How to Pretty Print JSON in a Shell Script
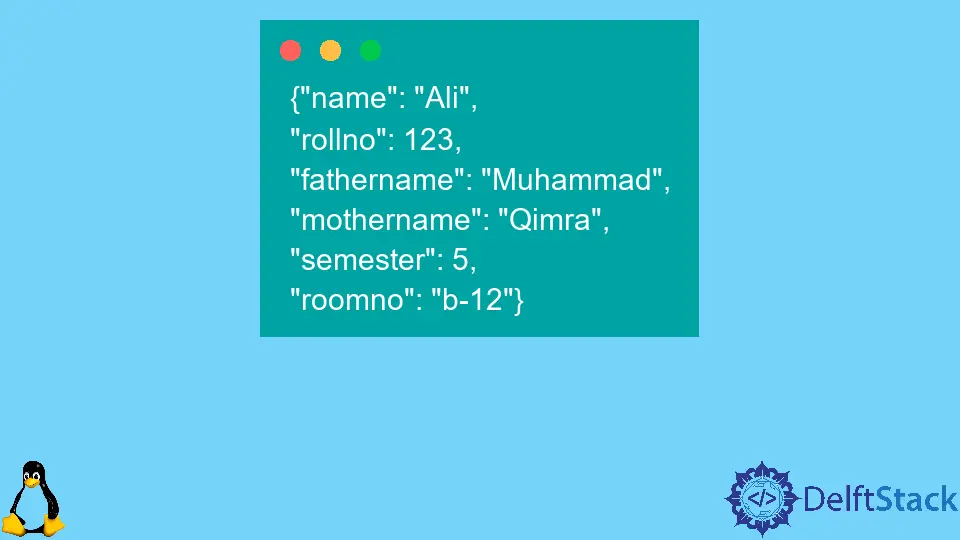
JSON is a textual approach to representing JavaScript object literals and arrays alongside the scalar data. It is comparatively easier to read and write and more manageable for software to parse and spawn.
JSON is often used for serializing structured data and exchanging it over a network, typically between a server and web applications.
Pretty Print JSON in a Shell Script
While working with JSON, one could want its output in a more human-understandable format. Consider the JSON object below:
{"name": "Ali", "rollno": 123, "fathername": "Muhammad", "mothername": "Qimra", "semester": 5, "roomno": "b-12", }
Understanding the attributes of the above JSON object is already hard enough with not even a dozen attributes, so imagine what sort of a nightmare it would be with hundreds of attributes.
Hence, it is important to format it into a more human-friendly format like the JSON attribute below:
{"name": "Ali",
"rollno": 123,
"fathername": "Muhammad",
"mothername": "Qimra",
"semester": 5,
"roomno": "b-12"}
Notice how much cleaner it looks now. We will now go through a multitude of ways to accomplish the above.
With python 2.6+, you can use:
echo '{"key1": "val1", "keyn": "valn"}' | python -m json.tool
# or use
echo '{"key1": "val1", "keyn": "valn"}' | python2 -m json.tool
# or use
echo '{"key1": "val1", "keyn": "valn"}' | python3 -m json.tool
The output will be as follows:
{
"key1": "val1",
"keyn": "valn"
}
However, if you haven’t installed Python, Python2, or Python3, then you will get one of the following errors:
Command 'python' not found
Command 'python2' not found
Command 'python3' not found
To avoid this error, use the below command:
sudo apt install python python2 python3
Another way to do it, if you have the JSON object stored in a JSON file, would be to run the below command:
python -m json.tool <path to json file>.json
If, just in case, the JSON is from the internet source like an API, then use:
curl <url> | python -m json.tool
We will now see the other ways to accomplish the above.
Assume we have a file my_json.json
with the structure:
{ "key1": "val1", " keyn": "valn" }
Do notice we use (.
) to specify the current directory, as the file resides there:
sudo jq . my_json.json | less –R
jq '.key1' <<< '{ "key1": "val1", " keyn": "valn" }'
cat my_json.json| jq .
echo '{ "key1": "val1", " keyn": "valn" }' | npx json
To use jq
, you have to install it using the below command:
sudo apt install jq
The output of the first, third (that uses a pipe to transfer data from file my_json.json
to process jq
), and fourth methods is as follows:
{
"key1": "val1",
"keyn": "valn"
}
The fourth method needs a dependency of npx
, but don’t worry when you run that command. It will automatically install npx
if you don’t have it already.
The second method defines a JSON object and prints the key key1
value. Below is its output:
"val1"
We can also combine Python’s json.tool
with pigmentize
to pretty print the JSON object. Consider the command below:
echo '{ "key1": "val1", " keyn": "valn" }' | python -m json.tool | pygmentize –g
The output of the command above is as follows:
{
"key1": "val1",
"keyn": "valn"
}
However, to use the command above, you must install pigmentize
. To install pigmentize
, use the command below:
sudo apt install python3-pygments