patterns. -
Are there any performance considerations when using these methods?
Generally, the[[
andgrep
methods are faster for most cases, whileexpr
may be slower for larger strings.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn