How to Append Text to a File Using Bash
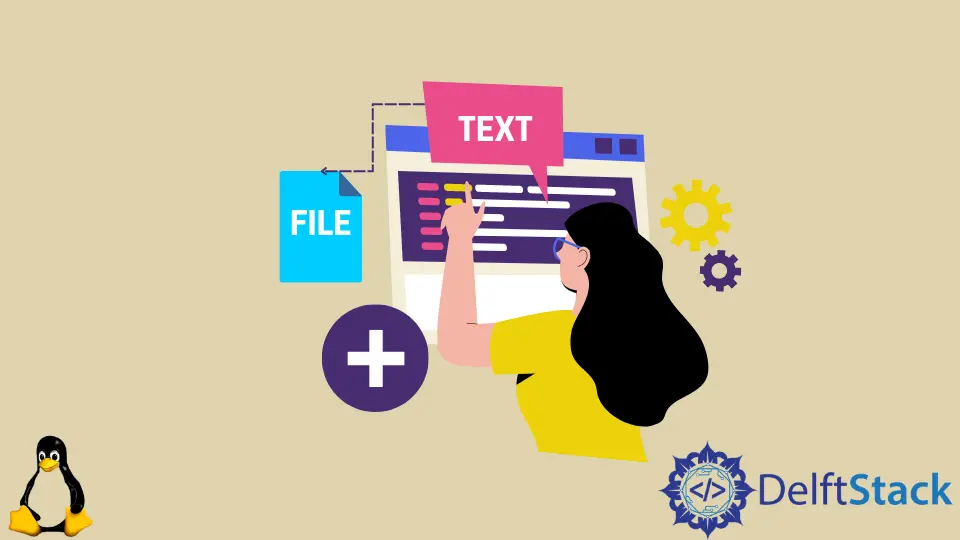
We can append text to a file using the Redirection (>>
) operator, and the tee
command.
We must make sure we have enough permissions to add text to the file. If we do not have enough permissions, we may get permission denied error.
Append Text to a File With the Redirection Operator >>
The redirection operator >>
fetches the output from the bash
commands and appends the output to another file. There are a lot of ways to print the text to the standard output, however echo
and printf
are the most popular commands.
To append the text to a file, we write a command to print the text using any of the output commands and then append the >>
operator to the command followed by name of the file where the text needs to be added.
echo "Hello World" >> abc.txt
This will add the text Hello World
at the end of the file abc.txt
.
If the file doesn’t exist in the current working directory, the command will create an empty file and write the text Hello World
in the file.
To interpret the escape sequence characters such as \n
we use the -e
option with the echo
command.
echo -e "Hello World! \nThis is DelftStack" >> abc.txt
This will append the text
Hello World!
This is DelftStack
to the file abc.txt
.
To verify whether the text has been appended or not, we can use the cat
command to view the contents of the file.
cat abc.txt
Output:
Hello World!
This is DelftStack
If the text has been appended, we can see the text at the end of the file.
If we want more formatted text, we can use the printf
command to produce more formatted output.
printf "The path of shell interpreter is %s\n" $SHELL >> abc.txt
It adds the text The path of shell interpreter is /bin/bash
to the file abc.txt
.
Append Text to a File With the tee
Command
tee
is a command-line utility that takes input from standard input and writes it to one or more files and standard output simultaneously.
By default, the tee
command overwrites the content of the files. To just append the text at the end of the file, we use the -a
or --append
option with the command.
echo "Hello World!" | tee -a abc.txt
Output:
Hello World!
It appends Hello World!
to the file abc.txt
and also writes the text to the standard output in the terminal.
If we get permission denied error while appending text to a file, we can add the sudo
keyword before the tee
command.
echo "Hello World!" | sudo tee -a abc.txt
If we don’t want to see the standard output, we can redirect the output to /dev/null
echo "Hello World!" | tee -a abc.txt >/dev/null
One advantage of using the tee
command is that we can write the text to multiple files simultaneously using tee
command. To write the text to multiple files, we can just list all the files separated by a space.
echo "Hello World!" | sudo tee -a abc.txt backup.txt
It appends the text to both files abc.txt
and backup.txt
simultaneously.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn