How to Perform Increment and Decrement Operation in Bash
-
Increment and Decrement With
+
and-
Operators -
Increment and Decrement With
+=
and-=
Operators -
Increment and Decrement With
++
and--
Operators
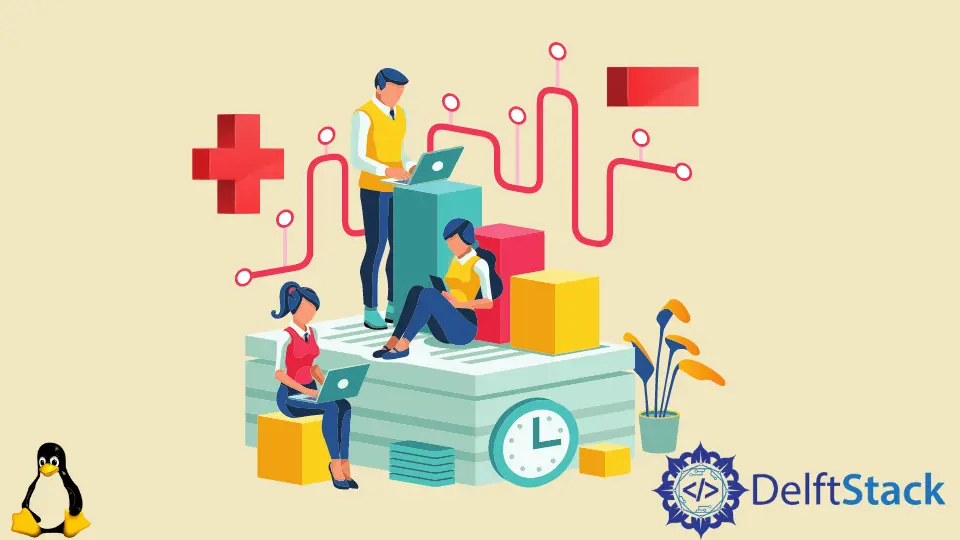
Increment and decrement operations are one of the most widely used operations in every programming language. They are mostly used as counters in loops. To perform variable increment and decrement in Bash, we can make use +
and -
operators or +=
and -=
operators. We can also perform increment and decrement in Bash using unary operators: ++
and --
.
Increment and Decrement With +
and -
Operators
It is the simplest way to perform increment and decrement operation. We carry out the operation by enclosing the expressions inside ((..))
or $((...))
or by using the built-in let
command.
x=3
y=3
z=3
x=$((x+1))
((y=y+1))
let "z=z+1"
echo x: $x
echo y: $y
echo z: $z
Output:
x: 4
y: 4
z: 4
We can see that we can perform the same increment operation using +
in three different ways.
We can also similarly perform the decrement operation.
x=3
y=3
z=3
x=$((x-1))
((y=y-1))
let "z=z-1"
echo x: $x
echo y: $y
echo z: $z
Output:
x: 2
y: 2
z: 2
It performs the decrement operation using the -
operator in 3 different ways.
Increment and Decrement With +=
and -=
Operators
The operators +=
and -=
increase or decrease the value at the operator’s left side by the value at the right side of the operator.
x+=y
is equivalent to x=x+y
and x-=y
is equivalent to x=x-y
.
x=3
y=3
((x+=1))
let "y-=1"
echo x: $x
echo y: $y
Output:
x: 4
y: 2
Here, in the beginning, the values of both x
and y
are 3
. After the increment operation on x
, its value becomes 4
. Similarly, after decrement operation on y
, the value of y
becomes 2
.
We can also implement increment or decrement in a loop.
x=1
while [ $x -le 5 ]
do
echo x: $x
let "x+=1"
done
Output:
x: 1
x: 2
x: 3
x: 4
x: 5
It increments the value of x as long as the value of x is less than or equal to 5.
Increment and Decrement With ++
and --
Operators
The operators ++
and --
are unary, increasing or decreasing the value operand by 1.
x++
is equivalent to x=x+1
and x--
is equivalent to x=x-1
.
x=3
y=3
((x++))
let "y--"
echo x: $x
echo y: $y
Output:
x: 4
y: 2
Here, in the beginning, the values of both x
and y
is 3
. After the increment operation on x
, it’s value becomes 4
. Similarly, after decrement operation on y
, the value of y
becomes 2
.
We can also implement ++
and --
operators inside a loop.
x=5
while [ $x -ge 1 ]
do
echo x: $x
let "x--"
done
Output:
x: 5
x: 4
x: 3
x: 2
x: 1
It decrements the value of x as long as the value of x is greater than or equal to 1.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn