Regex Match in Bash
- Re-Match Operator in Bash
- Match Numbers in Bash
- Regex Match the Beginning of a String in Bash
- Regex Match the End of a String in Bash
- Regex Match Email in Bash
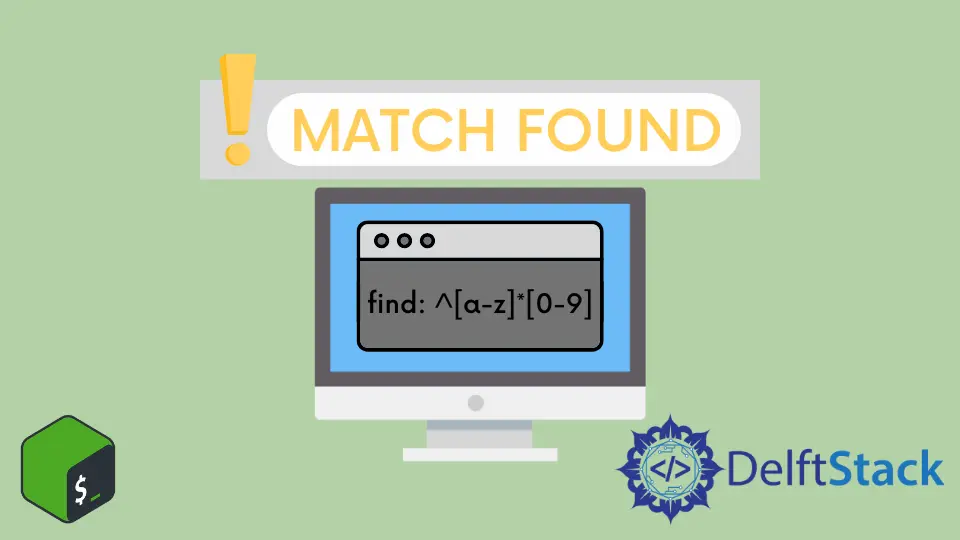
This tutorial demonstrates regular expression match using the re-match operator in bash.
Re-Match Operator in Bash
The =~
operator is known as the re-match operator. It uses the following syntax.
[[ string =~ regex ]]
The re-match operator performs regular expression matching of the string on its left to the right. If the left side matches the right side, the operator returns a 0
and a 1
otherwise.
Match Numbers in Bash
The script below checks if the variable $greet
has the characters mentioned on the right side, numbers in our case. If there is a complete match, the re-match operator returns a 0
, and the script prints out the correct output.
greet="Hello,World!"
if [[ $greet =~ [0-9] ]]; then
printf "$greet is a number\n"
else
printf "$greet is not a number\n"
fi
Since the value of the $greet
variable only has characters other than numbers, the re-match operator returns a 1
and prints the output below.
Hello,World! is not a number
Here we create a new variable in the script that has numbers only called num
. We then check if the num
variable has numbers only, and we print the respective output depending on the value returned by the re-match operator.
#!/bin/env bash
num="2021"
if [[ $num =~ [0-9] ]]; then
printf "$num is a number\n"
else
printf "$num is not a number\n"
fi
Since the variable num
has numbers only, the re-match operator returns a 0
, and the script prints the statement below.
2021 is a number
Regex Match the Beginning of a String in Bash
The script below checks if the value in the $greet
variable begins with numbers. The ^
is used to specify to match the characters at the beginning of a string. Depending on the value returned by the operator, we print the respective output.
#!/bin/env bash
greet="123Hello,World!"
if [[ $greet =~ ^[0-9] ]]; then
printf "$greet starts with a digit(s).\n"
else
printf "$greet does not start with a digit(s)\n"
fi
Since the value in the $greet
variable begins with a number, the operator returns a 0
, and prints the output below.
123Hello,World! starts with a digit(s).
Let us set $greet
to a new value as shown below and run the script.
#!/bin/env bash
greet="Hello,World!123"
if [[ $greet =~ ^[0-9] ]]; then
printf "$greet starts with a digit(s).\n"
else
printf "$greet does not start with a digit(s)\n"
fi
The bash operator will return 1
since the string in the variable, $greet
, does not begin with a number. The script will print the output shown below.
Hello,World!123 does not start with a digit(s)
Regex Match the End of a String in Bash
The script below checks if the value in the $greet
variable ends with numbers. The $
is used to specify to match the characters at the end of the string.
The output to be printed out will depend on the value returned by the re-match operator.
#!/bin/env bash
greet="Hello,World!123"
if [[ $greet =~ [0-9]$ ]]; then
printf "$greet ends with a digit(s).\n"
else
printf "$greet does not end with a digit(s)\n"
fi
The string value in the $greet
variable ends with numbers. The re-match operator returns a 0
, and the script prints the output below.
Hello,World!123 ends with a digit(s).
Let us change the value of the $greet
variable as shown in the script below.
#!/bin/env bash
greet="123Hello,World!"
if [[ $greet =~ [0-9]$ ]]; then
printf "$greet ends with a digit(s).\n"
else
printf "$greet does not end with a digit(s)\n"
fi
Since the string in the variable $greet
does not end with a number or numbers, the re-match operator returns 1
, and the script prints the output below.
123Hello,World! does not end with a digit(s)
Regex Match Email in Bash
To match emails, this ^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$
regex pattern is generally used.
#!/bin/env bash
email="example@delftstack.com"
if [[ $email =~ ^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$ ]]; then
printf "$email is a valid email.\n"
else
printf "$email is not a valid email.\n"
fi
The output below shows an email that was checked and has passed the check.
example@delftstack.com is a valid email.
Let us remove the .com
at the end of the email as shown below and run the script.
#!/bin/env bash
email="example@delftstack"
if [[ $email =~ ^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$ ]]; then
printf "$email is a valid email.\n"
else
printf "$email is not a valid email.\n"
fi
The email fails the test because it does not have the last part, the .com
.
example@delftstack is not a valid email.