How to Solve Unary Operator Expected Error in Bash
- Understanding the Unary Operator Expected Error
- Solution 1: Check for Empty Variables
- Solution 2: Use Double Brackets for Conditional Expressions
- Solution 3: Ensure Proper Syntax in Conditional Statements
- Conclusion
- FAQ
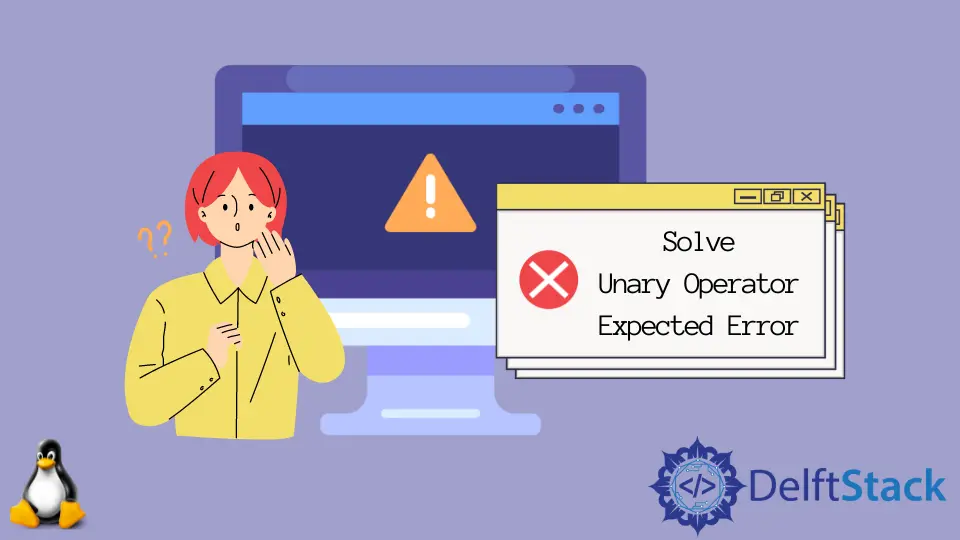
When working with Bash scripts, encountering errors can be frustrating, especially when they seem cryptic. One common error that developers face is the “unary operator expected” error. This error typically arises when a conditional expression in a script is misconfigured, often due to missing variables or incorrect syntax. Understanding how to effectively troubleshoot and resolve this error can save you time and prevent headaches in your development process.
In this article, we will delve into the causes of the unary operator expected error in Bash and provide clear, actionable solutions to resolve it. Whether you are a novice or an experienced developer, you’ll find valuable insights to help you navigate this issue.
Understanding the Unary Operator Expected Error
Before diving into solutions, it’s essential to understand what triggers the unary operator expected error. This error usually occurs in conditional statements, such as if
statements, when Bash expects a unary operator but doesn’t find one. The most common culprits include:
- Missing variables: If a variable is not set or is empty, Bash can’t evaluate the condition properly.
- Incorrect syntax: A misplaced operator or incorrect use of brackets can lead to this error.
By addressing these common issues, you can effectively eliminate the unary operator expected error from your scripts.
Solution 1: Check for Empty Variables
One of the primary reasons for the unary operator expected error is the use of uninitialized or empty variables in your conditional statements. To resolve this, ensure that the variables you are checking are properly initialized before the condition is evaluated. Here’s an example:
#!/bin/bash
var=""
if [ -z "$var" ]; then
echo "Variable is empty."
else
echo "Variable is not empty."
fi
In this script, we check if the variable var
is empty using the -z
operator. If var
is indeed empty, the script will print “Variable is empty.” Otherwise, it will print “Variable is not empty.”
Output:
Variable is empty.
In this case, using the -z
operator correctly avoids the unary operator expected error. Always ensure that your variables are initialized and that you use the appropriate operators for your conditions.
Solution 2: Use Double Brackets for Conditional Expressions
Another effective way to solve the unary operator expected error is to use double brackets [[ ]]
instead of single brackets [ ]
. Double brackets provide more flexibility and better error handling in Bash. Here’s how you can implement this:
#!/bin/bash
var="Hello"
if [[ "$var" == "Hello" ]]; then
echo "The variable matches."
else
echo "The variable does not match."
fi
In this example, we use double brackets to check if the variable var
equals “Hello.” Double brackets allow for more complex expressions and reduce the likelihood of encountering the unary operator expected error.
Output:
The variable matches.
Using double brackets not only makes your code cleaner but also enhances its robustness. This method is particularly useful when dealing with string comparisons or more complex logical conditions.
Solution 3: Ensure Proper Syntax in Conditional Statements
Syntax errors in your conditional statements can also lead to the unary operator expected error. It’s crucial to ensure that your syntax is correct, especially when using logical operators. Here’s an example of how to correctly structure your conditional statements:
#!/bin/bash
var1="Hello"
var2="World"
if [ "$var1" == "Hello" ] && [ "$var2" == "World" ]; then
echo "Both conditions are true."
else
echo "One or both conditions are false."
fi
In this script, we check two conditions using the logical AND operator &&
. Each condition is enclosed in its own set of brackets, which is essential to avoid syntax errors.
Output:
Both conditions are true.
Ensuring that your syntax is correct can help you avoid the unary operator expected error. Always double-check your brackets and operators when writing conditional expressions.
Conclusion
Encountering the unary operator expected error in Bash can be a common challenge, but understanding its causes and knowing how to address them can make a significant difference in your scripting experience. By checking for empty variables, using double brackets for conditional expressions, and ensuring proper syntax, you can effectively resolve this error and enhance the reliability of your scripts. As you continue to develop your Bash scripting skills, remember these solutions to keep your code clean and error-free.
FAQ
-
what causes the unary operator expected error in Bash?
The error is typically caused by uninitialized or empty variables in conditional statements or incorrect syntax. -
how can I check if a variable is empty in Bash?
You can use the-z
operator within anif
statement to check if a variable is empty. -
why should I use double brackets in Bash?
Double brackets provide better error handling and allow for more complex expressions in conditional statements. -
can syntax errors lead to the unary operator expected error?
Yes, improper syntax, such as misplaced brackets or operators, can trigger this error. -
what are some best practices to avoid this error in Bash?
Always initialize your variables, use appropriate operators, and ensure correct syntax in your conditional statements.
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedInRelated Article - Bash Operator
- How to Use Double and Single Pipes in Bash
- How to Use the Mod Operator in Bash
- Ternary Operator in Bash Script
- Logical OR Operator in Bash Script
- The -ne Operator in Bash