How to Compare Strings in Bash
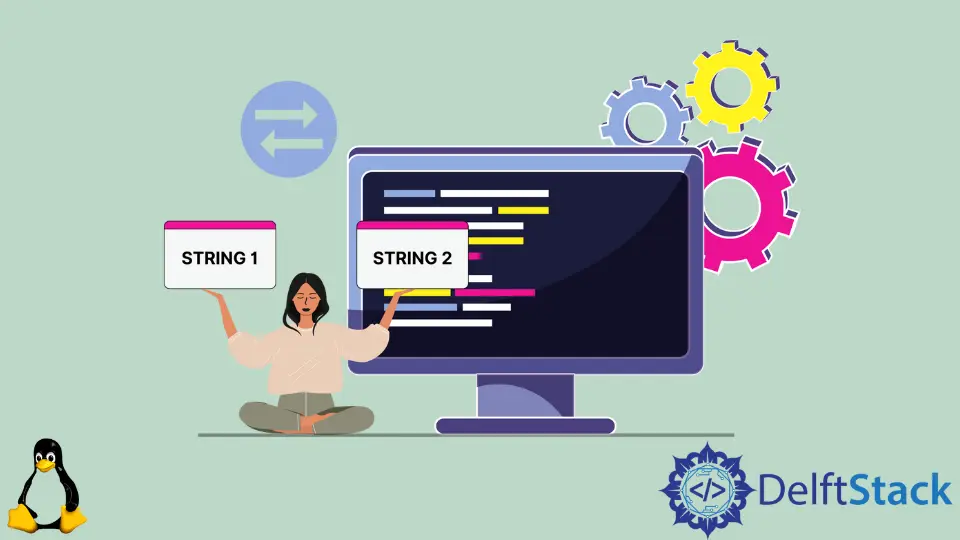
We can compare the strings using various comparison operators and check whether the string contains substring or not using the regular expressions.
String Comparison in Bash
String Comparison means to check whether the given strings are the same or not. Two or more strings are the same if they are of equal length and contain the same sequence of characters.
We use various string comparison operators which return true or false depending upon the condition. Some of the widely used string comparison operators could be listed as:
string1 = string2 |
Equality operator used with [ command and returns true if both operands are equal. |
string1 == string2 |
Equality operator used with [[ command and returns true if both operands are equal. |
string1 != string2 |
Inequality operator which returns true if two operands are not equal. |
string1 =~ regex |
Regex operator which returns true if the string1 matches the extended regex |
string1 > string2 |
Greater than operator which returns true if the string1 is greater than string2 based on lexicographical (alphabetical) order |
string1 < string2 |
Less than operator which returns true if the string1 is smaller than string2 based on lexicographical (alphabetical) order |
-z string |
Return true if the length of string is 0 . |
-n string |
Return true if the length of string is not 0 . |
String1="Hello World!!"
String2="Hello World!!"
String3="Delft Stack"
if [ "$String1" = "$String2" ]; then
echo "String1 and String2 are equal."
else
echo "String1 and String2 are not equal."
fi
if [[ "$String1" == "$String2" ]]; then
echo "String1 and String2 are equal."
else
echo "String1 and String2 are not equal."
fi
if [[ "$String1" != "$String3" ]]; then
echo "String1 and String3 are not equal."
else
echo "String1 and String3 are equal."
fi
Output:
String1 and String2 are equal.
String1 and String2 are equal.
String1 and String3 are not equal.
Here, if we compare String1
and String2
using the =
operator at first. As String1
and String2
both have the same length with the same sequence of characters, the comparison operator returns true
and hence we get String1 and String2 are equal.
as output from the first if-else
block of the program.
Similarly, in the second program, we compare String1
and String2
using the ==
operator. We need to use [[
for comparison in this case.
Finally, we compare String1
and String3
using the !=
operator.
Lexicographic Comparison in Bash
Lexicographic comparison means comparing strings based on alphabetical order. For lexicographic comparison, we use >
and <
operators.
name1="Kamal"
name2="Abinash"
if [[ "$name1" > "$name2" ]]; then
echo "${name1} is greater then ${name2}."
elif [[ "$name1" < "$name2" ]]; then
echo "${name2} is greater than ${name1}."
else
echo "Both the namees are equal"
fi
Output:
Kamal is greater then Abinash.
In this program, name1
and name2
are compared lexicographically. As K
comes after A
in the alphabetical order, K
has a higher value than A
and hence "$name1" > "$name2"
returns true
and we get Kamal is greater then Abinash.
as an output.
Check if a String Is Empty or Not
We use -n
and -z
operators to check if the string is empty or not.
String=""
if [[ -z $String ]]; then
echo "The variable String is an empty string."
fi
Output:
The variable String is an empty string.
In this program, String
is an empty variable. As -z
operator returns true
if the length of string
is 0
and hence we get The variable String is an empty string.
as an output from the given program.
String="Test"
if [[ -n $String ]]; then
echo "The variable String is not an empty string."
fi
Output:
The variable String is not an empty string.
In this program, String
is not an empty variable. As -n
operator returns true
if the length of string
is not 0
and hence we get The variable String is not an empty string.
as an output from the given program.
Check if a String Contains Substring
To check if a string contains a substring, we can use the =~
(Regex
) operator.
The regex
operator returns true
if the string
matches the extended regex
expression. We must make an appropriate regex
expression for comparison.
String='My name is Delft.'
if [[ $String =~ .*Delft.* ]]; then
echo "The given string has Delft on it."
fi
Output:
The given string has Delft on it.
Here, .*Delft.*
is the regex
expression to be matched, which says match any string, zero or more characters, before and after Delft.
. It checks if the string has substring Delft
in it or not.
As Delft
is present in the given string, the given condition is satisfied, and we get The given string has Delft on it.
as an output.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn