How to Emulate Do-While Loop in Bash
- Understanding the Do-While Loop Concept
-
Method 1: Using a
while
Loop with a Conditional Check - Method 2: Using a Function to Encapsulate Logic
- Method 3: Using a Until Loop
- Conclusion
- FAQ
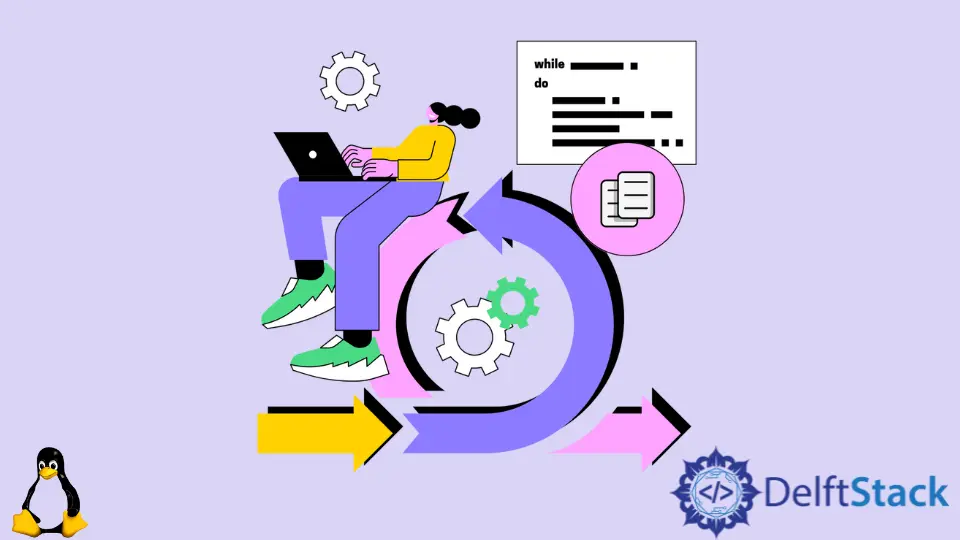
In the world of programming, loops are essential for executing repetitive tasks efficiently. While many programming languages, including C and Java, provide a built-in do-while loop, Bash does not offer this construct directly. However, you can still emulate a do-while loop in Bash with a few clever techniques.
This tutorial will guide you through various methods to achieve this functionality, ensuring you can control the flow of your Bash scripts effectively. Whether you’re a seasoned developer or a novice scripter, understanding how to implement a do-while loop in Bash can enhance your scripting capabilities. Let’s dive in!
Understanding the Do-While Loop Concept
Before we jump into the practical implementations, it’s essential to understand the concept of a do-while loop. In languages that support it, a do-while loop executes a block of code at least once before checking a condition. This means that the loop’s body runs first, and then the condition is evaluated. If the condition is true, the loop will continue; if false, it will terminate. This behavior is particularly useful when you need to ensure that a block of code runs at least once, regardless of the condition.
Method 1: Using a while
Loop with a Conditional Check
One of the simplest ways to emulate a do-while loop in Bash is by using a while
loop with a conditional check. This method involves running the loop body first and then using a conditional statement to determine if the loop should continue. Here’s how you can do it:
#!/bin/bash
count=1
while true; do
echo "Count is $count"
((count++))
if [ $count -gt 5 ]; then
break
fi
done
Output:
Count is 1
Count is 2
Count is 3
Count is 4
Count is 5
In this example, we initialize a variable count
to 1. The loop starts with while true
, meaning it will run indefinitely until we explicitly break out of it. Inside the loop, we print the current value of count
and increment it by 1. The condition to exit the loop checks if count
is greater than 5. Once this condition is met, the break
statement terminates the loop.
This approach effectively simulates the do-while behavior by ensuring that the loop body executes at least once before evaluating the exit condition.
Method 2: Using a Function to Encapsulate Logic
Another method to emulate a do-while loop in Bash is by encapsulating the logic within a function. This not only organizes your code better but also allows for reusability. Here’s how you can implement this:
#!/bin/bash
do_while_function() {
local count=1
while true; do
echo "Count is $count"
((count++))
if [ $count -gt 5 ]; then
break
fi
done
}
do_while_function
Output:
Count is 1
Count is 2
Count is 3
Count is 4
Count is 5
In this implementation, we define a function named do_while_function
. Inside this function, the same logic from the previous example is used to execute the loop. The advantage here is that you can call do_while_function
from anywhere in your script, making your code modular and easier to maintain. The while
loop again runs indefinitely until the condition to break is met. This approach is particularly beneficial for larger scripts where you want to reuse the loop logic.
Method 3: Using a Until Loop
While the until loop is not exactly the same as a do-while loop, it can be used creatively to achieve similar results. The until loop continues until a specified condition is true. Here’s how to implement it:
#!/bin/bash
count=1
until [ $count -gt 5 ]; do
echo "Count is $count"
((count++))
done
Output:
Count is 1
Count is 2
Count is 3
Count is 4
Count is 5
In this example, we initialize count
to 1 and use an until loop to execute the block of code until count
exceeds 5. The loop prints the current value of count
and increments it. Although the until loop checks the condition at the end, it effectively behaves similarly to a do-while loop in this context. This method can be particularly useful when you want to ensure the loop runs until a certain condition is met.
Conclusion
Emulating a do-while loop in Bash may not be as straightforward as in some other programming languages, but with the methods outlined above, you can achieve similar functionality effectively. Whether you choose to use a while
loop with a conditional check, encapsulate your logic in a function, or utilize an until loop, each method has its advantages. Understanding these techniques will enhance your scripting skills and enable you to write more efficient Bash scripts. Happy scripting!
FAQ
-
Can Bash natively support a do-while loop?
Bash does not have a built-in do-while loop, but you can emulate it using other loop constructs. -
What is the main difference between a
while
loop and a do-while loop?
Awhile
loop checks the condition before executing the loop body, while a do-while loop executes the body first and checks the condition afterward. -
Are there performance differences between the methods discussed?
Generally, the performance differences are negligible for small scripts. Choose the method that best fits your coding style and requirements. -
Can I use these methods in scripts that interact with Git?
Yes, these loop emulation techniques can be used in any Bash script, including those that involve Git commands. -
Is it better to use functions for loop logic in larger scripts?
Yes, encapsulating loop logic in functions can improve code organization and reusability, especially in larger scripts.