Logical OR Operator in Bash Script
-
Logical
OR
Operator (||
) in Bash -
Logical
OR
Operator (||
) With Non-Boolean Values -
Use Logical
OR
Operator (||
) in Bash Scripting
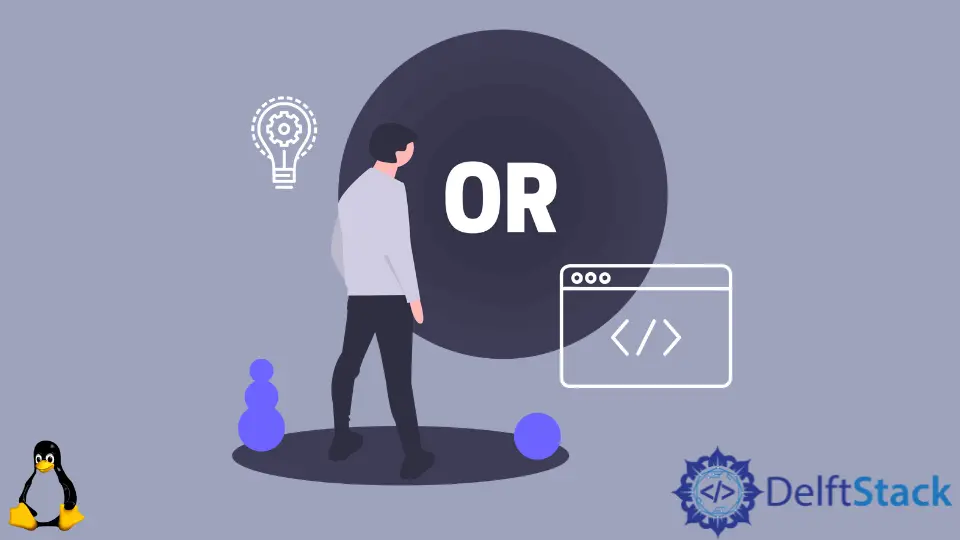
We will explain the logical OR
operator (||
) and how it works throughout this article. We will also give examples of how it can be used in Bash scripting.
Logical OR
Operator (||
) in Bash
The logical OR
operator ||
processes multiple values. It is usually used with boolean values and returns a boolean value. It returns true
if at least one of the operands is true. Returns false
if all values are false.
Let’s give a few examples.
True || False --> True
False || True --> True
True || True --> True
False || False --> False
Logical OR
Operator (||
) With Non-Boolean Values
The return value will not be a boolean if the logical OR
operator (||
) is used with non-boolean values like strings and numbers. Consider the example below.
param1 || param2 || param3
If the boolean value of param1
is true
, it returns the value of this operand, and the other operands are ignored. Otherwise, param2
is checked.
If its boolean value is true
, the result is the value of param2
, and the rest of the operands are ignored. This process continues until the last operand.
If the boolean value of the last operand is also not true
, the result is the last operand’s value.
The boolean value of the following expressions is false
. The rest is true
.
0
NaN
null
undefined
""
,''
(Empty string)
Let’s give examples for non-boolean values.
1 || 0 --> 1
"" || 1 --> 1
"" || 0 --> 0
1 || "x" --> 1
"x" || 1 --> "x"
Use Logical OR
Operator (||
) in Bash Scripting
The logical OR
operator (||
) is the same in Bash scripting. Now, we will examine the example with Bash scripting syntax.
if [ 1 -eq 2 ] || [ 1 -eq 1 ]; then
echo "The result of the operation is true"
else
echo "The result of the operation is false"
fi
In the above if
block, the operands are given to the logical OR
operator (||
). The result is false
in the first comparison because 1
and 2
are not equal.
Since the two values are the same in the second comparison, they are equal, resulting in true
. So, our process becomes false || true
. This operation returns true
, and the first echo
command runs as we explained above.
We can also use the -o
flag for this operation.
if [ 1 -eq 2 -o 3 -eq 4 ]; then
echo "The result of the operation is true"
else
echo "The result of the operation is false"
fi
The logic of this code is the same as the first one. But this time, the result of the operation will be false
as both comparisons are false. So, the echo
command inside the else
block runs.
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn