How to Use Functions in Bash
- Define Functions in Bash
- Scope of Variables in Bash
- Return Values From Function in Bash
- Pass Arguments to a Bash Function
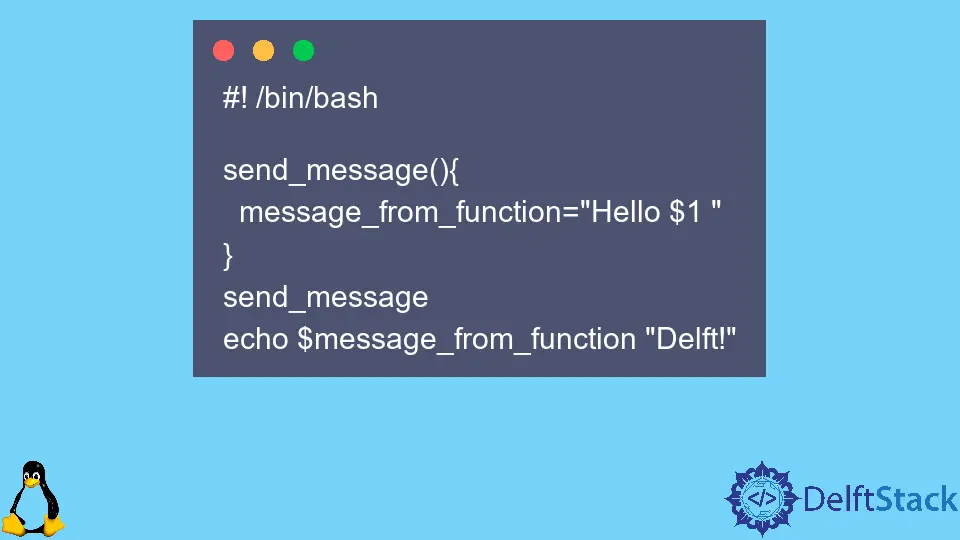
A function is one of the most important aspects of every programming language, and it makes our code reusable and readable. The same code used repeatedly is written inside a function. And when we need the code block, we simply call the defined function.
Define Functions in Bash
To define functions in Bash, we can use any one of the following two approaches:
In this approach, we write the name of the function followed by parentheses. Then we define the functions inside the curly braces. The functions using this format can be defined using any one of the formats:
function_name(){
statements
}
function_name() { statements }
In this approach, we specify the function
keyword before the function name.
function_name(){
statements
}
function_name() { statements }
The statements inside curly braces determine what the function does. The function’s name has nothing to do with what the function does, but we must make the function names descriptive. Defining the function won’t do anything. To execute the commands in the function definition, we must call the function.
To call the function in Bash
, we use the name of the function.
#! /bin/bash
greet(){
echo 'Hello, Folks. Welcome to DelftStack!'
}
greet
Output:
Hello, Folks. Welcome to DelftStack!
Here, we define the function at the beginning with the name greet
and then call the function. When we call the function, the statements inside a function definition are executed, and hence we see Hello, Folks. Welcome to DelftStack!
printed in the terminal.
We can also define the function using another approach as:
#! /bin/bash
function greet(){
echo 'Hello, Folks. Welcome to DelftStack!'
}
greet
Output:
Hello, Folks. Welcome to DelftStack!
We must make sure that we always define the function before calling it.
Scope of Variables in Bash
The scope of variables can be either global or local in Bash as of other programming languages. However, the default scope of variables is always global, even if it is declared inside the function scope. We must use the local
keyword to make a variable local.
#! /bin/bash
gvar1=1
gvar2=2
change_variables() {
local gvar1=10
gvar2=7
echo "Inside Function: gvar1: $gvar1, gvar2: $gvar2"
}
echo "Before executing function: gvar1: $gvar1, gvar2: $gvar2"
change_variables
echo "After executing function: gvar1: $gvar1, gvar2: $gvar2"
Output:
Before executing function: gvar1: 1, gvar2: 2
Inside Function: gvar1: 10, gvar2: 7
After executing function: gvar1: 1, gvar2: 7
Here, gvar1
and gvar2
declared at the top are global variables.
Inside the function, gvar1
is declared as a local variable since we use the local
keyword with gvar1
while gvar2
still refers to a global variable since it does not have a local
keyword. Hence, the value of gvar2
is changed globally by the function while the value of gvar1
is changed inside the function only.
Return Values From Function in Bash
In contrary to other programming languages, Bash doesn’t allow us to return values from the function. The value returned from the Bash function is the status of the last statement executed in the function. The number 0
represents the success, while the number from 1-255
represents the failure. We can specify the exit status of the function using the return
keyword. The return
statement also terminates the function.
#! /bin/bash
return_value(){
echo "This function returns 20!"
return 30
echo "After return statement"
}
return_value
echo $?
Output:
This function returns 20!
30
Here, we can see that the function return_value
returns the value 30
using the return
statement, and the value is assigned to $?
variable.
We can also note that the function is terminated after the return
statement is executed as commands below the return
statement are not executed.
If we want to return some value from a function, we can assign the value to a global variable and access the variable from outside the function to retrieve the value.
#! /bin/bash
send_message(){
message_from_function="Hello From the function!"
}
send_message
echo $message_from_function
Output:
Hello From the function!
In the program, we assign the value to be returned to the global variable message_from_function
and then access the variable’s returned value.
Pass Arguments to a Bash Function
To pass arguments to a Bash function, we simply put the arguments after the function. If we want to pass more than one argument to a function, we separate the arguments by a space. To avoid misparsing of arguments, we can wrap arguments in double-quotes.
The parameters are accessed with $1
$2
and so on based on their position. $0
represents the function name while $#
holds the number of positional arguments in the function.
#! /bin/bash
send_message(){
message_from_function="Hello $1 "
}
send_message
echo $message_from_function "Delft!"
Output:
Hello Delft!
Here, the argument Delft!
is passed into the function and accessed in the function by the parameter $1
, and we get Hello Delft!
as the final output from the function.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn