Fork in Bash
- What is Fork in Bash?
- Basic Forking in Bash
- Advanced Forking Techniques
- Managing Process IDs
- Conclusion
- FAQ
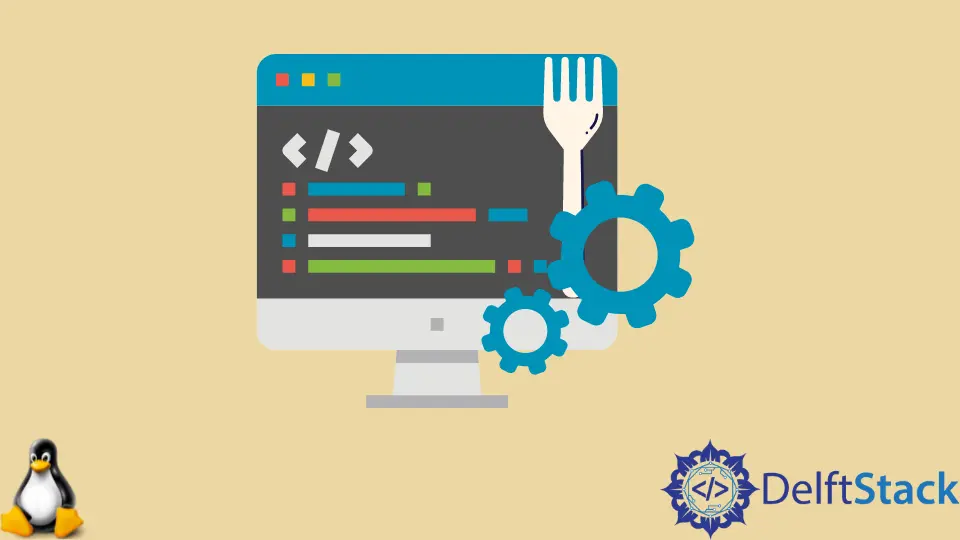
When it comes to scripting in Bash, understanding how to manage processes is crucial. One of the fundamental concepts in this area is the “fork” system call. Forking allows you to create a new process by duplicating an existing one, which can be particularly useful for executing multiple tasks simultaneously.
In this article, we will explore how to effectively use the fork call in Bash, including its implications and practical applications. If you’re looking to enhance your Bash scripting skills and understand how to handle concurrent processes, this guide is for you. Let’s dive into the details of forking in Bash and its relevance in the world of scripting.
What is Fork in Bash?
Forking in Bash is a method that enables the creation of a new process. When a script calls the fork function, it creates a child process that runs concurrently with the parent process. This is particularly useful when you want to perform tasks in parallel without blocking the main execution flow. The forked process inherits the memory and environment of the parent process, but they can execute different tasks independently.
In Bash, forking is typically done by executing a command in the background using the ampersand (&
) symbol. This allows the parent process to continue executing while the child process runs in the background. Understanding how to manage these processes can significantly enhance the efficiency of your scripts.
Basic Forking in Bash
To get started with forking in Bash, you can use a simple script that demonstrates how to create a child process. Here’s a basic example:
#!/bin/bash
echo "Starting parent process..."
# Forking a child process
(
echo "This is the child process."
sleep 2
echo "Child process is done."
) &
# Parent process continues
echo "Parent process continues to run."
wait
echo "Parent process is done."
Output:
Starting parent process...
Parent process continues to run.
This is the child process.
Child process is done.
Parent process is done.
In this script, we start by echoing that the parent process is beginning. We then create a child process using parentheses, which allows us to execute a block of commands in the background. The child process sleeps for two seconds before announcing its completion. Meanwhile, the parent process continues to run and outputs its own message. Finally, we use the wait
command to ensure that the parent process waits for the child to finish before completing its execution.
This simple example illustrates how forking can be used to run tasks concurrently, allowing for more efficient execution of scripts.
Advanced Forking Techniques
While basic forking is useful, there are more advanced techniques you can employ to manage multiple processes effectively. One common method is to use a loop to fork multiple child processes. Here’s an example:
#!/bin/bash
echo "Starting parent process..."
for i in {1..5}; do
(
echo "Child process $i is starting."
sleep $((RANDOM % 5 + 1))
echo "Child process $i is done."
) &
done
wait
echo "All child processes are done."
Output:
Starting parent process...
Child process 1 is starting.
Child process 2 is starting.
Child process 3 is starting.
Child process 4 is starting.
Child process 5 is starting.
Child process 3 is done.
Child process 1 is done.
Child process 4 is done.
Child process 2 is done.
Child process 5 is done.
All child processes are done.
In this script, we use a loop to create five child processes. Each child process announces its start and sleeps for a random duration before declaring its completion. The parent process waits for all child processes to finish before outputting a final message. This technique is particularly useful for tasks that can be executed in parallel, such as data processing or system monitoring.
Managing Process IDs
When working with multiple processes, it’s often important to manage their process IDs (PIDs). This allows you to track and control the execution of each process. Here’s how you can modify the previous example to capture and display the PIDs of the child processes:
#!/bin/bash
echo "Starting parent process..."
for i in {1..5}; do
(
echo "Child process $i (PID $$) is starting."
sleep $((RANDOM % 5 + 1))
echo "Child process $i is done."
) &
done
wait
echo "All child processes are done."
Output:
Starting parent process...
Child process 1 (PID 1234) is starting.
Child process 2 (PID 1235) is starting.
Child process 3 (PID 1236) is starting.
Child process 4 (PID 1237) is starting.
Child process 5 (PID 1238) is starting.
Child process 3 is done.
Child process 1 is done.
Child process 4 is done.
Child process 2 is done.
Child process 5 is done.
All child processes are done.
In this version, each child process outputs its own PID, which is represented by $$
. This can be particularly useful for debugging or logging purposes, as it allows you to identify which process is performing which task.
Conclusion
Understanding the fork call in Bash is essential for anyone looking to enhance their scripting skills. By mastering the art of forking, you can create efficient, concurrent processes that allow your scripts to run faster and more effectively. Whether you’re managing simple tasks or complex operations, forking provides the flexibility needed to handle multiple processes seamlessly. As you continue to explore Bash scripting, keep these techniques in mind to improve your workflow and productivity.
FAQ
-
What does forking mean in Bash?
Forking in Bash refers to the creation of a new process by duplicating an existing one, allowing concurrent execution of tasks. -
How do I create a child process in Bash?
You can create a child process by using parentheses and placing the commands you want to run inside them followed by an ampersand (&) to run it in the background. -
What is the purpose of the wait command in Bash?
The wait command is used to pause the execution of the parent process until all child processes have completed. -
Can I manage process IDs in Bash?
Yes, you can manage process IDs in Bash by using the special variable $$, which represents the PID of the current process. -
How can I run multiple processes simultaneously in Bash?
You can run multiple processes simultaneously by using a loop to fork several child processes, each executing its own set of commands in the background.