How to Pass All Arguments in Bash
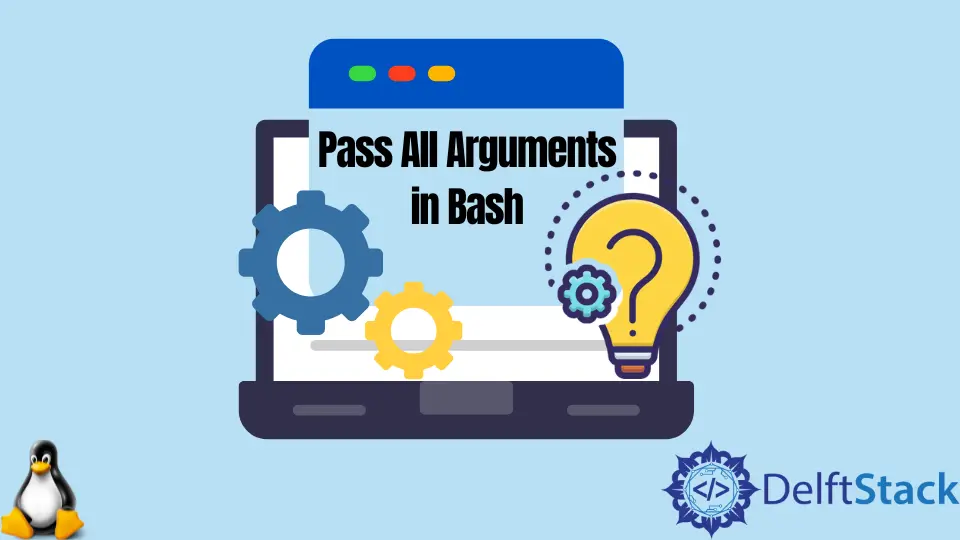
In Bash scripting, we mainly use the syntax $1 $2 $3 ... $N
for passing variables in the function. Here N
is the non-negative integer like 1, 2, 3, ...
.
But there is a shortcut way to do the same task of passing all the arguments. This article will discuss how we can pass all the arguments in a function.
Also, we will see necessary examples with explanations to make the topic easier to understand.
Pass All Arguments in Bash
For this purpose, we will use $@
to pass all the arguments. Let’s see the below example code.
My_Friends () {
echo "Your friend's names are: "$@""
}
My_Friends Alen Walker John
In the example above, we passed three parameters in a function named My_Friends
. We received all the functions in the function using $@
.
Now when you run the above example, you will get an output like the below.
Your friend's names are: Alen Walker John
Now the traditional way to perform the same task is like the below.
My_Friends () {
echo "Your friend's names are: $1 $2 $3"
}
My_Friends Alen Walker John
In the above code, we included all the parameters manually. This will also provide the same output as our previous example provided.
Please note that if you have to work with all the arguments separately, there is no way to include them manually. For example, if you are going to perform an add operation with all the passed arguments like our below example, then you cannot follow this shortcut method.
Let’s check our below example.
Add () {
echo The sum is: $(( $1+$2+$3 ))
}
Add 1 2 3
As we discussed, you must mention all the parameters separately in the recently shared example. This output of the above program is something like the below.
The sum is: 6
All the codes used in this article are written in Bash. It will only work in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn