How to Loop Through Directories Recursively in Bash
- Loop Through Directories Recursively in Bash
- Loop Through the Directory Recursively to Get the Files with a Particular Extension
- Loop Through the Directory Recursively to Move the File to Another Directory
- Loop Through the Directory Recursively to Delete the Files
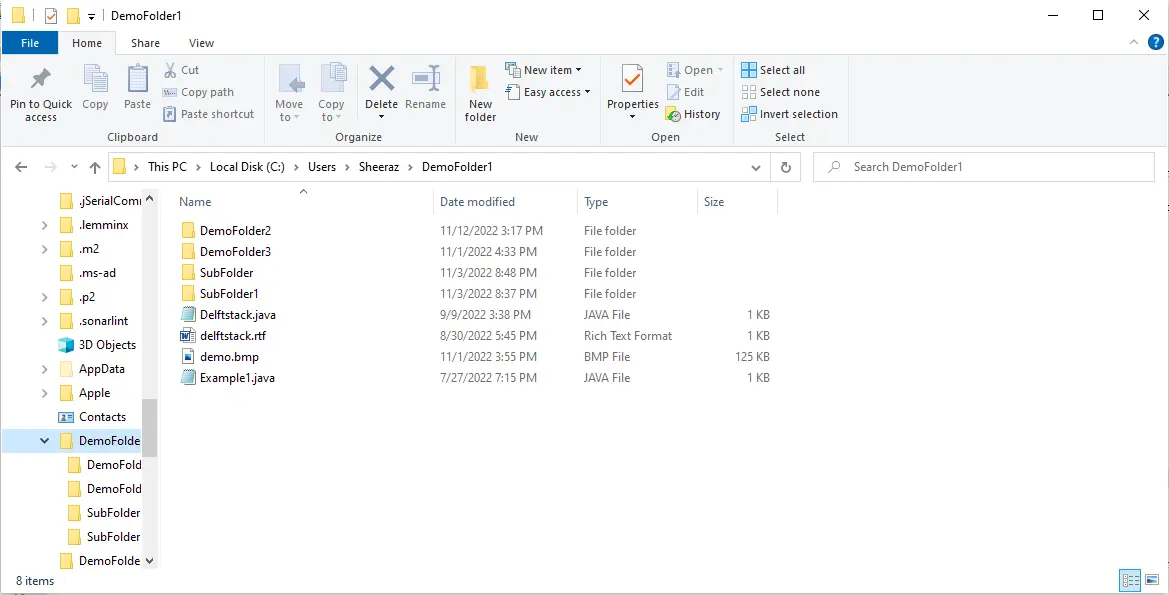
This tutorial demonstrates how to loop through directories recursively in Bash.
Loop Through Directories Recursively in Bash
Looping through directories is often required while working with different directories. We can use similar commands in all Linux terminals, including Bash, to loop through directories recursively.
First, we use the find
command to see the structure of the current directory. The find
command will show all the files and folders in the current or given directory.
To see all the files:
find . -type f -print0
To see the files of a particular directory:
find /mnt/c/Users/Sheeraz/DemoFolder1 -type f -print0
The /mnt/c/Users/Sheeraz/DemoFolder1
is the directory path we want to check. The structure of this directory is as follows:
The -type f
option is used to get only the files of that directory but not the folders. The output for this command is below.
/mnt/c/Users/Sheeraz/DemoFolder1/Delftstack.java/mnt/c/Users/Sheeraz/DemoFolder1/delftstack.rtf/mnt/c/Users/Sheeraz/DemoFolder1/demo.bmp/mnt/c/Users/Sheeraz/DemoFolder1/DemoFolder2/Delftstack.java/mnt/c/Users/Sheeraz/DemoFolder1/DemoFolder2/delftstack.rtf/mnt/c/Users/Sheeraz/DemoFolder1/DemoFolder2/demo.bmp/mnt/c/Users/Sheeraz/DemoFolder1/DemoFolder2/Example1.java/mnt/c/Users/Sheeraz/DemoFolder1/Example1.java/mnt/c/Users/Sheeraz/DemoFolder1/SubFolder/Delftstack.java/mnt/c/Users/Sheeraz/DemoFolder1/SubFolder/delftstack.rtf/mnt/c/Users/Sheeraz/DemoFolder1/SubFolder/demo.bmp/mnt/c/Users/Sheeraz/D
If you also want to get the folders of the directory, delete the -type f
from the above commands.
find /mnt/c/Users/Sheeraz/DemoFolder1 -print0
Once we know the file names structure then, we use the following code to loop through a directory recursively:
for x in $(find /mnt/c/Users/Sheeraz/DemoFolder1 -type f -print0)
do
echo $x;
done;
The code above loops through the directory /mnt/c/Users/Sheeraz/DemoFolder1
recursively and echo
the name of each file. See the output for this command:
/mnt/c/Users/Sheeraz/DemoFolder1/Delftstack.java/mnt/c/Users/Sheeraz/DemoFolder1/delftstack.rtf/mnt/c/Users/Sheeraz/DemoFolder1/demo.bmp/mnt/c/Users/Sheeraz/DemoFolder1/DemoFolder2/Delftstack.java/mnt/c/Users/Sheeraz/DemoFolder1/DemoFolder2/delftstack.rtf/mnt/c/Users/Sheeraz/DemoFolder1/DemoFolder2/demo.bmp/mnt/c/Users/Sheeraz/DemoFolder1/DemoFolder2/Example1.java/mnt/c/Users/Sheeraz/DemoFolder1/Example1.java/mnt/c/Users/Sheeraz/DemoFolder1/SubFolder/Delftstack.java/mnt/c/Users/Sheeraz/DemoFolder1/SubFolder/delftstack.rtf/mnt/c/Users/Sheeraz/DemoFolder1/SubFolder/demo.bmp/mnt/c/Users/Sheeraz/DemoFolder1/SubFolder/Example1.java
Loop Through the Directory Recursively to Get the Files with a Particular Extension
As we can see, we don’t need to show or work with all the files. We can also use this command with the -name
option to get the files with a particular extension.
See the commands:
for x in $(find /mnt/c/Users/Sheeraz/DemoFolder1 -type f -name '*.java');
do
echo $x;
done;
The above code will only print the files in /mnt/c/Users/Sheeraz/DemoFolder1
with the .java
extension. See the output:
/mnt/c/Users/Sheeraz/DemoFolder1/Delftstack.java
/mnt/c/Users/Sheeraz/DemoFolder1/DemoFolder2/Delftstack.java
/mnt/c/Users/Sheeraz/DemoFolder1/DemoFolder2/Example1.java
/mnt/c/Users/Sheeraz/DemoFolder1/Example1.java
/mnt/c/Users/Sheeraz/DemoFolder1/SubFolder/Delftstack.java
/mnt/c/Users/Sheeraz/DemoFolder1/SubFolder/Example1.java
Loop Through the Directory Recursively to Move the File to Another Directory
We can also move the files by recursively looping a directory using the Bash mv
command; the syntax for this command is below.
mv -v $filename $destination directory
We can use this command in a recursive loop to move the files to a destination directory. Let’s try an example.
for x in $(find /mnt/c/Users/Sheeraz/DemoFolder1 -type f -name '*.java');
do
mv -v $x /mnt/c/Users/Sheeraz/DemoFolder2
done;
The DemoFolder2
is an empty directory.
Output:
renamed '/mnt/c/Users/Sheeraz/DemoFolder1/Delftstack.java' -> '/mnt/c/Users/Sheeraz/DemoFolder2/Delftstack.java'
renamed '/mnt/c/Users/Sheeraz/DemoFolder1/DemoFolder2/Delftstack.java' -> '/mnt/c/Users/Sheeraz/DemoFolder2/Delftstack.java'
renamed '/mnt/c/Users/Sheeraz/DemoFolder1/DemoFolder2/Example1.java' -> '/mnt/c/Users/Sheeraz/DemoFolder2/Example1.java'
renamed '/mnt/c/Users/Sheeraz/DemoFolder1/Example1.java' -> '/mnt/c/Users/Sheeraz/DemoFolder2/Example1.java'
renamed '/mnt/c/Users/Sheeraz/DemoFolder1/SubFolder/Delftstack.java' -> '/mnt/c/Users/Sheeraz/DemoFolder2/Delftstack.java'
renamed '/mnt/c/Users/Sheeraz/DemoFolder1/SubFolder/Example1.java' -> '/mnt/c/Users/Sheeraz/DemoFolder2/Example1.java'
As we can see, the command looped recursively through the directory and moved the files with the extension java
to the destination directory.
Loop Through the Directory Recursively to Delete the Files
We can use the rm -rf
command to remove the files from the given directory. The syntax for this command is below.
rm -rf $filename
Let’s put this command in a recursive loop to recursively remove all the files from the directory.
for x in $(find /mnt/c/Users/Sheeraz/DemoFolder1 -type f);
do
rm -rf $x
done;
The above script will delete all the files from the main directory and subdirectories; it will not delete the folder because only the files are selected in the command. See the output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook